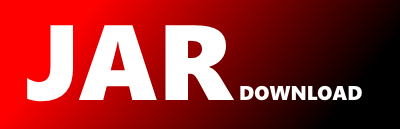
org.jtwig.reflection.model.java.JavaClass Maven / Gradle / Ivy
package org.jtwig.reflection.model.java;
import com.google.common.base.Optional;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
public class JavaClass {
private final Class nativeType;
private final Map constants;
private final Map fields;
private final Map methodsByName;
public JavaClass(Class nativeType, Map constants, Map fields, Map methodsByName) {
this.nativeType = nativeType;
this.constants = constants;
this.fields = fields;
this.methodsByName = methodsByName;
}
public Optional constant (String name) {
return Optional.fromNullable(constants.get(name));
}
public Collection constants () {
return constants.values();
}
public Optional field (String name) {
return Optional.fromNullable(fields.get(name));
}
public Collection fields () {
return fields.values();
}
public JavaMethods method (String name) {
return Optional.fromNullable(methodsByName.get(name))
.or(new JavaMethods(Collections.emptyMap()));
}
public void merge (JavaClass origin) {
for (Map.Entry entry : origin.methodsByName.entrySet()) {
if (methodsByName.containsKey(entry.getKey())) {
methodsByName.get(entry.getKey()).merge(origin.methodsByName.get(entry.getKey()));
} else {
methodsByName.put(entry.getKey(), entry.getValue());
}
}
for (Map.Entry entry : origin.fields.entrySet()) {
if (!fields.containsKey(entry.getKey())) {
fields.put(entry.getKey(), entry.getValue());
}
}
for (Map.Entry entry : constants.entrySet()) {
if (!constants.containsKey(entry.getKey())) {
constants.put(entry.getKey(), entry.getValue());
}
}
}
public Class getNative() {
return nativeType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy