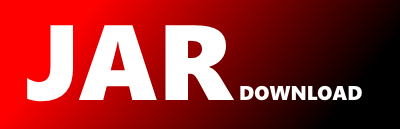
hudson.plugins.sfee.webservice.UserSoapDO Maven / Gradle / Ivy
/**
* UserSoapDO.java
*
* This file was auto-generated from WSDL
* by the Apache Axis 1.4 Apr 22, 2006 (06:55:48 PDT) WSDL2Java emitter.
*/
package hudson.plugins.sfee.webservice;
public class UserSoapDO extends hudson.plugins.sfee.webservice.ObjectSoapDO implements java.io.Serializable {
private java.lang.String email;
private java.lang.String fullName;
private java.lang.String locale;
private boolean restrictedUser;
private java.lang.String status;
private boolean superUser;
private java.lang.String timeZone;
private java.lang.String username;
public UserSoapDO() {
}
public UserSoapDO(
java.lang.String createdBy,
java.util.Calendar createdDate,
java.lang.String id,
java.lang.String lastModifiedBy,
java.util.Calendar lastModifiedDate,
int version,
java.lang.String email,
java.lang.String fullName,
java.lang.String locale,
boolean restrictedUser,
java.lang.String status,
boolean superUser,
java.lang.String timeZone,
java.lang.String username) {
super(
createdBy,
createdDate,
id,
lastModifiedBy,
lastModifiedDate,
version);
this.email = email;
this.fullName = fullName;
this.locale = locale;
this.restrictedUser = restrictedUser;
this.status = status;
this.superUser = superUser;
this.timeZone = timeZone;
this.username = username;
}
/**
* Gets the email value for this UserSoapDO.
*
* @return email
*/
public java.lang.String getEmail() {
return email;
}
/**
* Sets the email value for this UserSoapDO.
*
* @param email
*/
public void setEmail(java.lang.String email) {
this.email = email;
}
/**
* Gets the fullName value for this UserSoapDO.
*
* @return fullName
*/
public java.lang.String getFullName() {
return fullName;
}
/**
* Sets the fullName value for this UserSoapDO.
*
* @param fullName
*/
public void setFullName(java.lang.String fullName) {
this.fullName = fullName;
}
/**
* Gets the locale value for this UserSoapDO.
*
* @return locale
*/
public java.lang.String getLocale() {
return locale;
}
/**
* Sets the locale value for this UserSoapDO.
*
* @param locale
*/
public void setLocale(java.lang.String locale) {
this.locale = locale;
}
/**
* Gets the restrictedUser value for this UserSoapDO.
*
* @return restrictedUser
*/
public boolean isRestrictedUser() {
return restrictedUser;
}
/**
* Sets the restrictedUser value for this UserSoapDO.
*
* @param restrictedUser
*/
public void setRestrictedUser(boolean restrictedUser) {
this.restrictedUser = restrictedUser;
}
/**
* Gets the status value for this UserSoapDO.
*
* @return status
*/
public java.lang.String getStatus() {
return status;
}
/**
* Sets the status value for this UserSoapDO.
*
* @param status
*/
public void setStatus(java.lang.String status) {
this.status = status;
}
/**
* Gets the superUser value for this UserSoapDO.
*
* @return superUser
*/
public boolean isSuperUser() {
return superUser;
}
/**
* Sets the superUser value for this UserSoapDO.
*
* @param superUser
*/
public void setSuperUser(boolean superUser) {
this.superUser = superUser;
}
/**
* Gets the timeZone value for this UserSoapDO.
*
* @return timeZone
*/
public java.lang.String getTimeZone() {
return timeZone;
}
/**
* Sets the timeZone value for this UserSoapDO.
*
* @param timeZone
*/
public void setTimeZone(java.lang.String timeZone) {
this.timeZone = timeZone;
}
/**
* Gets the username value for this UserSoapDO.
*
* @return username
*/
public java.lang.String getUsername() {
return username;
}
/**
* Sets the username value for this UserSoapDO.
*
* @param username
*/
public void setUsername(java.lang.String username) {
this.username = username;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof UserSoapDO)) return false;
UserSoapDO other = (UserSoapDO) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = super.equals(obj) &&
((this.email==null && other.getEmail()==null) ||
(this.email!=null &&
this.email.equals(other.getEmail()))) &&
((this.fullName==null && other.getFullName()==null) ||
(this.fullName!=null &&
this.fullName.equals(other.getFullName()))) &&
((this.locale==null && other.getLocale()==null) ||
(this.locale!=null &&
this.locale.equals(other.getLocale()))) &&
this.restrictedUser == other.isRestrictedUser() &&
((this.status==null && other.getStatus()==null) ||
(this.status!=null &&
this.status.equals(other.getStatus()))) &&
this.superUser == other.isSuperUser() &&
((this.timeZone==null && other.getTimeZone()==null) ||
(this.timeZone!=null &&
this.timeZone.equals(other.getTimeZone()))) &&
((this.username==null && other.getUsername()==null) ||
(this.username!=null &&
this.username.equals(other.getUsername())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = super.hashCode();
if (getEmail() != null) {
_hashCode += getEmail().hashCode();
}
if (getFullName() != null) {
_hashCode += getFullName().hashCode();
}
if (getLocale() != null) {
_hashCode += getLocale().hashCode();
}
_hashCode += (isRestrictedUser() ? Boolean.TRUE : Boolean.FALSE).hashCode();
if (getStatus() != null) {
_hashCode += getStatus().hashCode();
}
_hashCode += (isSuperUser() ? Boolean.TRUE : Boolean.FALSE).hashCode();
if (getTimeZone() != null) {
_hashCode += getTimeZone().hashCode();
}
if (getUsername() != null) {
_hashCode += getUsername().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(UserSoapDO.class, true);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://schema.vasoftware.com/sf/soap43/type", "UserSoapDO"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("email");
elemField.setXmlName(new javax.xml.namespace.QName("", "email"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("fullName");
elemField.setXmlName(new javax.xml.namespace.QName("", "fullName"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("locale");
elemField.setXmlName(new javax.xml.namespace.QName("", "locale"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("restrictedUser");
elemField.setXmlName(new javax.xml.namespace.QName("", "restrictedUser"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "boolean"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("status");
elemField.setXmlName(new javax.xml.namespace.QName("", "status"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("superUser");
elemField.setXmlName(new javax.xml.namespace.QName("", "superUser"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "boolean"));
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("timeZone");
elemField.setXmlName(new javax.xml.namespace.QName("", "timeZone"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("username");
elemField.setXmlName(new javax.xml.namespace.QName("", "username"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setNillable(true);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy