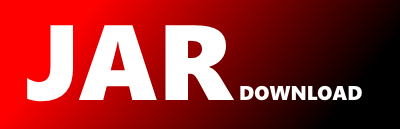
def.js.Promise Maven / Gradle / Ivy
The newest version!
package def.js;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import jsweet.lang.Name;
import jsweet.util.Lang;
/**
* Represents the completion of an asynchronous operation.
* Warning: in JSweet, this definition is slightly modifed because of
* Java type erasure & modified keywords. For instance catch
is
* Catch, then
with chained Promise is thenAsync.
*
* Note: this class could be used along with {@link Lang#async} / {@link Lang#await}. This is sweet!
*
* Example:
*
* getAnswer(5000)
.then(result -> {
console.log("you have waited long enough to know that the answer is: " + result);
});
private Promise getAnswer(int millis) {
return new Promise((Consumer resolve, Consumer
*/
public class Promise extends PromiseLike {
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
@jsweet.lang.Name("then")
native public Promise then(Function onfulfilled,
Function onrejected);
@jsweet.lang.Name("then")
native public Promise then(Function onfulfilled,
java.util.function.Consumer onrejected);
/**
* Attaches a callback for only the rejection of the Promise.
*
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of the callback.
*/
@jsweet.lang.Name("catch")
native public Promise Catch(Function onrejected);
@jsweet.lang.Name("catch")
native public Promise Catch(Consumer onrejected);
native public java.lang.String $get(Symbol toStringTag);
/**
* A reference to the prototype.
*/
public static Promise> prototype;
/**
* Creates a Promise that is resolved with an array of results when all of the
* provided Promises resolve, or rejected when any Promise is rejected.
*
* @param values
* An array of Promises.
* @returns A new Promise.
*/
@Name("all")
native public static Promise allFromValues(Iterable values);
/**
* Creates a Promise that is resolved with an array of results when all of the
* provided Promises resolve, or rejected when any Promise is rejected.
*
* @param values
* An array of Promises.
* @returns A new Promise.
*/
native public static Promise all(Iterable> values);
/**
* Creates a Promise that is resolved or rejected when any of the provided
* Promises are resolved or rejected.
*
* @param values
* An array of Promises.
* @returns A new Promise.
*/
native public static Promise race(IterableT values);
/**
* Creates a new rejected promise for the provided reason.
*
* @param reason
* The reason the promise was rejected.
* @returns A new rejected Promise.
*/
native public static Promise reject(java.lang.Object reason);
/**
* Creates a new resolved promise for the provided value.
*
* @param value
* A promise.
* @returns A promise whose internal state matches the provided promise.
*/
native public static Promise resolve(T value);
/**
* Creates a new resolved promise .
*
* @returns A resolved promise.
*/
native public static Promise resolve();
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
native public Promise then(Function onfulfilled);
native public Promise then(Supplier onfulfilled);
native public Promise then(Runnable onfulfilled);
native public Promise then(Consumer onfulfilled);
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
@jsweet.lang.Name("then")
native public Promise thenAsyncOnError(Function onfulfilled,
Function> onrejected);
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
@jsweet.lang.Name("then")
native public Promise thenAsyncWithOnError(Function> onfulfilled,
Function> onrejected);
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
@jsweet.lang.Name("then")
native public Promise thenAsync(Function> onfulfilled,
Function onrejected);
@jsweet.lang.Name("then")
native public Promise thenAsync(Supplier> onfulfilled);
@jsweet.lang.Name("then")
native public Promise thenAsync(Function> onfulfilled,
java.util.function.Consumer onrejected);
/**
* Attaches a callback for only the rejection of the Promise.
*
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of the callback.
*/
@jsweet.lang.Name("catch")
native public Promise catchAsync(Function> onrejected);
/**
* Creates a new Promise.
*
* @param executor
* A callback used to initialize the promise. This callback is passed
* two arguments: a resolve callback used resolve the promise with a
* value or the result of another promise, and a reject callback used
* to reject the promise with a provided reason or error.
*/
public Promise(
ExecutorPromiseLikeBiConsumer>, java.util.function.Consumer> executor) {
}
/**
* Creates a new Promise.
*
* @param executor
* A callback used to initialize the promise. This callback is passed
* two arguments: a resolve callback used resolve the promise with a
* value or the result of another promise, and a reject callback used
* to reject the promise with a provided reason or error.
*/
public Promise(BiConsumer, java.util.function.Consumer> executor) {
}
/**
* Creates a Promise that is resolved or rejected when any of the provided
* Promises are resolved or rejected.
*
* @param values
* An array of Promises.
* @returns A new Promise.
*/
native public static Promise race(IterablePromiseLikeT values);
/**
* Creates a new resolved promise for the provided value.
*
* @param value
* A promise.
* @returns A promise whose internal state matches the provided promise.
*/
native public static Promise resolve(PromiseLike value);
/**
* Attaches callbacks for the resolution and/or rejection of the Promise.
*
* @param onfulfilled
* The callback to execute when the Promise is resolved.
* @param onrejected
* The callback to execute when the Promise is rejected.
* @returns A Promise for the completion of which ever callback is executed.
*/
@jsweet.lang.Name("then")
native public Promise thenAsync(Function> onfulfilled);
protected Promise() {
}
/**
* This functional interface should be used for disambiguating lambdas in
* function parameters (by casting to this interface).
*
* It was automatically generated for functions (taking lambdas) that lead to
* the same erased signature.
*/
@java.lang.FunctionalInterface()
@jsweet.lang.Erased
public interface ExecutorPromiseLikeBiConsumer {
public void $apply(T1 p1, T2 p2);
}
/**
* This class was automatically generated for disambiguating erased method
* signatures.
*/
@jsweet.lang.Erased
public static class IterableT extends def.js.Object {
public IterableT(Iterable values) {
}
}
/**
* This class was automatically generated for disambiguating erased method
* signatures.
*/
@jsweet.lang.Erased
public static class IterablePromiseLikeT extends def.js.Object {
public IterablePromiseLikeT(Iterable> values) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy