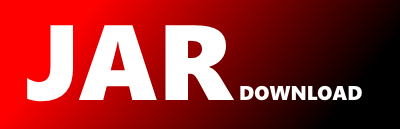
org.jvnet.hyperjaxb3.xml.bind.JAXBContextUtils Maven / Gradle / Ivy
package org.jvnet.hyperjaxb3.xml.bind;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.Map;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.namespace.QName;
import org.jvnet.hyperjaxb3.xml.bind.annotation.adapters.ElementAsString;
import org.jvnet.hyperjaxb3.xml.bind.annotation.adapters.XmlAdapterUtils;
import org.w3c.dom.Element;
public class JAXBContextUtils {
private JAXBContextUtils() {
}
public static boolean isElement(String contextPath, Object object) {
try {
return object != null
&& getJAXBContext(contextPath).createJAXBIntrospector()
.isElement(object);
} catch (JAXBException ex) {
throw new RuntimeException(ex);
}
}
public static boolean isMarshallable(String contextPath, Object object) {
try {
return object != null
&& (object instanceof Element || getJAXBContext(contextPath)
.createJAXBIntrospector().isElement(object));
} catch (JAXBException ex) {
throw new RuntimeException(ex);
}
}
public static boolean isMarshallableElement(Object object) {
return object != null && (object instanceof Element);
}
public static String unmarshallElement(Object object) {
return object != null && (object instanceof Element) ? XmlAdapterUtils
.unmarshall(ElementAsString.class, (Element) object) : null;
}
public static Object marshallElement(String object) {
return object == null ? null :
XmlAdapterUtils.marshall(
ElementAsString.class, object);
}
public static boolean isMarshallableObject(String contextPath, Object object) {
try {
return object != null
&& (getJAXBContext(contextPath).createJAXBIntrospector()
.isElement(object));
} catch (JAXBException ex) {
throw new RuntimeException(ex);
}
}
public static String unmarshallObject(String contextPath, Object object) {
if (object != null)
{
try {
final Marshaller marshaller = getJAXBContext(contextPath).createMarshaller();
final StringWriter sw = new StringWriter();
marshaller.marshal(object, sw);
return sw.toString();
} catch (JAXBException ex) {
throw new RuntimeException(ex);
}
}
else
{
return null;
}
}
public static Object marshallObject(String contextPath, String object) {
if (object == null) {
return null;
} else {
final Element element = XmlAdapterUtils.marshall(
ElementAsString.class, object);
try {
final Unmarshaller unmarshaller = getJAXBContext(contextPath).createUnmarshaller();
return unmarshaller.unmarshal(element);
} catch (JAXBException ex) {
return element;
}
}
}
public static String unmarshall(String contextPath, Object object) {
try {
return object == null ? null : unmarshall(
getJAXBContext(contextPath), object);
} catch (JAXBException ex) {
throw new RuntimeException(ex);
}
}
public static String unmarshallJAXBElement(String contextPath,
JAXBElement
© 2015 - 2025 Weber Informatics LLC | Privacy Policy