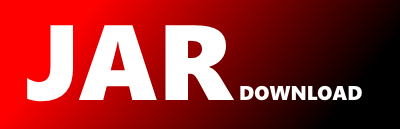
org.jspringbot.PythonUtils Maven / Gradle / Ivy
package org.jspringbot;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import org.python.core.*;
import org.springframework.aop.framework.ProxyFactory;
import java.util.*;
public class PythonUtils {
@SuppressWarnings("unchecked")
public static Object toJava(Object arg) {
if(List.class.isInstance(arg)) {
return proxy(List.class, (List) arg);
} else if(Set.class.isInstance(arg)) {
return proxy(Set.class, (Set) arg);
} else if(Map.class.isInstance(arg)) {
return proxy(Map.class, (Map) arg);
} else if(PyObject.class.isInstance(arg)) {
return ((PyObject) arg).__tojava__(Object.class);
} else if(PyNone.class.isInstance(arg)) {
return null;
} else {
return arg;
}
}
public static Object proxy(Class clazz, T object) {
return new PyProxyFactory(clazz, object).getProxy();
}
private static class PyProxyFactory implements MethodInterceptor {
private T delegate;
private Class clazz;
private PyProxyFactory(Class clazz, T delegate) {
this.clazz = clazz;
this.delegate = delegate;
}
public T getProxy() {
return ProxyFactory.getProxy(clazz, this);
}
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
Object returnedValue = invocation.getMethod().invoke(delegate, invocation.getArguments());
return toJava(returnedValue);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy