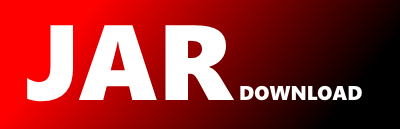
hudson.scm.SubversionReleaseChangeLogSet Maven / Gradle / Ivy
Show all versions of svn-release-mgr Show documentation
package hudson.scm;
import hudson.model.AbstractBuild;
import hudson.model.User;
import hudson.scm.SubversionReleaseChangeLogSet.LogEntry;
import hudson.scm.ChangeLogSet;
import org.kohsuke.stapler.export.Exported;
import org.kohsuke.stapler.export.ExportedBean;
import java.io.IOException;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Comparator;
/**
* {@link ChangeLogSet} for Subversion.
*
* @author Kohsuke Kawaguchi
*/
public final class SubversionReleaseChangeLogSet extends ChangeLogSet {
private final List logs;
/**
* @GuardedBy this
*/
private Map revisionMap;
/*package*/ SubversionReleaseChangeLogSet(AbstractBuild build, List logs) {
super(build);
// we want recent changes first
Collections.sort(logs,new Comparator() {
public int compare(LogEntry a, LogEntry b) {
return b.getRevision()-a.getRevision();
}
});
this.logs = Collections.unmodifiableList(logs);
for (LogEntry log : logs)
log.setParent(this);
}
public boolean isEmptySet() {
return logs.isEmpty();
}
public List getLogs() {
return logs;
}
public Iterator iterator() {
return logs.iterator();
}
public synchronized Map getRevisionMap() throws IOException {
if(revisionMap==null)
revisionMap = SubversionReleaseSCM.parseRevisionFile(build);
return revisionMap;
}
@Exported
public List getRevisions() throws IOException {
List r = new ArrayList();
for (Map.Entry e : getRevisionMap().entrySet())
r.add(new RevisionInfo(e.getKey(),e.getValue()));
return r;
}
@ExportedBean(defaultVisibility=999)
public static final class RevisionInfo {
@Exported public final String module;
@Exported public final long revision;
public RevisionInfo(String module, long revision) {
this.module = module;
this.revision = revision;
}
}
/**
* One commit.
*
* Setter methods are public only so that the objects can be constructed from Digester.
* So please consider this object read-only.
*/
public static class LogEntry extends ChangeLogSet.Entry {
private ChangeLogSet parent;
private int revision;
private User author;
private String date;
private String msg;
private List paths = new ArrayList();
/**
* Gets the {@link SubversionReleaseChangeLogSet} to which this change set belongs.
*/
public SubversionReleaseChangeLogSet getParent() {
return (SubversionReleaseChangeLogSet)getParent();
}
/**
* Gets the revision of the commit.
*
*
* If the commit made the repository revision 1532, this
* method returns 1532.
*/
@Exported
public int getRevision() {
return revision;
}
public void setRevision(int revision) {
this.revision = revision;
}
@Override
public User getAuthor() {
if(author==null)
return User.getUnknown();
return author;
}
@Override
public Collection getAffectedPaths() {
return new AbstractList() {
public String get(int index) {
return paths.get(index).value;
}
public int size() {
return paths.size();
}
};
}
public void setUser(String author) {
this.author = User.get(author);
}
@Exported
public String getUser() {// digester wants read/write property, even though it never reads. Duh.
return author.getDisplayName();
}
@Exported
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
@Override @Exported
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public void addPath( Path p ) {
p.entry = this;
paths.add(p);
}
/**
* Gets the files that are changed in this commit.
* @return
* can be empty but never null.
*/
@Exported
public List getPaths() {
return paths;
}
public void setParent(ChangeLogSet parent) {
this.parent = parent;
}
}
/**
* A file in a commit.
*
* Setter methods are public only so that the objects can be constructed from Digester.
* So please consider this object read-only.
*/
@ExportedBean(defaultVisibility=999)
public static class Path {
private LogEntry entry;
private char action;
private String value;
/**
* Gets the {@link LogEntry} of which this path is a member.
*/
public LogEntry getLogEntry() {
return entry;
}
/**
* Sets the {@link LogEntry} of which this path is a member.
*/
public void setLogEntry(LogEntry entry) {
this.entry = entry;
}
public void setAction(String action) {
this.action = action.charAt(0);
}
/**
* Path in the repository. Such as /test/trunk/foo.c
*/
@Exported(name="file")
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
@Exported
public EditType getEditType() {
if( action=='A' )
return EditType.ADD;
if( action=='D' )
return EditType.DELETE;
return EditType.EDIT;
}
}
}