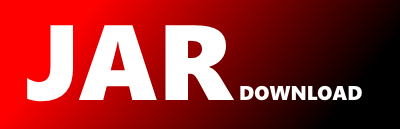
org.junit.gen5.console.options.AvailableOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-console Show documentation
Show all versions of junit-console Show documentation
Module "junit-console" of JUnit 5.
The newest version!
/*
* Copyright 2015-2016 the original author or authors.
*
* All rights reserved. This program and the accompanying materials are
* made available under the terms of the Eclipse Public License v1.0 which
* accompanies this distribution and is available at
*
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.junit.gen5.console.options;
import static java.util.Arrays.asList;
import joptsimple.OptionParser;
import joptsimple.OptionSet;
import joptsimple.OptionSpec;
class AvailableOptions {
private final OptionParser parser = new OptionParser();
private final OptionSpec help;
private final OptionSpec enableExitCode;
private final OptionSpec disableAnsiColors;
private final OptionSpec runAllTests;
private final OptionSpec hideDetails;
private final OptionSpec classnameFilter;
private final OptionSpec requiredTagsFilter;
private final OptionSpec excludedTagsFilter;
private final OptionSpec additionalClasspathEntries;
private final OptionSpec xmlReportsDir;
private final OptionSpec arguments;
AvailableOptions() {
runAllTests = parser.acceptsAll(asList("a", "all"), //
"Run all tests");
additionalClasspathEntries = parser.acceptsAll(asList("p", "classpath"), //
"Additional classpath entries, e.g. for adding engines and their dependencies") //
.withRequiredArg();
classnameFilter = parser.acceptsAll(asList("n", "filter-classname"),
"Give a regular expression to include only classes whose fully qualified names match.") //
.withRequiredArg();
requiredTagsFilter = parser.acceptsAll(asList("t", "require-tag"),
"Give a tag to be required in the test run. This option can be repeated.") //
.withRequiredArg();
excludedTagsFilter = parser.acceptsAll(asList("T", "exclude-tag"),
"Give a tag to exclude from the test run. This option can be repeated.") //
.withRequiredArg();
xmlReportsDir = parser.acceptsAll(asList("r", "xml-reports-dir"), //
"Enable XML report output into a specified local directory (will be created if it does not exist)") //
.withRequiredArg();
enableExitCode = parser.acceptsAll(asList("x", "enable-exit-code"), //
"Exit process with number of failing tests as exit code");
disableAnsiColors = parser.acceptsAll(asList("C", "disable-ansi-colors"),
"Disable colored output (not supported by all terminals)");
hideDetails = parser.acceptsAll(asList("D", "hide-details"),
"Hide details while tests are being executed. Only show the summary and test failures.");
help = parser.acceptsAll(asList("h", "help"), //
"Display help information");
arguments = parser.nonOptions("Test classes, methods or packages to execute. If --all|-a has been chosen, "
+ "arguments can list all classpath roots that should be considered for test scanning, "
+ "or none if the full classpath shall be scanned.");
}
OptionParser getParser() {
return parser;
}
CommandLineOptions toCommandLineOptions(OptionSet detectedOptions) {
CommandLineOptions result = new CommandLineOptions();
result.setDisplayHelp(detectedOptions.has(help));
result.setExitCodeEnabled(detectedOptions.has(enableExitCode));
result.setAnsiColorOutputDisabled(detectedOptions.has(disableAnsiColors));
result.setRunAllTests(detectedOptions.has(runAllTests));
result.setHideDetails(detectedOptions.has(hideDetails));
result.setClassnameFilter(detectedOptions.valueOf(classnameFilter));
result.setRequiredTagsFilter(detectedOptions.valuesOf(requiredTagsFilter));
result.setExcludedTagsFilter(detectedOptions.valuesOf(excludedTagsFilter));
result.setAdditionalClasspathEntries(detectedOptions.valuesOf(additionalClasspathEntries));
result.setXmlReportsDir(detectedOptions.valueOf(xmlReportsDir));
result.setArguments(detectedOptions.valuesOf(arguments));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy