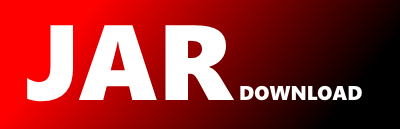
org.jtwig.reflection.model.java.JavaClassFactory Maven / Gradle / Ivy
package org.jtwig.reflection.model.java;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.Map;
public class JavaClassFactory {
public JavaClass create(Class type) {
Map constants = new HashMap();
Map fields = new HashMap();
Map> methodsByName = new HashMap>();
Map javaMethods = new HashMap();
extractFields(type, constants, fields);
extractMethods(type, methodsByName);
for (Map.Entry> entry : methodsByName.entrySet()) {
javaMethods.put(entry.getKey(), new JavaMethods(entry.getValue()));
}
JavaClass result = new JavaClass(type, constants, fields, javaMethods);
if (type.getSuperclass() != Object.class) {
JavaClass javaClass = create(type.getSuperclass());
result.merge(javaClass);
}
return result;
}
private void extractMethods(Class type, Map> methodsByName) {
for (Method method : type.getDeclaredMethods()) {
if (!Modifier.isStatic(method.getModifiers())) {
JavaMethod javaMethod = new JavaMethod(method);
if (!methodsByName.containsKey(method.getName())) {
methodsByName.put(method.getName(), new HashMap());
}
methodsByName.get(method.getName()).put(new MethodSignature(method.getParameterTypes()), javaMethod);
}
}
}
private void extractFields(Class type, Map constants, Map fields) {
for (Field field : type.getDeclaredFields()) {
if (Modifier.isStatic(field.getModifiers())) {
constants.put(field.getName(), new JavaConstant(field));
} else {
fields.put(field.getName(), new JavaField(field));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy