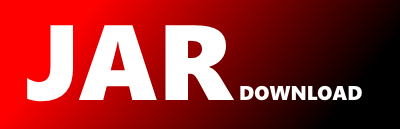
org.jtwig.reflection.model.java.JavaMethod Maven / Gradle / Ivy
package org.jtwig.reflection.model.java;
import com.google.common.base.Optional;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
public class JavaMethod {
private final Method method;
private List arguments;
public JavaMethod(Method method) {
this.method = method;
this.method.setAccessible(true);
}
public List arguments() {
if (arguments == null) {
List result = new ArrayList();
Class>[] parameterTypes = method.getParameterTypes();
for (int i = 0; i < parameterTypes.length; i++) {
result.add(new JavaMethodArgument(this, i));
}
arguments = result;
}
return arguments;
}
public Object invoke(Object bean, Object[] arguments) throws InvocationTargetException, IllegalAccessException {
return method.invoke(bean, arguments);
}
public Class type(int position) {
return method.getParameterTypes()[position];
}
public boolean isVarArgs() {
return method.isVarArgs();
}
public int numberOfArguments() {
return method.getParameterTypes().length;
}
public Optional argumentAnnotation(int position, Class type) {
Annotation[] annotations = method.getParameterAnnotations()[position];
for (Annotation annotation : annotations) {
if (annotation.annotationType().equals(type)) {
return Optional.of(type.cast(annotation));
}
}
return Optional.absent();
}
public Optional annotation(Class type) {
Annotation[] annotations = method.getAnnotations();
for (Annotation annotation : annotations) {
if (annotation.annotationType().equals(type)) {
return Optional.of(type.cast(annotation));
}
}
return Optional.absent();
}
public String name () {
return method.getName();
}
public Method getNative () {
return method;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy