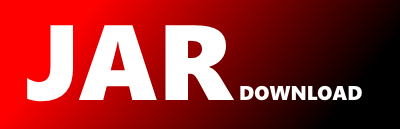
hudson.plugins.sfee.webservice.ArtifactDetail2SoapRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfee Show documentation
Show all versions of sfee Show documentation
This plugin integrates Hudson with a Source Forge Enterprise Edition (SFEE) Server
/**
* ArtifactDetail2SoapRow.java
*
* This file was auto-generated from WSDL
* by the Apache Axis 1.4 Apr 22, 2006 (06:55:48 PDT) WSDL2Java emitter.
*/
package hudson.plugins.sfee.webservice;
public class ArtifactDetail2SoapRow implements java.io.Serializable {
private int actualHours;
private java.lang.String artifactGroup;
private java.lang.String assignedToFullname;
private java.lang.String assignedToUsername;
private java.lang.String category;
private java.util.Calendar closeDate;
private java.lang.String customer;
private java.lang.String description;
private int estimatedHours;
private hudson.plugins.sfee.webservice.SoapFieldValues flexFields;
private java.lang.String folderId;
private java.lang.String folderPathString;
private java.lang.String folderTitle;
private java.lang.String id;
private java.util.Calendar lastModifiedDate;
private int priority;
private java.lang.String projectId;
private java.lang.String projectPathString;
private java.lang.String projectTitle;
private java.lang.String reportedInReleaseTitle;
private java.lang.String resolvedInReleaseTitle;
private java.lang.String status;
private java.lang.String statusClass;
private java.lang.String submittedByFullname;
private java.lang.String submittedByUsername;
private java.util.Calendar submittedDate;
private java.lang.String title;
private int version;
public ArtifactDetail2SoapRow() {
}
public ArtifactDetail2SoapRow(
int actualHours,
java.lang.String artifactGroup,
java.lang.String assignedToFullname,
java.lang.String assignedToUsername,
java.lang.String category,
java.util.Calendar closeDate,
java.lang.String customer,
java.lang.String description,
int estimatedHours,
hudson.plugins.sfee.webservice.SoapFieldValues flexFields,
java.lang.String folderId,
java.lang.String folderPathString,
java.lang.String folderTitle,
java.lang.String id,
java.util.Calendar lastModifiedDate,
int priority,
java.lang.String projectId,
java.lang.String projectPathString,
java.lang.String projectTitle,
java.lang.String reportedInReleaseTitle,
java.lang.String resolvedInReleaseTitle,
java.lang.String status,
java.lang.String statusClass,
java.lang.String submittedByFullname,
java.lang.String submittedByUsername,
java.util.Calendar submittedDate,
java.lang.String title,
int version) {
this.actualHours = actualHours;
this.artifactGroup = artifactGroup;
this.assignedToFullname = assignedToFullname;
this.assignedToUsername = assignedToUsername;
this.category = category;
this.closeDate = closeDate;
this.customer = customer;
this.description = description;
this.estimatedHours = estimatedHours;
this.flexFields = flexFields;
this.folderId = folderId;
this.folderPathString = folderPathString;
this.folderTitle = folderTitle;
this.id = id;
this.lastModifiedDate = lastModifiedDate;
this.priority = priority;
this.projectId = projectId;
this.projectPathString = projectPathString;
this.projectTitle = projectTitle;
this.reportedInReleaseTitle = reportedInReleaseTitle;
this.resolvedInReleaseTitle = resolvedInReleaseTitle;
this.status = status;
this.statusClass = statusClass;
this.submittedByFullname = submittedByFullname;
this.submittedByUsername = submittedByUsername;
this.submittedDate = submittedDate;
this.title = title;
this.version = version;
}
/**
* Gets the actualHours value for this ArtifactDetail2SoapRow.
*
* @return actualHours
*/
public int getActualHours() {
return actualHours;
}
/**
* Sets the actualHours value for this ArtifactDetail2SoapRow.
*
* @param actualHours
*/
public void setActualHours(int actualHours) {
this.actualHours = actualHours;
}
/**
* Gets the artifactGroup value for this ArtifactDetail2SoapRow.
*
* @return artifactGroup
*/
public java.lang.String getArtifactGroup() {
return artifactGroup;
}
/**
* Sets the artifactGroup value for this ArtifactDetail2SoapRow.
*
* @param artifactGroup
*/
public void setArtifactGroup(java.lang.String artifactGroup) {
this.artifactGroup = artifactGroup;
}
/**
* Gets the assignedToFullname value for this ArtifactDetail2SoapRow.
*
* @return assignedToFullname
*/
public java.lang.String getAssignedToFullname() {
return assignedToFullname;
}
/**
* Sets the assignedToFullname value for this ArtifactDetail2SoapRow.
*
* @param assignedToFullname
*/
public void setAssignedToFullname(java.lang.String assignedToFullname) {
this.assignedToFullname = assignedToFullname;
}
/**
* Gets the assignedToUsername value for this ArtifactDetail2SoapRow.
*
* @return assignedToUsername
*/
public java.lang.String getAssignedToUsername() {
return assignedToUsername;
}
/**
* Sets the assignedToUsername value for this ArtifactDetail2SoapRow.
*
* @param assignedToUsername
*/
public void setAssignedToUsername(java.lang.String assignedToUsername) {
this.assignedToUsername = assignedToUsername;
}
/**
* Gets the category value for this ArtifactDetail2SoapRow.
*
* @return category
*/
public java.lang.String getCategory() {
return category;
}
/**
* Sets the category value for this ArtifactDetail2SoapRow.
*
* @param category
*/
public void setCategory(java.lang.String category) {
this.category = category;
}
/**
* Gets the closeDate value for this ArtifactDetail2SoapRow.
*
* @return closeDate
*/
public java.util.Calendar getCloseDate() {
return closeDate;
}
/**
* Sets the closeDate value for this ArtifactDetail2SoapRow.
*
* @param closeDate
*/
public void setCloseDate(java.util.Calendar closeDate) {
this.closeDate = closeDate;
}
/**
* Gets the customer value for this ArtifactDetail2SoapRow.
*
* @return customer
*/
public java.lang.String getCustomer() {
return customer;
}
/**
* Sets the customer value for this ArtifactDetail2SoapRow.
*
* @param customer
*/
public void setCustomer(java.lang.String customer) {
this.customer = customer;
}
/**
* Gets the description value for this ArtifactDetail2SoapRow.
*
* @return description
*/
public java.lang.String getDescription() {
return description;
}
/**
* Sets the description value for this ArtifactDetail2SoapRow.
*
* @param description
*/
public void setDescription(java.lang.String description) {
this.description = description;
}
/**
* Gets the estimatedHours value for this ArtifactDetail2SoapRow.
*
* @return estimatedHours
*/
public int getEstimatedHours() {
return estimatedHours;
}
/**
* Sets the estimatedHours value for this ArtifactDetail2SoapRow.
*
* @param estimatedHours
*/
public void setEstimatedHours(int estimatedHours) {
this.estimatedHours = estimatedHours;
}
/**
* Gets the flexFields value for this ArtifactDetail2SoapRow.
*
* @return flexFields
*/
public hudson.plugins.sfee.webservice.SoapFieldValues getFlexFields() {
return flexFields;
}
/**
* Sets the flexFields value for this ArtifactDetail2SoapRow.
*
* @param flexFields
*/
public void setFlexFields(hudson.plugins.sfee.webservice.SoapFieldValues flexFields) {
this.flexFields = flexFields;
}
/**
* Gets the folderId value for this ArtifactDetail2SoapRow.
*
* @return folderId
*/
public java.lang.String getFolderId() {
return folderId;
}
/**
* Sets the folderId value for this ArtifactDetail2SoapRow.
*
* @param folderId
*/
public void setFolderId(java.lang.String folderId) {
this.folderId = folderId;
}
/**
* Gets the folderPathString value for this ArtifactDetail2SoapRow.
*
* @return folderPathString
*/
public java.lang.String getFolderPathString() {
return folderPathString;
}
/**
* Sets the folderPathString value for this ArtifactDetail2SoapRow.
*
* @param folderPathString
*/
public void setFolderPathString(java.lang.String folderPathString) {
this.folderPathString = folderPathString;
}
/**
* Gets the folderTitle value for this ArtifactDetail2SoapRow.
*
* @return folderTitle
*/
public java.lang.String getFolderTitle() {
return folderTitle;
}
/**
* Sets the folderTitle value for this ArtifactDetail2SoapRow.
*
* @param folderTitle
*/
public void setFolderTitle(java.lang.String folderTitle) {
this.folderTitle = folderTitle;
}
/**
* Gets the id value for this ArtifactDetail2SoapRow.
*
* @return id
*/
public java.lang.String getId() {
return id;
}
/**
* Sets the id value for this ArtifactDetail2SoapRow.
*
* @param id
*/
public void setId(java.lang.String id) {
this.id = id;
}
/**
* Gets the lastModifiedDate value for this ArtifactDetail2SoapRow.
*
* @return lastModifiedDate
*/
public java.util.Calendar getLastModifiedDate() {
return lastModifiedDate;
}
/**
* Sets the lastModifiedDate value for this ArtifactDetail2SoapRow.
*
* @param lastModifiedDate
*/
public void setLastModifiedDate(java.util.Calendar lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
* Gets the priority value for this ArtifactDetail2SoapRow.
*
* @return priority
*/
public int getPriority() {
return priority;
}
/**
* Sets the priority value for this ArtifactDetail2SoapRow.
*
* @param priority
*/
public void setPriority(int priority) {
this.priority = priority;
}
/**
* Gets the projectId value for this ArtifactDetail2SoapRow.
*
* @return projectId
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* Sets the projectId value for this ArtifactDetail2SoapRow.
*
* @param projectId
*/
public void setProjectId(java.lang.String projectId) {
this.projectId = projectId;
}
/**
* Gets the projectPathString value for this ArtifactDetail2SoapRow.
*
* @return projectPathString
*/
public java.lang.String getProjectPathString() {
return projectPathString;
}
/**
* Sets the projectPathString value for this ArtifactDetail2SoapRow.
*
* @param projectPathString
*/
public void setProjectPathString(java.lang.String projectPathString) {
this.projectPathString = projectPathString;
}
/**
* Gets the projectTitle value for this ArtifactDetail2SoapRow.
*
* @return projectTitle
*/
public java.lang.String getProjectTitle() {
return projectTitle;
}
/**
* Sets the projectTitle value for this ArtifactDetail2SoapRow.
*
* @param projectTitle
*/
public void setProjectTitle(java.lang.String projectTitle) {
this.projectTitle = projectTitle;
}
/**
* Gets the reportedInReleaseTitle value for this ArtifactDetail2SoapRow.
*
* @return reportedInReleaseTitle
*/
public java.lang.String getReportedInReleaseTitle() {
return reportedInReleaseTitle;
}
/**
* Sets the reportedInReleaseTitle value for this ArtifactDetail2SoapRow.
*
* @param reportedInReleaseTitle
*/
public void setReportedInReleaseTitle(java.lang.String reportedInReleaseTitle) {
this.reportedInReleaseTitle = reportedInReleaseTitle;
}
/**
* Gets the resolvedInReleaseTitle value for this ArtifactDetail2SoapRow.
*
* @return resolvedInReleaseTitle
*/
public java.lang.String getResolvedInReleaseTitle() {
return resolvedInReleaseTitle;
}
/**
* Sets the resolvedInReleaseTitle value for this ArtifactDetail2SoapRow.
*
* @param resolvedInReleaseTitle
*/
public void setResolvedInReleaseTitle(java.lang.String resolvedInReleaseTitle) {
this.resolvedInReleaseTitle = resolvedInReleaseTitle;
}
/**
* Gets the status value for this ArtifactDetail2SoapRow.
*
* @return status
*/
public java.lang.String getStatus() {
return status;
}
/**
* Sets the status value for this ArtifactDetail2SoapRow.
*
* @param status
*/
public void setStatus(java.lang.String status) {
this.status = status;
}
/**
* Gets the statusClass value for this ArtifactDetail2SoapRow.
*
* @return statusClass
*/
public java.lang.String getStatusClass() {
return statusClass;
}
/**
* Sets the statusClass value for this ArtifactDetail2SoapRow.
*
* @param statusClass
*/
public void setStatusClass(java.lang.String statusClass) {
this.statusClass = statusClass;
}
/**
* Gets the submittedByFullname value for this ArtifactDetail2SoapRow.
*
* @return submittedByFullname
*/
public java.lang.String getSubmittedByFullname() {
return submittedByFullname;
}
/**
* Sets the submittedByFullname value for this ArtifactDetail2SoapRow.
*
* @param submittedByFullname
*/
public void setSubmittedByFullname(java.lang.String submittedByFullname) {
this.submittedByFullname = submittedByFullname;
}
/**
* Gets the submittedByUsername value for this ArtifactDetail2SoapRow.
*
* @return submittedByUsername
*/
public java.lang.String getSubmittedByUsername() {
return submittedByUsername;
}
/**
* Sets the submittedByUsername value for this ArtifactDetail2SoapRow.
*
* @param submittedByUsername
*/
public void setSubmittedByUsername(java.lang.String submittedByUsername) {
this.submittedByUsername = submittedByUsername;
}
/**
* Gets the submittedDate value for this ArtifactDetail2SoapRow.
*
* @return submittedDate
*/
public java.util.Calendar getSubmittedDate() {
return submittedDate;
}
/**
* Sets the submittedDate value for this ArtifactDetail2SoapRow.
*
* @param submittedDate
*/
public void setSubmittedDate(java.util.Calendar submittedDate) {
this.submittedDate = submittedDate;
}
/**
* Gets the title value for this ArtifactDetail2SoapRow.
*
* @return title
*/
public java.lang.String getTitle() {
return title;
}
/**
* Sets the title value for this ArtifactDetail2SoapRow.
*
* @param title
*/
public void setTitle(java.lang.String title) {
this.title = title;
}
/**
* Gets the version value for this ArtifactDetail2SoapRow.
*
* @return version
*/
public int getVersion() {
return version;
}
/**
* Sets the version value for this ArtifactDetail2SoapRow.
*
* @param version
*/
public void setVersion(int version) {
this.version = version;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof ArtifactDetail2SoapRow)) return false;
ArtifactDetail2SoapRow other = (ArtifactDetail2SoapRow) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
this.actualHours == other.getActualHours() &&
((this.artifactGroup==null && other.getArtifactGroup()==null) ||
(this.artifactGroup!=null &&
this.artifactGroup.equals(other.getArtifactGroup()))) &&
((this.assignedToFullname==null && other.getAssignedToFullname()==null) ||
(this.assignedToFullname!=null &&
this.assignedToFullname.equals(other.getAssignedToFullname()))) &&
((this.assignedToUsername==null && other.getAssignedToUsername()==null) ||
(this.assignedToUsername!=null &&
this.assignedToUsername.equals(other.getAssignedToUsername()))) &&
((this.category==null && other.getCategory()==null) ||
(this.category!=null &&
this.category.equals(other.getCategory()))) &&
((this.closeDate==null && other.getCloseDate()==null) ||
(this.closeDate!=null &&
this.closeDate.equals(other.getCloseDate()))) &&
((this.customer==null && other.getCustomer()==null) ||
(this.customer!=null &&
this.customer.equals(other.getCustomer()))) &&
((this.description==null && other.getDescription()==null) ||
(this.description!=null &&
this.description.equals(other.getDescription()))) &&
this.estimatedHours == other.getEstimatedHours() &&
((this.flexFields==null && other.getFlexFields()==null) ||
(this.flexFields!=null &&
this.flexFields.equals(other.getFlexFields()))) &&
((this.folderId==null && other.getFolderId()==null) ||
(this.folderId!=null &&
this.folderId.equals(other.getFolderId()))) &&
((this.folderPathString==null && other.getFolderPathString()==null) ||
(this.folderPathString!=null &&
this.folderPathString.equals(other.getFolderPathString()))) &&
((this.folderTitle==null && other.getFolderTitle()==null) ||
(this.folderTitle!=null &&
this.folderTitle.equals(other.getFolderTitle()))) &&
((this.id==null && other.getId()==null) ||
(this.id!=null &&
this.id.equals(other.getId()))) &&
((this.lastModifiedDate==null && other.getLastModifiedDate()==null) ||
(this.lastModifiedDate!=null &&
this.lastModifiedDate.equals(other.getLastModifiedDate()))) &&
this.priority == other.getPriority() &&
((this.projectId==null && other.getProjectId()==null) ||
(this.projectId!=null &&
this.projectId.equals(other.getProjectId()))) &&
((this.projectPathString==null && other.getProjectPathString()==null) ||
(this.projectPathString!=null &&
this.projectPathString.equals(other.getProjectPathString()))) &&
((this.projectTitle==null && other.getProjectTitle()==null) ||
(this.projectTitle!=null &&
this.projectTitle.equals(other.getProjectTitle()))) &&
((this.reportedInReleaseTitle==null && other.getReportedInReleaseTitle()==null) ||
(this.reportedInReleaseTitle!=null &&
this.reportedInReleaseTitle.equals(other.getReportedInReleaseTitle()))) &&
((this.resolvedInReleaseTitle==null && other.getResolvedInReleaseTitle()==null) ||
(this.resolvedInReleaseTitle!=null &&
this.resolvedInReleaseTitle.equals(other.getResolvedInReleaseTitle()))) &&
((this.status==null && other.getStatus()==null) ||
(this.status!=null &&
this.status.equals(other.getStatus()))) &&
((this.statusClass==null && other.getStatusClass()==null) ||
(this.statusClass!=null &&
this.statusClass.equals(other.getStatusClass()))) &&
((this.submittedByFullname==null && other.getSubmittedByFullname()==null) ||
(this.submittedByFullname!=null &&
this.submittedByFullname.equals(other.getSubmittedByFullname()))) &&
((this.submittedByUsername==null && other.getSubmittedByUsername()==null) ||
(this.submittedByUsername!=null &&
this.submittedByUsername.equals(other.getSubmittedByUsername()))) &&
((this.submittedDate==null && other.getSubmittedDate()==null) ||
(this.submittedDate!=null &&
this.submittedDate.equals(other.getSubmittedDate()))) &&
((this.title==null && other.getTitle()==null) ||
(this.title!=null &&
this.title.equals(other.getTitle()))) &&
this.version == other.getVersion();
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
_hashCode += getActualHours();
if (getArtifactGroup() != null) {
_hashCode += getArtifactGroup().hashCode();
}
if (getAssignedToFullname() != null) {
_hashCode += getAssignedToFullname().hashCode();
}
if (getAssignedToUsername() != null) {
_hashCode += getAssignedToUsername().hashCode();
}
if (getCategory() != null) {
_hashCode += getCategory().hashCode();
}
if (getCloseDate() != null) {
_hashCode += getCloseDate().hashCode();
}
if (getCustomer() != null) {
_hashCode += getCustomer().hashCode();
}
if (getDescription() != null) {
_hashCode += getDescription().hashCode();
}
_hashCode += getEstimatedHours();
if (getFlexFields() != null) {
_hashCode += getFlexFields().hashCode();
}
if (getFolderId() != null) {
_hashCode += getFolderId().hashCode();
}
if (getFolderPathString() != null) {
_hashCode += getFolderPathString().hashCode();
}
if (getFolderTitle() != null) {
_hashCode += getFolderTitle().hashCode();
}
if (getId() != null) {
_hashCode += getId().hashCode();
}
if (getLastModifiedDate() != null) {
_hashCode += getLastModifiedDate().hashCode();
}
_hashCode += getPriority();
if (getProjectId() != null) {
_hashCode += getProjectId().hashCode();
}
if (getProjectPathString() != null) {
_hashCode += getProjectPathString().hashCode();
}
if (getProjectTitle() != null) {
_hashCode += getProjectTitle().hashCode();
}
if (getReportedInReleaseTitle() != null) {
_hashCode += getReportedInReleaseTitle().hashCode();
}
if (getResolvedInReleaseTitle() != null) {
_hashCode += getResolvedInReleaseTitle().hashCode();
}
if (getStatus() != null) {
_hashCode += getStatus().hashCode();
}
if (getStatusClass() != null) {
_hashCode += getStatusClass().hashCode();
}
if (getSubmittedByFullname() != null) {
_hashCode += getSubmittedByFullname().hashCode();
}
if (getSubmittedByUsername() != null) {
_hashCode += getSubmittedByUsername().hashCode();
}
if (getSubmittedDate() != null) {
_hashCode += getSubmittedDate().hashCode();
}
if (getTitle() != null) {
_hashCode += getTitle().hashCode();
}
_hashCode += getVersion();
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(ArtifactDetail2SoapRow.class, true);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://schema.vasoftware.com/sf/soap43/type", "ArtifactDetail2SoapRow"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("actualHours");
elemField.setXmlName(new javax.xml.namespace.QName("", "actualHours"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("artifactGroup");
elemField.setXmlName(new javax.xml.namespace.QName("", "artifactGroup"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("assignedToFullname");
elemField.setXmlName(new javax.xml.namespace.QName("", "assignedToFullname"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("assignedToUsername");
elemField.setXmlName(new javax.xml.namespace.QName("", "assignedToUsername"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("category");
elemField.setXmlName(new javax.xml.namespace.QName("", "category"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("closeDate");
elemField.setXmlName(new javax.xml.namespace.QName("", "closeDate"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("customer");
elemField.setXmlName(new javax.xml.namespace.QName("", "customer"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("description");
elemField.setXmlName(new javax.xml.namespace.QName("", "description"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("estimatedHours");
elemField.setXmlName(new javax.xml.namespace.QName("", "estimatedHours"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("flexFields");
elemField.setXmlName(new javax.xml.namespace.QName("", "flexFields"));
elemField.setXmlType(new javax.xml.namespace.QName("http://schema.vasoftware.com/sf/soap43/type", "SoapFieldValues"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderId");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderId"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderPathString");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderPathString"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("id");
elemField.setXmlName(new javax.xml.namespace.QName("", "id"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("lastModifiedDate");
elemField.setXmlName(new javax.xml.namespace.QName("", "lastModifiedDate"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("priority");
elemField.setXmlName(new javax.xml.namespace.QName("", "priority"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectId");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectId"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectPathString");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectPathString"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("reportedInReleaseTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "reportedInReleaseTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("resolvedInReleaseTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "resolvedInReleaseTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("status");
elemField.setXmlName(new javax.xml.namespace.QName("", "status"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("statusClass");
elemField.setXmlName(new javax.xml.namespace.QName("", "statusClass"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("submittedByFullname");
elemField.setXmlName(new javax.xml.namespace.QName("", "submittedByFullname"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("submittedByUsername");
elemField.setXmlName(new javax.xml.namespace.QName("", "submittedByUsername"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("submittedDate");
elemField.setXmlName(new javax.xml.namespace.QName("", "submittedDate"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("title");
elemField.setXmlName(new javax.xml.namespace.QName("", "title"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("version");
elemField.setXmlName(new javax.xml.namespace.QName("", "version"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy