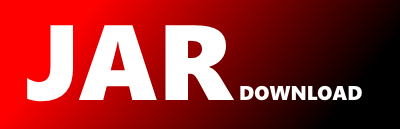
org.junit.gen5.commons.util.CollectionUtils Maven / Gradle / Ivy
Show all versions of junit-commons Show documentation
/*
* Copyright 2015-2016 the original author or authors.
*
* All rights reserved. This program and the accompanying materials are
* made available under the terms of the Eclipse Public License v1.0 which
* accompanies this distribution and is available at
*
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.junit.gen5.commons.util;
import static org.junit.gen5.commons.meta.API.Usage.Internal;
import java.util.Collection;
import org.junit.gen5.commons.meta.API;
/**
* Collection of utilities for working with {@link Collection Collections}.
*
* DISCLAIMER
*
* These utilities are intended solely for usage within the JUnit framework
* itself. Any usage by external parties is not supported.
* Use at your own risk!
*
* @since 5.0
*/
@API(Internal)
public final class CollectionUtils {
private CollectionUtils() {
/* no-op */
}
/**
* Read the only element of a collection of size 1.
*
* @param collection the collection to get the element from
* @return the only element of the collection
* @throws PreconditionViolationException if the collection is {@code null}
* or does not contain exactly one element
*/
public static T getOnlyElement(Collection collection) {
Preconditions.notNull(collection, "collection must not be null");
Preconditions.condition(collection.size() == 1,
() -> "collection must contain exactly one element: " + collection);
return collection.iterator().next();
}
}