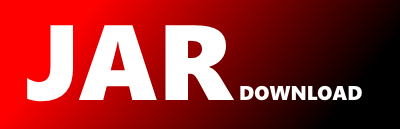
org.junit.gen5.launcher.TestIdentifier Maven / Gradle / Ivy
Show all versions of junit-launcher Show documentation
/*
* Copyright 2015-2016 the original author or authors.
*
* All rights reserved. This program and the accompanying materials are
* made available under the terms of the Eclipse Public License v1.0 which
* accompanies this distribution and is available at
*
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.junit.gen5.launcher;
import static java.util.Collections.unmodifiableSet;
import static org.junit.gen5.commons.meta.API.Usage.Experimental;
import java.io.Serializable;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import org.junit.gen5.commons.meta.API;
import org.junit.gen5.engine.TestDescriptor;
import org.junit.gen5.engine.TestSource;
import org.junit.gen5.engine.TestTag;
/**
* Immutable data transfer object that represents a test or container which is
* usually part of a {@link TestPlan}.
*
* @since 5.0
* @see TestPlan
*/
@API(Experimental)
public final class TestIdentifier implements Serializable {
private static final long serialVersionUID = 1L;
private final TestId uniqueId;
private final String name;
private final String displayName;
private final TestSource source;
private final Set tags;
private final boolean test;
private final boolean container;
private final TestId parentId;
public static TestIdentifier from(TestDescriptor testDescriptor) {
// TODO Use Flyweight Pattern for TestId?
TestId uniqueId = new TestId(testDescriptor.getUniqueId());
String name = testDescriptor.getName();
String displayName = testDescriptor.getDisplayName();
Optional source = testDescriptor.getSource();
Set tags = testDescriptor.getTags();
boolean test = testDescriptor.isTest();
boolean container = !test || !testDescriptor.getChildren().isEmpty();
Optional parentId = testDescriptor.getParent().map(TestDescriptor::getUniqueId).map(TestId::new);
return new TestIdentifier(uniqueId, name, displayName, source, tags, test, container, parentId);
}
private TestIdentifier(TestId uniqueId, String name, String displayName, Optional source,
Set tags, boolean test, boolean container, Optional parentId) {
this.uniqueId = uniqueId;
this.name = name;
this.displayName = displayName;
this.source = source.orElse(null);
this.tags = unmodifiableSet(new LinkedHashSet<>(tags));
this.test = test;
this.container = container;
this.parentId = parentId.orElse(null);
}
/**
* Get the unique ID of the represented test or container.
*
* Uniqueness must be guaranteed across an entire
* {@linkplain TestPlan test plan}, regardless of how many engines are used
* behind the scenes.
*/
public TestId getUniqueId() {
return uniqueId;
}
/**
* Get the name for the represented test or container.
*
*
The name is a technical name for a test or container. It
* must not be parsed or processed besides being displayed to end-users.
*/
public String getName() {
return name;
}
/**
* Get the display name for the represented test or container.
*
*
The display name is a human-readable name for a test or
* container. It must not be parsed or processed besides being displayed
* to end-users.
*/
public String getDisplayName() {
return displayName;
}
/**
* Returns {@code true} if this identifier represents a test.
*/
public boolean isTest() {
return test;
}
/**
* Returns {@code true} if this identifier represents a container.
*/
public boolean isContainer() {
return container;
}
/**
* Get the {@linkplain TestSource source location} of the represented test
* or container, if available.
*
* @see TestSource
*/
public Optional getSource() {
return Optional.ofNullable(source);
}
/**
* Get the set of {@linkplain TestTag tags} of the represented test or
* container.
*
* @see TestTag
*/
public Set getTags() {
return tags;
}
/**
* Get the unique ID of this identifier's parent, if available.
*
* An identifier without a parent ID is called a root.
*/
public Optional getParentId() {
return Optional.ofNullable(parentId);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof TestIdentifier) {
TestIdentifier that = (TestIdentifier) obj;
return Objects.equals(this.uniqueId, that.uniqueId);
}
return false;
}
@Override
public int hashCode() {
return uniqueId.hashCode();
}
@Override
public String toString() {
return getDisplayName() + " [" + uniqueId + "]";
}
}