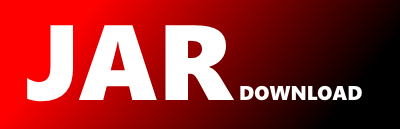
org.jtrim2.collections.Equality Maven / Gradle / Ivy
package org.jtrim2.collections;
import java.util.Objects;
/**
* Defines static factory methods for {@link EqualityComparator} instances.
*/
public final class Equality {
/**
* Returns an {@code EqualityComparator} which compares objects based on
* their {@link Object#equals(Object) equals} method. That is, the
* comparator returns the same value for the comparison as the static
* method {@link java.util.Objects#equals(Object, Object)}.
*
* @return an {@code EqualityComparator} which compares objects based on
* their {@link Object#equals(Object) equals} method. This method never
* returns {@code null}.
*/
public static EqualityComparator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy