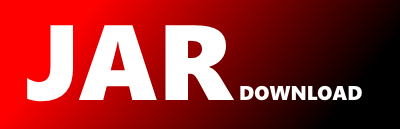
org.jspringbot.keyword.expression.ELUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2012. JSpringBot. All Rights Reserved.
*
* See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The JSpringBot licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jspringbot.keyword.expression;
import au.com.bytecode.opencsv.CSVReader;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang.StringUtils;
import org.jspringbot.MainContextHolder;
import org.jspringbot.PythonUtils;
import org.jspringbot.keyword.expression.plugin.VariableProviderManager;
import org.jspringbot.spring.ApplicationContextHolder;
import org.jspringbot.syntax.HighlightRobotLogger;
import org.python.util.PythonInterpreter;
import org.springframework.core.io.Resource;
import org.springframework.core.io.ResourceEditor;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.StringReader;
import java.math.BigInteger;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.text.DecimalFormat;
import java.text.ParseException;
import java.util.*;
import java.util.concurrent.Callable;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class ELUtils {
public static final HighlightRobotLogger LOG = HighlightRobotLogger.getLogger(ExpressionHelper.class);
public static final Pattern PATTERN = Pattern.compile("\\$\\{([^\\}]+)\\}", Pattern.CASE_INSENSITIVE);
public static final String EXCLUDE_INDICES = "excludeIndices";
private static final Map inCache = new HashMap();
public static final String IN_FILE_DEFAULT_LOCATION = "classpath:/expression/in.properties";
public static String resource(String resourceAsText) throws Exception {
ResourceEditor editor = new ResourceEditor();
editor.setAsText(resourceAsText);
Resource resource = (Resource) editor.getValue();
String resourceString = IOUtils.toString(resource.getInputStream());
return replaceVars(resourceString);
}
public static String md5(String str) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("MD5");
byte[] data = str.getBytes();
digest.update(data,0,data.length);
BigInteger i = new BigInteger(1,digest.digest());
String output = i.toString(16);
while (output.length() < 32){
output = "0"+output;
}
return output;
}
private static ExpressionHelper getHelper() {
return ApplicationContextHolder.get().getBean(ExpressionHelper.class);
}
private static VariableProviderManager getVariables() {
return new VariableProviderManager(ApplicationContextHolder.get());
}
@SuppressWarnings("unchecked")
public static List getExcludeIndices() {
Map variables = getVariables().getVariables();
if(variables.containsKey(EXCLUDE_INDICES)) {
return (List) variables.get(EXCLUDE_INDICES);
}
return Collections.emptyList();
}
public static Long getMillis(String str) {
return System.currentTimeMillis();
}
public static String concatMillis(String name) {
return name + System.currentTimeMillis();
}
public static int randomBetweenInt(int min, int max) {
return min + (int)(Math.random() * ((max - min) + 1));
}
public static String replaceVars(String string) throws Exception {
StringBuilder buf = new StringBuilder(string);
Matcher matcher = PATTERN.matcher(buf);
int startIndex = 0;
while(startIndex < buf.length() && matcher.find(startIndex)) {
String name = matcher.group(1);
Object value = getVariables().getVariables().get(name);
LOG.keywordAppender().appendProperty("Replacement EL Value ['" + name + "']", value);
if(value == null) {
value = robotVar(name);
LOG.keywordAppender().appendProperty("Replacement Robot Value ['" + name + "']", value);
}
if(value == null) {
value = System.getProperty(name);
LOG.keywordAppender().appendProperty("Replacement System Property Value ['" + name + "']", value);
}
if(value == null) {
value = System.getenv(name);
LOG.keywordAppender().appendProperty("Replacement System Environment Value ['" + name + "']", value);
}
String strValue = String.valueOf(value);
buf.replace(matcher.start(), matcher.end(), strValue);
startIndex = matcher.start() + strValue.length();
}
if(!StringUtils.equals(string, buf.toString())) {
LOG.keywordAppender().appendProperty(String.format("Replacement ['%s']", string), buf.toString());
}
return buf.toString();
}
public static Object eval(final String expression, Object... args) throws Exception {
LOG.keywordAppender().appendProperty("(eval) Expression", expression);
if(args != null && args.length > 0) {
LOG.keywordAppender().appendProperty("(eval) Arguments", args);
}
if(args == null || args.length == 0) {
return getHelper().evaluate(expression);
}
return getHelper().variableScope(Arrays.asList(args), new Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy