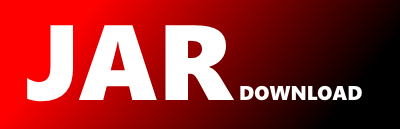
org.jsonddl.impl.Protected Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2011 Robert W. Vawter III
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.jsonddl.impl;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.jsonddl.JsonDdlObject;
public class Protected {
private static final Class> unmodifiableListClass;
private static final Class> unmodifiableMapClass;
static {
unmodifiableListClass = Collections.unmodifiableList(Arrays.asList(1, 2, 3)).getClass();
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy