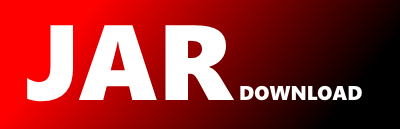
org.jtrim2.taskgraph.basic.CollectingTaskGraphDefConfigurer Maven / Gradle / Ivy
package org.jtrim2.taskgraph.basic;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import org.jtrim2.taskgraph.TaskFactoryConfig;
import org.jtrim2.taskgraph.TaskFactoryDefiner;
import org.jtrim2.taskgraph.TaskFactoryGroupConfigurer;
import org.jtrim2.taskgraph.TaskFactoryKey;
import org.jtrim2.taskgraph.TaskFactorySetup;
import org.jtrim2.taskgraph.TaskGraphBuilder;
import org.jtrim2.taskgraph.TaskGraphDefConfigurer;
/**
* Defines a simple implementation of {@code TaskGraphDefConfigurer} which collects the
* added task factory definitions and simply passes them to a given {@link TaskGraphBuilderFactory}.
*
* Thread safety
* The methods of this class may not be used by multiple threads concurrently, unless otherwise noted.
*
* Synchronization transparency
* The methods of this class are not synchronization transparent in general.
*
* @see CollectingTaskGraphBuilder
* @see RestrictableTaskGraphExecutor
*/
public final class CollectingTaskGraphDefConfigurer implements TaskGraphDefConfigurer {
private final TaskGraphBuilderFactory graphBuilderFactory;
private final Map, TaskFactoryConfig, ?>> factoryDefs;
/**
* Creates a new {@code CollectingTaskGraphDefConfigurer} with the given
* {@code TaskGraphBuilderFactory} to be called when the {@link #build() build()}
* method is called.
*
* @param graphBuilderFactory the {@code TaskGraphBuilderFactory} to be called when
* the {@code build()} method is called. This argument cannot be {@code null}.
*/
public CollectingTaskGraphDefConfigurer(TaskGraphBuilderFactory graphBuilderFactory) {
Objects.requireNonNull(graphBuilderFactory, "graphBuilderFactory");
this.graphBuilderFactory = graphBuilderFactory;
this.factoryDefs = new ConcurrentHashMap<>();
}
/**
* {@inheritDoc }
*
* This implementation allows this method to be called concurrently and also
* allows concurrent use of the returned {@code TaskFactoryDefiner}. However,
* none of them might be used concurrently with the {@link #build() build()}
* method call.
*/
@Override
public TaskFactoryDefiner factoryGroupDefiner(TaskFactoryGroupConfigurer groupConfigurer) {
return new GroupDefiner(factoryDefs, groupConfigurer);
}
/**
* {@inheritDoc }
*/
@Override
public TaskGraphBuilder build() {
return graphBuilderFactory.createGraphBuilder(
Collections.unmodifiableCollection(factoryDefs.values()));
}
private static final class GroupDefiner implements TaskFactoryDefiner {
private final Map, TaskFactoryConfig, ?>> factoryDefs;
private final TaskFactoryGroupConfigurer groupConfigurer;
public GroupDefiner(
Map, TaskFactoryConfig, ?>> factoryDefs,
TaskFactoryGroupConfigurer groupConfigurer) {
this.factoryDefs = factoryDefs;
this.groupConfigurer = groupConfigurer;
}
@Override
public TaskFactoryConfig defineFactory(
TaskFactoryKey defKey,
TaskFactorySetup setup) {
TaskFactoryConfig config = new TaskFactoryConfig<>(defKey, groupConfigurer, setup);
@SuppressWarnings("unchecked")
TaskFactoryConfig prev = (TaskFactoryConfig) factoryDefs.put(defKey, config);
return prev;
}
}
}