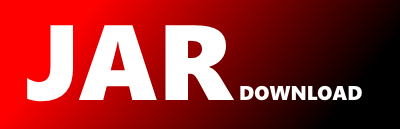
hudson.plugins.analysis.collector.AnalysisResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-collector Show documentation
Show all versions of analysis-collector Show documentation
This plug-in is an add-on for the plug-ins Checkstyle, Dry, FindBugs, PMD, Tasks, and Warnings:
the plug-in collects the different analysis results and shows the results in a combined trend graph.
Additionally, the plug-in provides health reporting and build stability based on these combined results.
The newest version!
package hudson.plugins.analysis.collector; // NOPMD
import hudson.model.AbstractBuild;
import hudson.plugins.analysis.core.BuildHistory;
import hudson.plugins.analysis.core.BuildResult;
import hudson.plugins.analysis.core.ParserResult;
import hudson.plugins.analysis.core.ResultAction;
import hudson.plugins.analysis.util.model.FileAnnotation;
import java.lang.ref.WeakReference;
import java.util.Map;
import com.google.common.collect.Maps;
/**
* Stores the results of the analysis plug-ins. One instance of this class is
* persisted for each build via an XML file.
*
* @author Ulli Hafner
*/
public class AnalysisResult extends BuildResult {
/** Unique identifier of this class. */
private static final long serialVersionUID = 847650789493429154L;
/** Number of annotations by origin mapping. */
private transient WeakReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy