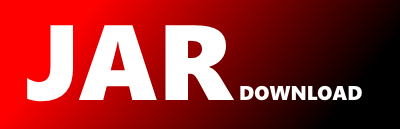
com.gargoylesoftware.htmlunit.html.InputElementFactory Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2008 Gargoyle Software Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gargoylesoftware.htmlunit.html;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.xml.sax.Attributes;
import com.gargoylesoftware.htmlunit.SgmlPage;
import java.util.Map;
import java.util.HashMap;
/**
* A specialized creator that knows how to create input objects.
*
* @version $Revision: 3075 $
* @author Christian Sell
* @author Marc Guillemot
* @author Ahmed Ashour
* @author David K. Taylor
* @author Dmitri Zoubkov
*/
public final class InputElementFactory implements IElementFactory {
/** The singleton instance. */
public static final InputElementFactory instance = new InputElementFactory();
private final transient Log mainLog_ = LogFactory.getLog(getClass());
/** Private singleton constructor. */
private InputElementFactory() {
}
/**
* Creates an HtmlElement for the specified xmlElement, contained in the specified page.
*
* @param page the page that this element will belong to
* @param tagName the HTML tag name
* @param attributes the SAX attributes
*
* @return a new HtmlInput element
*/
public HtmlElement createElement(
final SgmlPage page, final String tagName,
final Attributes attributes) {
return createElementNS(page, null, tagName, attributes);
}
/**
* {@inheritDoc}
*/
public HtmlElement createElementNS(final SgmlPage page, final String namespaceURI,
final String qualifiedName, final Attributes attributes) {
Map attributeMap = DefaultElementFactory.setAttributes(page, attributes);
if (attributeMap == null) {
attributeMap = new HashMap();
}
String type = null;
if (attributes != null) {
type = attributes.getValue("type");
}
if (type == null) {
type = "";
}
else {
type = type.toLowerCase();
attributeMap.get("type").setValue(type); // type value has to be lower case
}
final HtmlInput result;
if (type.length() == 0) {
// This not an illegal value, as it defaults to "text"
// cf http://www.w3.org/TR/REC-html40/interact/forms.html#adef-type-INPUT
// and the common browsers seem to treat it as a "text" input so we will as well.
HtmlElement.addAttributeToMap(page, attributeMap, null, "type", "text");
result = new HtmlTextInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("submit")) {
result = new HtmlSubmitInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("checkbox")) {
result = new HtmlCheckBoxInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("radio")) {
result = new HtmlRadioButtonInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("text")) {
result = new HtmlTextInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("hidden")) {
result = new HtmlHiddenInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("password")) {
result = new HtmlPasswordInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("image")) {
result = new HtmlImageInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("reset")) {
result = new HtmlResetInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("button")) {
result = new HtmlButtonInput(namespaceURI, qualifiedName, page, attributeMap);
}
else if (type.equals("file")) {
result = new HtmlFileInput(namespaceURI, qualifiedName, page, attributeMap);
}
else {
if (mainLog_.isInfoEnabled()) {
mainLog_.info("Bad input type: \"" + type + "\", creating a text input");
}
result = new HtmlTextInput(namespaceURI, qualifiedName, page, attributeMap);
}
return result;
}
/**
* Returns the log that is being used for all scripting objects.
* @return the log
* @deprecated As of 2.0, use local log variable enclosed in a conditional block.
*/
@Deprecated
protected Log getLog() {
return mainLog_;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy