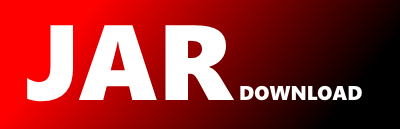
def.dom.Document Maven / Gradle / Ivy
The newest version!
package def.dom;
@jsweet.lang.Extends({GlobalEventHandlers.class,NodeSelector.class,DocumentEvent.class})
public class Document extends Node {
/**
* Sets or gets the URL for the current document.
*/
public java.lang.String URL;
/**
* Gets the URL for the document, stripped of any character encoding.
*/
public java.lang.String URLUnencoded;
/**
* Gets the object that has the focus when the parent document has focus.
*/
public Element activeElement;
/**
* Sets or gets the color of all active links in the document.
*/
public java.lang.String alinkColor;
/**
* Returns a reference to the collection of elements contained by the object.
*/
public HTMLCollection all;
/**
* Retrieves a collection of all a objects that have a name and/or id property. Objects in this collection are in HTML source order.
*/
public HTMLCollection anchors;
/**
* Retrieves a collection of all applet objects in the document.
*/
public HTMLCollection applets;
/**
* Deprecated. Sets or retrieves a value that indicates the background color behind the object.
*/
public java.lang.String bgColor;
/**
* Specifies the beginning and end of the document body.
*/
public HTMLElement body;
public java.lang.String characterSet;
/**
* Gets or sets the character set used to encode the object.
*/
public java.lang.String charset;
/**
* Gets a value that indicates whether standards-compliant mode is switched on for the object.
*/
public java.lang.String compatMode;
public java.lang.String cookie;
/**
* Gets the default character set from the current regional language settings.
*/
public java.lang.String defaultCharset;
public Window defaultView;
/**
* Sets or gets a value that indicates whether the document can be edited.
*/
public java.lang.String designMode;
/**
* Sets or retrieves a value that indicates the reading order of the object.
*/
public java.lang.String dir;
/**
* Gets an object representing the document type declaration associated with the current document.
*/
public DocumentType doctype;
/**
* Gets a reference to the root node of the document.
*/
public HTMLElement documentElement;
/**
* Sets or gets the security domain of the document.
*/
public java.lang.String domain;
/**
* Retrieves a collection of all embed objects in the document.
*/
public HTMLCollection embeds;
/**
* Sets or gets the foreground (text) color of the document.
*/
public java.lang.String fgColor;
/**
* Retrieves a collection, in source order, of all form objects in the document.
*/
public HTMLCollection forms;
public Element fullscreenElement;
public java.lang.Boolean fullscreenEnabled;
public HTMLHeadElement head;
public java.lang.Boolean hidden;
/**
* Retrieves a collection, in source order, of img objects in the document.
*/
public HTMLCollection images;
/**
* Gets the implementation object of the current document.
*/
public DOMImplementation implementation;
/**
* Returns the character encoding used to create the webpage that is loaded into the document object.
*/
public java.lang.String inputEncoding;
/**
* Gets the date that the page was last modified, if the page supplies one.
*/
public java.lang.String lastModified;
/**
* Sets or gets the color of the document links.
*/
public java.lang.String linkColor;
/**
* Retrieves a collection of all a objects that specify the href property and all area objects in the document.
*/
public HTMLCollection links;
/**
* Contains information about the current URL.
*/
public Location location;
public java.lang.String media;
public java.lang.Boolean msCSSOMElementFloatMetrics;
public java.lang.Boolean msCapsLockWarningOff;
public java.lang.Boolean msHidden;
public java.lang.String msVisibilityState;
/**
* Fires when the user aborts the download.
* @param ev The event.
*/
public java.util.function.Function onabort;
/**
* Fires when the object is set as the active element.
* @param ev The event.
*/
public java.util.function.Function onactivate;
/**
* Fires immediately before the object is set as the active element.
* @param ev The event.
*/
public java.util.function.Function onbeforeactivate;
/**
* Fires immediately before the activeElement is changed from the current object to another object in the parent document.
* @param ev The event.
*/
public java.util.function.Function onbeforedeactivate;
/**
* Fires when the object loses the input focus.
* @param ev The focus event.
*/
public java.util.function.Function onblur;
/**
* Occurs when playback is possible, but would require further buffering.
* @param ev The event.
*/
public java.util.function.Function oncanplay;
public java.util.function.Function oncanplaythrough;
/**
* Fires when the contents of the object or selection have changed.
* @param ev The event.
*/
public java.util.function.Function onchange;
/**
* Fires when the user clicks the left mouse button on the object
* @param ev The mouse event.
*/
public java.util.function.Function onclick;
/**
* Fires when the user clicks the right mouse button in the client area, opening the context menu.
* @param ev The mouse event.
*/
public java.util.function.Function oncontextmenu;
/**
* Fires when the user double-clicks the object.
* @param ev The mouse event.
*/
public java.util.function.Function ondblclick;
/**
* Fires when the activeElement is changed from the current object to another object in the parent document.
* @param ev The UI Event
*/
public java.util.function.Function ondeactivate;
/**
* Fires on the source object continuously during a drag operation.
* @param ev The event.
*/
public java.util.function.Function ondrag;
/**
* Fires on the source object when the user releases the mouse at the close of a drag operation.
* @param ev The event.
*/
public java.util.function.Function ondragend;
/**
* Fires on the target element when the user drags the object to a valid drop target.
* @param ev The drag event.
*/
public java.util.function.Function ondragenter;
/**
* Fires on the target object when the user moves the mouse out of a valid drop target during a drag operation.
* @param ev The drag event.
*/
public java.util.function.Function ondragleave;
/**
* Fires on the target element continuously while the user drags the object over a valid drop target.
* @param ev The event.
*/
public java.util.function.Function ondragover;
/**
* Fires on the source object when the user starts to drag a text selection or selected object.
* @param ev The event.
*/
public java.util.function.Function ondragstart;
public java.util.function.Function ondrop;
/**
* Occurs when the duration attribute is updated.
* @param ev The event.
*/
public java.util.function.Function ondurationchange;
/**
* Occurs when the media element is reset to its initial state.
* @param ev The event.
*/
public java.util.function.Function onemptied;
/**
* Occurs when the end of playback is reached.
* @param ev The event
*/
public java.util.function.Function onended;
/**
* Fires when an error occurs during object loading.
* @param ev The event.
*/
public java.util.function.Function onerror;
/**
* Fires when the object receives focus.
* @param ev The event.
*/
public java.util.function.Function onfocus;
public java.util.function.Function onfullscreenchange;
public java.util.function.Function onfullscreenerror;
public java.util.function.Function oninput;
/**
* Fires when the user presses a key.
* @param ev The keyboard event
*/
public java.util.function.Function onkeydown;
/**
* Fires when the user presses an alphanumeric key.
* @param ev The event.
*/
public java.util.function.Function onkeypress;
/**
* Fires when the user releases a key.
* @param ev The keyboard event
*/
public java.util.function.Function onkeyup;
/**
* Fires immediately after the browser loads the object.
* @param ev The event.
*/
public java.util.function.Function onload;
/**
* Occurs when media data is loaded at the current playback position.
* @param ev The event.
*/
public java.util.function.Function onloadeddata;
/**
* Occurs when the duration and dimensions of the media have been determined.
* @param ev The event.
*/
public java.util.function.Function onloadedmetadata;
/**
* Occurs when Internet Explorer begins looking for media data.
* @param ev The event.
*/
public java.util.function.Function onloadstart;
/**
* Fires when the user clicks the object with either mouse button.
* @param ev The mouse event.
*/
public java.util.function.Function onmousedown;
/**
* Fires when the user moves the mouse over the object.
* @param ev The mouse event.
*/
public java.util.function.Function onmousemove;
/**
* Fires when the user moves the mouse pointer outside the boundaries of the object.
* @param ev The mouse event.
*/
public java.util.function.Function onmouseout;
/**
* Fires when the user moves the mouse pointer into the object.
* @param ev The mouse event.
*/
public java.util.function.Function onmouseover;
/**
* Fires when the user releases a mouse button while the mouse is over the object.
* @param ev The mouse event.
*/
public java.util.function.Function onmouseup;
/**
* Fires when the wheel button is rotated.
* @param ev The mouse event
*/
public java.util.function.Function onmousewheel;
public java.util.function.Function onmscontentzoom;
public java.util.function.Function onmsgesturechange;
public java.util.function.Function onmsgesturedoubletap;
public java.util.function.Function onmsgestureend;
public java.util.function.Function onmsgesturehold;
public java.util.function.Function onmsgesturestart;
public java.util.function.Function onmsgesturetap;
public java.util.function.Function onmsinertiastart;
public java.util.function.Function onmsmanipulationstatechanged;
public java.util.function.Function onmspointercancel;
public java.util.function.Function onmspointerdown;
public java.util.function.Function onmspointerenter;
public java.util.function.Function onmspointerleave;
public java.util.function.Function onmspointermove;
public java.util.function.Function onmspointerout;
public java.util.function.Function onmspointerover;
public java.util.function.Function onmspointerup;
/**
* Occurs when an item is removed from a Jump List of a webpage running in Site Mode.
* @param ev The event.
*/
public java.util.function.Function onmssitemodejumplistitemremoved;
/**
* Occurs when a user clicks a button in a Thumbnail Toolbar of a webpage running in Site Mode.
* @param ev The event.
*/
public java.util.function.Function onmsthumbnailclick;
/**
* Occurs when playback is paused.
* @param ev The event.
*/
public java.util.function.Function onpause;
/**
* Occurs when the play method is requested.
* @param ev The event.
*/
public java.util.function.Function onplay;
/**
* Occurs when the audio or video has started playing.
* @param ev The event.
*/
public java.util.function.Function onplaying;
public java.util.function.Function onpointerlockchange;
public java.util.function.Function onpointerlockerror;
/**
* Occurs to indicate progress while downloading media data.
* @param ev The event.
*/
public java.util.function.Function onprogress;
/**
* Occurs when the playback rate is increased or decreased.
* @param ev The event.
*/
public java.util.function.Function onratechange;
/**
* Fires when the state of the object has changed.
* @param ev The event
*/
public java.util.function.Function onreadystatechange;
/**
* Fires when the user resets a form.
* @param ev The event.
*/
public java.util.function.Function onreset;
/**
* Fires when the user repositions the scroll box in the scroll bar on the object.
* @param ev The event.
*/
public java.util.function.Function onscroll;
/**
* Occurs when the seek operation ends.
* @param ev The event.
*/
public java.util.function.Function onseeked;
/**
* Occurs when the current playback position is moved.
* @param ev The event.
*/
public java.util.function.Function onseeking;
/**
* Fires when the current selection changes.
* @param ev The event.
*/
public java.util.function.Function onselect;
public java.util.function.Function onselectstart;
/**
* Occurs when the download has stopped.
* @param ev The event.
*/
public java.util.function.Function onstalled;
/**
* Fires when the user clicks the Stop button or leaves the Web page.
* @param ev The event.
*/
public java.util.function.Function onstop;
public java.util.function.Function onsubmit;
/**
* Occurs if the load operation has been intentionally halted.
* @param ev The event.
*/
public java.util.function.Function onsuspend;
/**
* Occurs to indicate the current playback position.
* @param ev The event.
*/
public java.util.function.Function ontimeupdate;
public java.util.function.Function ontouchcancel;
public java.util.function.Function ontouchend;
public java.util.function.Function ontouchmove;
public java.util.function.Function ontouchstart;
/**
* Occurs when the volume is changed, or playback is muted or unmuted.
* @param ev The event.
*/
public java.util.function.Function onvolumechange;
/**
* Occurs when playback stops because the next frame of a video resource is not available.
* @param ev The event.
*/
public java.util.function.Function onwaiting;
public java.util.function.Function onwebkitfullscreenchange;
public java.util.function.Function onwebkitfullscreenerror;
public HTMLCollection plugins;
public Element pointerLockElement;
/**
* Retrieves a value that indicates the current state of the object.
*/
public java.lang.String readyState;
/**
* Gets the URL of the location that referred the user to the current page.
*/
public java.lang.String referrer;
/**
* Gets the root svg element in the document hierarchy.
*/
public SVGSVGElement rootElement;
/**
* Retrieves a collection of all script objects in the document.
*/
public HTMLCollection scripts;
public java.lang.String security;
/**
* Retrieves a collection of styleSheet objects representing the style sheets that correspond to each instance of a link or style object in the document.
*/
public StyleSheetList styleSheets;
/**
* Contains the title of the document.
*/
public java.lang.String title;
public java.lang.String visibilityState;
/**
* Sets or gets the color of the links that the user has visited.
*/
public java.lang.String vlinkColor;
public Element webkitCurrentFullScreenElement;
public Element webkitFullscreenElement;
public java.lang.Boolean webkitFullscreenEnabled;
public java.lang.Boolean webkitIsFullScreen;
public java.lang.String xmlEncoding;
public java.lang.Boolean xmlStandalone;
/**
* Gets or sets the version attribute specified in the declaration of an XML document.
*/
public java.lang.String xmlVersion;
native public Node adoptNode(Node source);
native public void captureEvents();
native public void clear();
/**
* Closes an output stream and forces the sent data to display.
*/
native public void close();
/**
* Creates an attribute object with a specified name.
* @param name String that sets the attribute object's name.
*/
native public Attr createAttribute(java.lang.String name);
native public Attr createAttributeNS(java.lang.String namespaceURI, java.lang.String qualifiedName);
native public CDATASection createCDATASection(java.lang.String data);
/**
* Creates a comment object with the specified data.
* @param data Sets the comment object's data.
*/
native public Comment createComment(java.lang.String data);
/**
* Creates a new document.
*/
native public DocumentFragment createDocumentFragment();
/**
* Creates an instance of the element for the specified tag.
* @param tagName The name of an element.
*/
native public HTMLAnchorElement createElement(jsweet.util.StringTypes.a tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.abbr tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.acronym tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.address tagName);
native public HTMLAppletElement createElement(jsweet.util.StringTypes.applet tagName);
native public HTMLAreaElement createElement(jsweet.util.StringTypes.area tagName);
native public HTMLAudioElement createElement(jsweet.util.StringTypes.audio tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.b tagName);
native public HTMLBaseElement createElement(jsweet.util.StringTypes.base tagName);
native public HTMLBaseFontElement createElement(jsweet.util.StringTypes.basefont tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.bdo tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.big tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.blockquote tagName);
native public HTMLBodyElement createElement(jsweet.util.StringTypes.body tagName);
native public HTMLBRElement createElement(jsweet.util.StringTypes.br tagName);
native public HTMLButtonElement createElement(jsweet.util.StringTypes.button tagName);
native public HTMLCanvasElement createElement(jsweet.util.StringTypes.canvas tagName);
native public HTMLTableCaptionElement createElement(jsweet.util.StringTypes.caption tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.center tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.cite tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.code tagName);
native public HTMLTableColElement createElement(jsweet.util.StringTypes.col tagName);
native public HTMLTableColElement createElement(jsweet.util.StringTypes.colgroup tagName);
native public HTMLDataListElement createElement(jsweet.util.StringTypes.datalist tagName);
native public HTMLDDElement createElement(jsweet.util.StringTypes.dd tagName);
native public HTMLModElement createElement(jsweet.util.StringTypes.del tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.dfn tagName);
native public HTMLDirectoryElement createElement(jsweet.util.StringTypes.dir tagName);
native public HTMLDivElement createElement(jsweet.util.StringTypes.div tagName);
native public HTMLDListElement createElement(jsweet.util.StringTypes.dl tagName);
native public HTMLDTElement createElement(jsweet.util.StringTypes.dt tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.em tagName);
native public HTMLEmbedElement createElement(jsweet.util.StringTypes.embed tagName);
native public HTMLFieldSetElement createElement(jsweet.util.StringTypes.fieldset tagName);
native public HTMLFontElement createElement(jsweet.util.StringTypes.font tagName);
native public HTMLFormElement createElement(jsweet.util.StringTypes.form tagName);
native public HTMLFrameElement createElement(jsweet.util.StringTypes.frame tagName);
native public HTMLFrameSetElement createElement(jsweet.util.StringTypes.frameset tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h1 tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h2 tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h3 tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h4 tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h5 tagName);
native public HTMLHeadingElement createElement(jsweet.util.StringTypes.h6 tagName);
native public HTMLHeadElement createElement(jsweet.util.StringTypes.head tagName);
native public HTMLHRElement createElement(jsweet.util.StringTypes.hr tagName);
native public HTMLHtmlElement createElement(jsweet.util.StringTypes.html tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.i tagName);
native public HTMLIFrameElement createElement(jsweet.util.StringTypes.iframe tagName);
native public HTMLImageElement createElement(jsweet.util.StringTypes.img tagName);
native public HTMLInputElement createElement(jsweet.util.StringTypes.input tagName);
native public HTMLModElement createElement(jsweet.util.StringTypes.ins tagName);
native public HTMLIsIndexElement createElement(jsweet.util.StringTypes.isindex tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.kbd tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.keygen tagName);
native public HTMLLabelElement createElement(jsweet.util.StringTypes.label tagName);
native public HTMLLegendElement createElement(jsweet.util.StringTypes.legend tagName);
native public HTMLLIElement createElement(jsweet.util.StringTypes.li tagName);
native public HTMLLinkElement createElement(jsweet.util.StringTypes.link tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.listing tagName);
native public HTMLMapElement createElement(jsweet.util.StringTypes.map tagName);
native public HTMLMarqueeElement createElement(jsweet.util.StringTypes.marquee tagName);
native public HTMLMenuElement createElement(jsweet.util.StringTypes.menu tagName);
native public HTMLMetaElement createElement(jsweet.util.StringTypes.meta tagName);
native public HTMLNextIdElement createElement(jsweet.util.StringTypes.nextid tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.nobr tagName);
native public HTMLObjectElement createElement(jsweet.util.StringTypes.object tagName);
native public HTMLOListElement createElement(jsweet.util.StringTypes.ol tagName);
native public HTMLOptGroupElement createElement(jsweet.util.StringTypes.optgroup tagName);
native public HTMLOptionElement createElement(jsweet.util.StringTypes.option tagName);
native public HTMLParagraphElement createElement(jsweet.util.StringTypes.p tagName);
native public HTMLParamElement createElement(jsweet.util.StringTypes.param tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.plaintext tagName);
native public HTMLPreElement createElement(jsweet.util.StringTypes.pre tagName);
native public HTMLProgressElement createElement(jsweet.util.StringTypes.progress tagName);
native public HTMLQuoteElement createElement(jsweet.util.StringTypes.q tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.rt tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.ruby tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.s tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.samp tagName);
native public HTMLScriptElement createElement(jsweet.util.StringTypes.script tagName);
native public HTMLSelectElement createElement(jsweet.util.StringTypes.select tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.small tagName);
native public HTMLSourceElement createElement(jsweet.util.StringTypes.source tagName);
native public HTMLSpanElement createElement(jsweet.util.StringTypes.span tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.strike tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.strong tagName);
native public HTMLStyleElement createElement(jsweet.util.StringTypes.style tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.sub tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.sup tagName);
native public HTMLTableElement createElement(jsweet.util.StringTypes.table tagName);
native public HTMLTableSectionElement createElement(jsweet.util.StringTypes.tbody tagName);
native public HTMLTableDataCellElement createElement(jsweet.util.StringTypes.td tagName);
native public HTMLTextAreaElement createElement(jsweet.util.StringTypes.textarea tagName);
native public HTMLTableSectionElement createElement(jsweet.util.StringTypes.tfoot tagName);
native public HTMLTableHeaderCellElement createElement(jsweet.util.StringTypes.th tagName);
native public HTMLTableSectionElement createElement(jsweet.util.StringTypes.thead tagName);
native public HTMLTitleElement createElement(jsweet.util.StringTypes.title tagName);
native public HTMLTableRowElement createElement(jsweet.util.StringTypes.tr tagName);
native public HTMLTrackElement createElement(jsweet.util.StringTypes.track tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.tt tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.u tagName);
native public HTMLUListElement createElement(jsweet.util.StringTypes.ul tagName);
native public HTMLPhraseElement createElement(jsweet.util.StringTypes.Var tagName);
native public HTMLVideoElement createElement(jsweet.util.StringTypes.video tagName);
native public MSHTMLWebViewElement createElement(jsweet.util.StringTypes.x_ms_webview tagName);
native public HTMLBlockElement createElement(jsweet.util.StringTypes.xmp tagName);
native public HTMLElement createElement(java.lang.String tagName);
native public Element createElementNS(java.lang.String namespaceURI, java.lang.String qualifiedName);
native public XPathExpression createExpression(java.lang.String expression, XPathNSResolver resolver);
native public XPathNSResolver createNSResolver(Node nodeResolver);
/**
* Creates a NodeIterator object that you can use to traverse filtered lists of nodes or elements in a document.
* @param root The root element or node to start traversing on.
* @param whatToShow The type of nodes or elements to appear in the node list
* @param filter A custom NodeFilter function to use. For more information, see filter. Use null for no filter.
* @param entityReferenceExpansion A flag that specifies whether entity reference nodes are expanded.
*/
native public NodeIterator createNodeIterator(Node root, double whatToShow, NodeFilter filter, java.lang.Boolean entityReferenceExpansion);
native public ProcessingInstruction createProcessingInstruction(java.lang.String target, java.lang.String data);
/**
* Returns an empty range object that has both of its boundary points positioned at the beginning of the document.
*/
native public Range createRange();
/**
* Creates a text string from the specified value.
* @param data String that specifies the nodeValue property of the text node.
*/
native public Text createTextNode(java.lang.String data);
native public Touch createTouch(java.lang.Object view, EventTarget target, double identifier, double pageX, double pageY, double screenX, double screenY);
native public TouchList createTouchList(Touch... touches);
/**
* Creates a TreeWalker object that you can use to traverse filtered lists of nodes or elements in a document.
* @param root The root element or node to start traversing on.
* @param whatToShow The type of nodes or elements to appear in the node list. For more information, see whatToShow.
* @param filter A custom NodeFilter function to use.
* @param entityReferenceExpansion A flag that specifies whether entity reference nodes are expanded.
*/
native public TreeWalker createTreeWalker(Node root, double whatToShow, NodeFilter filter, java.lang.Boolean entityReferenceExpansion);
/**
* Returns the element for the specified x coordinate and the specified y coordinate.
* @param x The x-offset
* @param y The y-offset
*/
native public Element elementFromPoint(double x, double y);
native public XPathResult evaluate(java.lang.String expression, Node contextNode, XPathNSResolver resolver, double type, XPathResult result);
/**
* Executes a command on the current document, current selection, or the given range.
* @param commandId String that specifies the command to execute. This command can be any of the command identifiers that can be executed in script.
* @param showUI Display the user interface, defaults to false.
* @param value Value to assign.
*/
native public java.lang.Boolean execCommand(java.lang.String commandId, java.lang.Boolean showUI, java.lang.Object value);
/**
* Displays help information for the given command identifier.
* @param commandId Displays help information for the given command identifier.
*/
native public java.lang.Boolean execCommandShowHelp(java.lang.String commandId);
native public void exitFullscreen();
native public void exitPointerLock();
/**
* Causes the element to receive the focus and executes the code specified by the onfocus event.
*/
native public void focus();
/**
* Returns a reference to the first object with the specified value of the ID or NAME attribute.
* @param elementId String that specifies the ID value. Case-insensitive.
*/
native public HTMLElement getElementById(java.lang.String elementId);
native public NodeList getElementsByClassName(java.lang.String classNames);
/**
* Gets a collection of objects based on the value of the NAME or ID attribute.
* @param elementName Gets a collection of objects based on the value of the NAME or ID attribute.
*/
native public NodeList getElementsByName(java.lang.String elementName);
/**
* Retrieves a collection of objects based on the specified element name.
* @param name Specifies the name of an element.
*/
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.a tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.abbr tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.acronym tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.address tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.applet tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.area tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.article tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.aside tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.audio tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.b tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.base tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.basefont tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.bdo tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.big tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.blockquote tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.body tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.br tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.button tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.canvas tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.caption tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.center tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.circle tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.cite tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.clippath tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.code tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.col tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.colgroup tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.datalist tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.dd tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.defs tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.del tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.desc tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.dfn tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.dir tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.div tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.dl tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.dt tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.ellipse tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.em tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.embed tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feblend tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fecolormatrix tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fecomponenttransfer tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fecomposite tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feconvolvematrix tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fediffuselighting tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fedisplacementmap tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fedistantlight tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feflood tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fefunca tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fefuncb tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fefuncg tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fefuncr tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fegaussianblur tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feimage tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.femerge tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.femergenode tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.femorphology tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feoffset tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fepointlight tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fespecularlighting tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fespotlight tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fetile tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.feturbulence tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.fieldset tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.figcaption tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.figure tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.filter tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.font tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.footer tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.foreignobject tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.form tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.frame tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.frameset tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.g tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h1 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h2 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h3 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h4 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h5 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.h6 tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.head tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.header tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.hgroup tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.hr tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.html tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.i tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.iframe tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.image tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.img tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.input tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.ins tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.isindex tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.kbd tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.keygen tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.label tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.legend tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.li tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.line tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.lineargradient tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.link tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.listing tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.map tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.mark tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.marker tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.marquee tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.mask tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.menu tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.meta tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.metadata tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.nav tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.nextid tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.nobr tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.noframes tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.noscript tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.object tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.ol tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.optgroup tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.option tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.p tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.param tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.path tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.pattern tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.plaintext tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.polygon tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.polyline tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.pre tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.progress tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.q tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.radialgradient tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.rect tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.rt tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.ruby tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.s tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.samp tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.script tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.section tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.select tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.small tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.source tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.span tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.stop tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.strike tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.strong tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.style tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.sub tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.sup tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.svg tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.Switch tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.symbol tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.table tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.tbody tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.td tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.text tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.textpath tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.textarea tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.tfoot tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.th tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.thead tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.title tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.tr tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.track tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.tspan tagname);
native public HTMLCollectionOf getElementsByTagName(jsweet.util.StringTypes.tt tagname);
native public HTMLCollectionOf