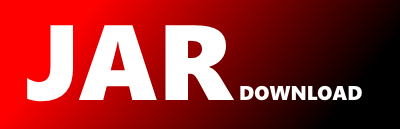
org.jtrim2.executor.ContextAwareWrapper Maven / Gradle / Ivy
package org.jtrim2.executor;
import java.util.Objects;
import java.util.concurrent.CompletionStage;
import org.jtrim2.cancel.CancellationToken;
/**
* A {@code TaskExecutor} implementation forwarding tasks to another task
* executor an being able to determine if tasks submitted to the
* {@code ContextAwareWrapper} run in the the context of the same
* {@code ContextAwareWrapper}.
*
* It is also possible to create a task executor which shares execution context
* with the {@code ContextAwareWrapper} via the
* {@link #sameContextExecutor(TaskExecutor) sameContextExecutor} method.
*
* This class may only be instantiated by the static factory methods in
* {@link TaskExecutors}:
* {@link TaskExecutors#contextAware(TaskExecutor) TaskExecutors.contextAware} and
* {@link TaskExecutors#contextAwareIfNecessary(TaskExecutor) TaskExecutors.contextAwareIfNecessary}.
*
*
Thread safety
* Methods of this class are safely accessible from multiple threads
* concurrently.
*
* Synchronization transparency
* Method of this class are not synchronization transparent unless
* otherwise noted.
*
* @see TaskExecutors#contextAware(TaskExecutor)
* @see TaskExecutors#contextAwareIfNecessary(TaskExecutor)
*/
public final class ContextAwareWrapper implements ContextAwareTaskExecutor {
private final TaskExecutor wrapped;
// null if not in context, running in context otherwise.
private final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy