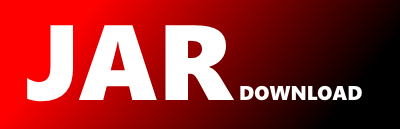
hudson.plugins.sfee.webservice.DocumentSoapRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfee Show documentation
Show all versions of sfee Show documentation
This plugin integrates Hudson with a Source Forge Enterprise Edition (SFEE) Server
/**
* DocumentSoapRow.java
*
* This file was auto-generated from WSDL
* by the Apache Axis 1.4 Apr 22, 2006 (06:55:48 PDT) WSDL2Java emitter.
*/
package hudson.plugins.sfee.webservice;
public class DocumentSoapRow implements java.io.Serializable {
private java.lang.String createdBy;
private int currentVersion;
private java.util.Calendar dateCreated;
private java.util.Calendar dateLastModified;
private java.util.Calendar dateVersionCreated;
private java.lang.String description;
private java.lang.String fileName;
private long fileSize;
private java.lang.String folderId;
private java.lang.String folderPathString;
private java.lang.String folderTitle;
private java.lang.String id;
private java.lang.String lastModifiedBy;
private java.lang.String lastModifiedByFullName;
private java.lang.String mimeType;
private java.lang.String projectId;
private java.lang.String projectPathString;
private java.lang.String projectTitle;
private java.lang.String title;
private java.lang.String versionComment;
private java.lang.String versionCreatedBy;
private java.lang.String versionCreatedByFullname;
private java.lang.String versionId;
private int versionNumber;
private java.lang.String versionStatus;
public DocumentSoapRow() {
}
public DocumentSoapRow(
java.lang.String createdBy,
int currentVersion,
java.util.Calendar dateCreated,
java.util.Calendar dateLastModified,
java.util.Calendar dateVersionCreated,
java.lang.String description,
java.lang.String fileName,
long fileSize,
java.lang.String folderId,
java.lang.String folderPathString,
java.lang.String folderTitle,
java.lang.String id,
java.lang.String lastModifiedBy,
java.lang.String lastModifiedByFullName,
java.lang.String mimeType,
java.lang.String projectId,
java.lang.String projectPathString,
java.lang.String projectTitle,
java.lang.String title,
java.lang.String versionComment,
java.lang.String versionCreatedBy,
java.lang.String versionCreatedByFullname,
java.lang.String versionId,
int versionNumber,
java.lang.String versionStatus) {
this.createdBy = createdBy;
this.currentVersion = currentVersion;
this.dateCreated = dateCreated;
this.dateLastModified = dateLastModified;
this.dateVersionCreated = dateVersionCreated;
this.description = description;
this.fileName = fileName;
this.fileSize = fileSize;
this.folderId = folderId;
this.folderPathString = folderPathString;
this.folderTitle = folderTitle;
this.id = id;
this.lastModifiedBy = lastModifiedBy;
this.lastModifiedByFullName = lastModifiedByFullName;
this.mimeType = mimeType;
this.projectId = projectId;
this.projectPathString = projectPathString;
this.projectTitle = projectTitle;
this.title = title;
this.versionComment = versionComment;
this.versionCreatedBy = versionCreatedBy;
this.versionCreatedByFullname = versionCreatedByFullname;
this.versionId = versionId;
this.versionNumber = versionNumber;
this.versionStatus = versionStatus;
}
/**
* Gets the createdBy value for this DocumentSoapRow.
*
* @return createdBy
*/
public java.lang.String getCreatedBy() {
return createdBy;
}
/**
* Sets the createdBy value for this DocumentSoapRow.
*
* @param createdBy
*/
public void setCreatedBy(java.lang.String createdBy) {
this.createdBy = createdBy;
}
/**
* Gets the currentVersion value for this DocumentSoapRow.
*
* @return currentVersion
*/
public int getCurrentVersion() {
return currentVersion;
}
/**
* Sets the currentVersion value for this DocumentSoapRow.
*
* @param currentVersion
*/
public void setCurrentVersion(int currentVersion) {
this.currentVersion = currentVersion;
}
/**
* Gets the dateCreated value for this DocumentSoapRow.
*
* @return dateCreated
*/
public java.util.Calendar getDateCreated() {
return dateCreated;
}
/**
* Sets the dateCreated value for this DocumentSoapRow.
*
* @param dateCreated
*/
public void setDateCreated(java.util.Calendar dateCreated) {
this.dateCreated = dateCreated;
}
/**
* Gets the dateLastModified value for this DocumentSoapRow.
*
* @return dateLastModified
*/
public java.util.Calendar getDateLastModified() {
return dateLastModified;
}
/**
* Sets the dateLastModified value for this DocumentSoapRow.
*
* @param dateLastModified
*/
public void setDateLastModified(java.util.Calendar dateLastModified) {
this.dateLastModified = dateLastModified;
}
/**
* Gets the dateVersionCreated value for this DocumentSoapRow.
*
* @return dateVersionCreated
*/
public java.util.Calendar getDateVersionCreated() {
return dateVersionCreated;
}
/**
* Sets the dateVersionCreated value for this DocumentSoapRow.
*
* @param dateVersionCreated
*/
public void setDateVersionCreated(java.util.Calendar dateVersionCreated) {
this.dateVersionCreated = dateVersionCreated;
}
/**
* Gets the description value for this DocumentSoapRow.
*
* @return description
*/
public java.lang.String getDescription() {
return description;
}
/**
* Sets the description value for this DocumentSoapRow.
*
* @param description
*/
public void setDescription(java.lang.String description) {
this.description = description;
}
/**
* Gets the fileName value for this DocumentSoapRow.
*
* @return fileName
*/
public java.lang.String getFileName() {
return fileName;
}
/**
* Sets the fileName value for this DocumentSoapRow.
*
* @param fileName
*/
public void setFileName(java.lang.String fileName) {
this.fileName = fileName;
}
/**
* Gets the fileSize value for this DocumentSoapRow.
*
* @return fileSize
*/
public long getFileSize() {
return fileSize;
}
/**
* Sets the fileSize value for this DocumentSoapRow.
*
* @param fileSize
*/
public void setFileSize(long fileSize) {
this.fileSize = fileSize;
}
/**
* Gets the folderId value for this DocumentSoapRow.
*
* @return folderId
*/
public java.lang.String getFolderId() {
return folderId;
}
/**
* Sets the folderId value for this DocumentSoapRow.
*
* @param folderId
*/
public void setFolderId(java.lang.String folderId) {
this.folderId = folderId;
}
/**
* Gets the folderPathString value for this DocumentSoapRow.
*
* @return folderPathString
*/
public java.lang.String getFolderPathString() {
return folderPathString;
}
/**
* Sets the folderPathString value for this DocumentSoapRow.
*
* @param folderPathString
*/
public void setFolderPathString(java.lang.String folderPathString) {
this.folderPathString = folderPathString;
}
/**
* Gets the folderTitle value for this DocumentSoapRow.
*
* @return folderTitle
*/
public java.lang.String getFolderTitle() {
return folderTitle;
}
/**
* Sets the folderTitle value for this DocumentSoapRow.
*
* @param folderTitle
*/
public void setFolderTitle(java.lang.String folderTitle) {
this.folderTitle = folderTitle;
}
/**
* Gets the id value for this DocumentSoapRow.
*
* @return id
*/
public java.lang.String getId() {
return id;
}
/**
* Sets the id value for this DocumentSoapRow.
*
* @param id
*/
public void setId(java.lang.String id) {
this.id = id;
}
/**
* Gets the lastModifiedBy value for this DocumentSoapRow.
*
* @return lastModifiedBy
*/
public java.lang.String getLastModifiedBy() {
return lastModifiedBy;
}
/**
* Sets the lastModifiedBy value for this DocumentSoapRow.
*
* @param lastModifiedBy
*/
public void setLastModifiedBy(java.lang.String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
}
/**
* Gets the lastModifiedByFullName value for this DocumentSoapRow.
*
* @return lastModifiedByFullName
*/
public java.lang.String getLastModifiedByFullName() {
return lastModifiedByFullName;
}
/**
* Sets the lastModifiedByFullName value for this DocumentSoapRow.
*
* @param lastModifiedByFullName
*/
public void setLastModifiedByFullName(java.lang.String lastModifiedByFullName) {
this.lastModifiedByFullName = lastModifiedByFullName;
}
/**
* Gets the mimeType value for this DocumentSoapRow.
*
* @return mimeType
*/
public java.lang.String getMimeType() {
return mimeType;
}
/**
* Sets the mimeType value for this DocumentSoapRow.
*
* @param mimeType
*/
public void setMimeType(java.lang.String mimeType) {
this.mimeType = mimeType;
}
/**
* Gets the projectId value for this DocumentSoapRow.
*
* @return projectId
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* Sets the projectId value for this DocumentSoapRow.
*
* @param projectId
*/
public void setProjectId(java.lang.String projectId) {
this.projectId = projectId;
}
/**
* Gets the projectPathString value for this DocumentSoapRow.
*
* @return projectPathString
*/
public java.lang.String getProjectPathString() {
return projectPathString;
}
/**
* Sets the projectPathString value for this DocumentSoapRow.
*
* @param projectPathString
*/
public void setProjectPathString(java.lang.String projectPathString) {
this.projectPathString = projectPathString;
}
/**
* Gets the projectTitle value for this DocumentSoapRow.
*
* @return projectTitle
*/
public java.lang.String getProjectTitle() {
return projectTitle;
}
/**
* Sets the projectTitle value for this DocumentSoapRow.
*
* @param projectTitle
*/
public void setProjectTitle(java.lang.String projectTitle) {
this.projectTitle = projectTitle;
}
/**
* Gets the title value for this DocumentSoapRow.
*
* @return title
*/
public java.lang.String getTitle() {
return title;
}
/**
* Sets the title value for this DocumentSoapRow.
*
* @param title
*/
public void setTitle(java.lang.String title) {
this.title = title;
}
/**
* Gets the versionComment value for this DocumentSoapRow.
*
* @return versionComment
*/
public java.lang.String getVersionComment() {
return versionComment;
}
/**
* Sets the versionComment value for this DocumentSoapRow.
*
* @param versionComment
*/
public void setVersionComment(java.lang.String versionComment) {
this.versionComment = versionComment;
}
/**
* Gets the versionCreatedBy value for this DocumentSoapRow.
*
* @return versionCreatedBy
*/
public java.lang.String getVersionCreatedBy() {
return versionCreatedBy;
}
/**
* Sets the versionCreatedBy value for this DocumentSoapRow.
*
* @param versionCreatedBy
*/
public void setVersionCreatedBy(java.lang.String versionCreatedBy) {
this.versionCreatedBy = versionCreatedBy;
}
/**
* Gets the versionCreatedByFullname value for this DocumentSoapRow.
*
* @return versionCreatedByFullname
*/
public java.lang.String getVersionCreatedByFullname() {
return versionCreatedByFullname;
}
/**
* Sets the versionCreatedByFullname value for this DocumentSoapRow.
*
* @param versionCreatedByFullname
*/
public void setVersionCreatedByFullname(java.lang.String versionCreatedByFullname) {
this.versionCreatedByFullname = versionCreatedByFullname;
}
/**
* Gets the versionId value for this DocumentSoapRow.
*
* @return versionId
*/
public java.lang.String getVersionId() {
return versionId;
}
/**
* Sets the versionId value for this DocumentSoapRow.
*
* @param versionId
*/
public void setVersionId(java.lang.String versionId) {
this.versionId = versionId;
}
/**
* Gets the versionNumber value for this DocumentSoapRow.
*
* @return versionNumber
*/
public int getVersionNumber() {
return versionNumber;
}
/**
* Sets the versionNumber value for this DocumentSoapRow.
*
* @param versionNumber
*/
public void setVersionNumber(int versionNumber) {
this.versionNumber = versionNumber;
}
/**
* Gets the versionStatus value for this DocumentSoapRow.
*
* @return versionStatus
*/
public java.lang.String getVersionStatus() {
return versionStatus;
}
/**
* Sets the versionStatus value for this DocumentSoapRow.
*
* @param versionStatus
*/
public void setVersionStatus(java.lang.String versionStatus) {
this.versionStatus = versionStatus;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof DocumentSoapRow)) return false;
DocumentSoapRow other = (DocumentSoapRow) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.createdBy==null && other.getCreatedBy()==null) ||
(this.createdBy!=null &&
this.createdBy.equals(other.getCreatedBy()))) &&
this.currentVersion == other.getCurrentVersion() &&
((this.dateCreated==null && other.getDateCreated()==null) ||
(this.dateCreated!=null &&
this.dateCreated.equals(other.getDateCreated()))) &&
((this.dateLastModified==null && other.getDateLastModified()==null) ||
(this.dateLastModified!=null &&
this.dateLastModified.equals(other.getDateLastModified()))) &&
((this.dateVersionCreated==null && other.getDateVersionCreated()==null) ||
(this.dateVersionCreated!=null &&
this.dateVersionCreated.equals(other.getDateVersionCreated()))) &&
((this.description==null && other.getDescription()==null) ||
(this.description!=null &&
this.description.equals(other.getDescription()))) &&
((this.fileName==null && other.getFileName()==null) ||
(this.fileName!=null &&
this.fileName.equals(other.getFileName()))) &&
this.fileSize == other.getFileSize() &&
((this.folderId==null && other.getFolderId()==null) ||
(this.folderId!=null &&
this.folderId.equals(other.getFolderId()))) &&
((this.folderPathString==null && other.getFolderPathString()==null) ||
(this.folderPathString!=null &&
this.folderPathString.equals(other.getFolderPathString()))) &&
((this.folderTitle==null && other.getFolderTitle()==null) ||
(this.folderTitle!=null &&
this.folderTitle.equals(other.getFolderTitle()))) &&
((this.id==null && other.getId()==null) ||
(this.id!=null &&
this.id.equals(other.getId()))) &&
((this.lastModifiedBy==null && other.getLastModifiedBy()==null) ||
(this.lastModifiedBy!=null &&
this.lastModifiedBy.equals(other.getLastModifiedBy()))) &&
((this.lastModifiedByFullName==null && other.getLastModifiedByFullName()==null) ||
(this.lastModifiedByFullName!=null &&
this.lastModifiedByFullName.equals(other.getLastModifiedByFullName()))) &&
((this.mimeType==null && other.getMimeType()==null) ||
(this.mimeType!=null &&
this.mimeType.equals(other.getMimeType()))) &&
((this.projectId==null && other.getProjectId()==null) ||
(this.projectId!=null &&
this.projectId.equals(other.getProjectId()))) &&
((this.projectPathString==null && other.getProjectPathString()==null) ||
(this.projectPathString!=null &&
this.projectPathString.equals(other.getProjectPathString()))) &&
((this.projectTitle==null && other.getProjectTitle()==null) ||
(this.projectTitle!=null &&
this.projectTitle.equals(other.getProjectTitle()))) &&
((this.title==null && other.getTitle()==null) ||
(this.title!=null &&
this.title.equals(other.getTitle()))) &&
((this.versionComment==null && other.getVersionComment()==null) ||
(this.versionComment!=null &&
this.versionComment.equals(other.getVersionComment()))) &&
((this.versionCreatedBy==null && other.getVersionCreatedBy()==null) ||
(this.versionCreatedBy!=null &&
this.versionCreatedBy.equals(other.getVersionCreatedBy()))) &&
((this.versionCreatedByFullname==null && other.getVersionCreatedByFullname()==null) ||
(this.versionCreatedByFullname!=null &&
this.versionCreatedByFullname.equals(other.getVersionCreatedByFullname()))) &&
((this.versionId==null && other.getVersionId()==null) ||
(this.versionId!=null &&
this.versionId.equals(other.getVersionId()))) &&
this.versionNumber == other.getVersionNumber() &&
((this.versionStatus==null && other.getVersionStatus()==null) ||
(this.versionStatus!=null &&
this.versionStatus.equals(other.getVersionStatus())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getCreatedBy() != null) {
_hashCode += getCreatedBy().hashCode();
}
_hashCode += getCurrentVersion();
if (getDateCreated() != null) {
_hashCode += getDateCreated().hashCode();
}
if (getDateLastModified() != null) {
_hashCode += getDateLastModified().hashCode();
}
if (getDateVersionCreated() != null) {
_hashCode += getDateVersionCreated().hashCode();
}
if (getDescription() != null) {
_hashCode += getDescription().hashCode();
}
if (getFileName() != null) {
_hashCode += getFileName().hashCode();
}
_hashCode += new Long(getFileSize()).hashCode();
if (getFolderId() != null) {
_hashCode += getFolderId().hashCode();
}
if (getFolderPathString() != null) {
_hashCode += getFolderPathString().hashCode();
}
if (getFolderTitle() != null) {
_hashCode += getFolderTitle().hashCode();
}
if (getId() != null) {
_hashCode += getId().hashCode();
}
if (getLastModifiedBy() != null) {
_hashCode += getLastModifiedBy().hashCode();
}
if (getLastModifiedByFullName() != null) {
_hashCode += getLastModifiedByFullName().hashCode();
}
if (getMimeType() != null) {
_hashCode += getMimeType().hashCode();
}
if (getProjectId() != null) {
_hashCode += getProjectId().hashCode();
}
if (getProjectPathString() != null) {
_hashCode += getProjectPathString().hashCode();
}
if (getProjectTitle() != null) {
_hashCode += getProjectTitle().hashCode();
}
if (getTitle() != null) {
_hashCode += getTitle().hashCode();
}
if (getVersionComment() != null) {
_hashCode += getVersionComment().hashCode();
}
if (getVersionCreatedBy() != null) {
_hashCode += getVersionCreatedBy().hashCode();
}
if (getVersionCreatedByFullname() != null) {
_hashCode += getVersionCreatedByFullname().hashCode();
}
if (getVersionId() != null) {
_hashCode += getVersionId().hashCode();
}
_hashCode += getVersionNumber();
if (getVersionStatus() != null) {
_hashCode += getVersionStatus().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(DocumentSoapRow.class, true);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://schema.vasoftware.com/sf/soap43/type", "DocumentSoapRow"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("createdBy");
elemField.setXmlName(new javax.xml.namespace.QName("", "createdBy"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("currentVersion");
elemField.setXmlName(new javax.xml.namespace.QName("", "currentVersion"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("dateCreated");
elemField.setXmlName(new javax.xml.namespace.QName("", "dateCreated"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("dateLastModified");
elemField.setXmlName(new javax.xml.namespace.QName("", "dateLastModified"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("dateVersionCreated");
elemField.setXmlName(new javax.xml.namespace.QName("", "dateVersionCreated"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("description");
elemField.setXmlName(new javax.xml.namespace.QName("", "description"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("fileName");
elemField.setXmlName(new javax.xml.namespace.QName("", "fileName"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("fileSize");
elemField.setXmlName(new javax.xml.namespace.QName("", "fileSize"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "long"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderId");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderId"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderPathString");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderPathString"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("folderTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "folderTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("id");
elemField.setXmlName(new javax.xml.namespace.QName("", "id"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("lastModifiedBy");
elemField.setXmlName(new javax.xml.namespace.QName("", "lastModifiedBy"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("lastModifiedByFullName");
elemField.setXmlName(new javax.xml.namespace.QName("", "lastModifiedByFullName"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("mimeType");
elemField.setXmlName(new javax.xml.namespace.QName("", "mimeType"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectId");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectId"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectPathString");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectPathString"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("projectTitle");
elemField.setXmlName(new javax.xml.namespace.QName("", "projectTitle"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("title");
elemField.setXmlName(new javax.xml.namespace.QName("", "title"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionComment");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionComment"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionCreatedBy");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionCreatedBy"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionCreatedByFullname");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionCreatedByFullname"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionId");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionId"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionNumber");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionNumber"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "int"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("versionStatus");
elemField.setXmlName(new javax.xml.namespace.QName("", "versionStatus"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy