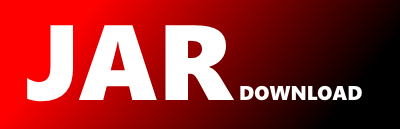
org.jtrim2.executor.DoneFuture Maven / Gradle / Ivy
package org.jtrim2.executor;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
/**
* Defines a future of an already done task. No underlying task is actually
* necessary, only the result of the (possibly not existent) task. This future
* is not cancelable, attempting to cancel it does nothing.
*
* Thread safety
* Methods of this class are safe to use by multiple threads concurrently.
*
* Synchronization transparency
* The methods of this class are synchronization transparent.
*
* @param the type of the result of this future
*/
public final class DoneFuture implements Future {
private final ResultType result;
/**
* Creates a new {@code DoneFuture} with the given result.
* The specified result will be returned by the {@code get} methods without
* actually waiting.
*
* @param result the object to be returned by the {@code get} methods. This
* argument can be {@code null}.
*/
public DoneFuture(ResultType result) {
this.result = result;
}
/**
* This method does nothing but returns {@code false}.
*
* @param mayInterruptIfRunning this argument is ignored
* @return {@code false}
*/
@Override
public boolean cancel(boolean mayInterruptIfRunning) {
return false;
}
/**
* This method does nothing but returns {@code false}.
*
* @return {@code false}
*/
@Override
public boolean isCancelled() {
return false;
}
/**
* This method does nothing but returns {@code true}.
*
* @return {@code true}
*/
@Override
public boolean isDone() {
return true;
}
/**
* This method returns the result specified at construction time.
*
* @return the object specified at construction time which can also be
* {@code null}
*/
@Override
public ResultType get() {
return result;
}
/**
* This method returns the result specified at construction time.
*
* @param timeout this argument is ignored
* @param unit this argument is ignored
* @return the object specified at construction time which can also be
* {@code null}
*/
@Override
public ResultType get(long timeout, TimeUnit unit) {
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy