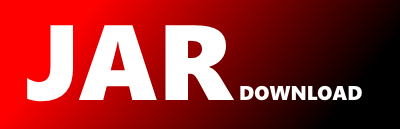
com.sun.java.xml.ns.persistence.Persistence Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-792
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2010.01.20 at 09:50:07 PM GMT
//
package com.sun.java.xml.ns.persistence;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.jvnet.jaxb2_commons.lang.CopyTo;
import org.jvnet.jaxb2_commons.lang.Copyable;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.MergeFrom;
import org.jvnet.jaxb2_commons.lang.Mergeable;
import org.jvnet.jaxb2_commons.lang.builder.CopyBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBCopyBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBEqualsBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBHashCodeBuilder;
import org.jvnet.jaxb2_commons.lang.builder.MergeBuilder;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="persistence-unit" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="provider" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="jta-data-source" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="non-jta-data-source" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="mapping-file" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="jar-file" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="class" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="exclude-unlisted-classes" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="properties" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="property" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="value" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="transaction-type" type="{http://java.sun.com/xml/ns/persistence}persistence-unit-transaction-type" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <attribute name="version" use="required" type="{http://java.sun.com/xml/ns/persistence}versionType" fixed="1.0" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"persistenceUnit"
})
@XmlRootElement(name = "persistence")
public class Persistence
implements CopyTo, Copyable, Equals, HashCode, MergeFrom, Mergeable
{
@XmlElement(name = "persistence-unit")
protected List persistenceUnit;
@XmlAttribute(required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String version;
/**
* Gets the value of the persistenceUnit property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the persistenceUnit property.
*
*
* For example, to add a new item, do as follows:
*
* getPersistenceUnit().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Persistence.PersistenceUnit }
*
*
*/
public List getPersistenceUnit() {
if (persistenceUnit == null) {
persistenceUnit = new ArrayList();
}
return this.persistenceUnit;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
if (version == null) {
return "1.0";
} else {
return version;
}
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Persistence)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Persistence that = ((Persistence) object);
equalsBuilder.append(this.getPersistenceUnit(), that.getPersistenceUnit());
equalsBuilder.append(this.getVersion(), that.getVersion());
}
public boolean equals(Object object) {
if (!(object instanceof Persistence)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getPersistenceUnit());
hashCodeBuilder.append(this.getVersion());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
public Object copyTo(Object target, CopyBuilder copyBuilder) {
final Persistence copy = ((target == null)?((Persistence) createCopy()):((Persistence) target));
if ((this.persistenceUnit!= null)&&(!this.persistenceUnit.isEmpty())) {
List sourcePersistenceUnit;
sourcePersistenceUnit = this.getPersistenceUnit();
@SuppressWarnings("unchecked")
List copyPersistenceUnit = ((List ) copyBuilder.copy(sourcePersistenceUnit));
copy.persistenceUnit = null;
List uniquePersistenceUnitl = copy.getPersistenceUnit();
uniquePersistenceUnitl.addAll(copyPersistenceUnit);
} else {
copy.persistenceUnit = null;
}
if (this.version!= null) {
String sourceVersion;
sourceVersion = this.getVersion();
String copyVersion = ((String) copyBuilder.copy(sourceVersion));
copy.setVersion(copyVersion);
} else {
copy.version = null;
}
return copy;
}
public Object copyTo(Object target) {
final CopyBuilder copyBuilder = new JAXBCopyBuilder();
return copyTo(target, copyBuilder);
}
public Object createCopy() {
return new Persistence();
}
public Object mergeFrom(Object leftObject, Object rightObject, MergeBuilder mergeBuilder) {
if ((leftObject instanceof Persistence)&&(rightObject instanceof Persistence)) {
final Persistence target = this;
final Persistence left = ((Persistence) leftObject);
final Persistence right = ((Persistence) rightObject);
{
List leftPersistenceUnit;
leftPersistenceUnit = left.getPersistenceUnit();
List rightPersistenceUnit;
rightPersistenceUnit = right.getPersistenceUnit();
target.persistenceUnit = null;
List uniquePersistenceUnitl = target.getPersistenceUnit();
uniquePersistenceUnitl.addAll(mergeBuilder.merge(this, target, "PersistenceUnit", leftPersistenceUnit, rightPersistenceUnit));
}
{
String leftVersion;
leftVersion = left.getVersion();
String rightVersion;
rightVersion = right.getVersion();
target.setVersion(mergeBuilder.merge(this, target, "Version", leftVersion, rightVersion));
}
}
return this;
}
public Object mergeFrom(Object left, Object right) {
final MergeBuilder mergeBuilder = new MergeBuilder();
return mergeFrom(left, right, mergeBuilder);
}
/**
*
*
* Configuration of a persistence unit.
*
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="provider" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="jta-data-source" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="non-jta-data-source" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="mapping-file" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="jar-file" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="class" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="exclude-unlisted-classes" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="properties" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="property" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="value" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="transaction-type" type="{http://java.sun.com/xml/ns/persistence}persistence-unit-transaction-type" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"description",
"provider",
"jtaDataSource",
"nonJtaDataSource",
"mappingFile",
"jarFile",
"clazz",
"excludeUnlistedClasses",
"properties"
})
public static class PersistenceUnit
implements CopyTo, Copyable, Equals, HashCode, MergeFrom, Mergeable
{
protected String description;
protected String provider;
@XmlElement(name = "jta-data-source")
protected String jtaDataSource;
@XmlElement(name = "non-jta-data-source")
protected String nonJtaDataSource;
@XmlElement(name = "mapping-file")
protected List mappingFile;
@XmlElement(name = "jar-file")
protected List jarFile;
@XmlElement(name = "class")
protected List clazz;
@XmlElement(name = "exclude-unlisted-classes", defaultValue = "false")
protected Boolean excludeUnlistedClasses;
protected Persistence.PersistenceUnit.Properties properties;
@XmlAttribute(required = true)
protected String name;
@XmlAttribute(name = "transaction-type")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String transactionType;
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the provider property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProvider() {
return provider;
}
/**
* Sets the value of the provider property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProvider(String value) {
this.provider = value;
}
/**
* Gets the value of the jtaDataSource property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJtaDataSource() {
return jtaDataSource;
}
/**
* Sets the value of the jtaDataSource property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJtaDataSource(String value) {
this.jtaDataSource = value;
}
/**
* Gets the value of the nonJtaDataSource property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNonJtaDataSource() {
return nonJtaDataSource;
}
/**
* Sets the value of the nonJtaDataSource property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNonJtaDataSource(String value) {
this.nonJtaDataSource = value;
}
/**
* Gets the value of the mappingFile property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the mappingFile property.
*
*
* For example, to add a new item, do as follows:
*
* getMappingFile().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getMappingFile() {
if (mappingFile == null) {
mappingFile = new ArrayList();
}
return this.mappingFile;
}
/**
* Gets the value of the jarFile property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the jarFile property.
*
*
* For example, to add a new item, do as follows:
*
* getJarFile().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getJarFile() {
if (jarFile == null) {
jarFile = new ArrayList();
}
return this.jarFile;
}
/**
* Gets the value of the clazz property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the clazz property.
*
*
* For example, to add a new item, do as follows:
*
* getClazz().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getClazz() {
if (clazz == null) {
clazz = new ArrayList();
}
return this.clazz;
}
/**
* Gets the value of the excludeUnlistedClasses property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isExcludeUnlistedClasses() {
return excludeUnlistedClasses;
}
/**
* Sets the value of the excludeUnlistedClasses property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setExcludeUnlistedClasses(Boolean value) {
this.excludeUnlistedClasses = value;
}
/**
* Gets the value of the properties property.
*
* @return
* possible object is
* {@link Persistence.PersistenceUnit.Properties }
*
*/
public Persistence.PersistenceUnit.Properties getProperties() {
return properties;
}
/**
* Sets the value of the properties property.
*
* @param value
* allowed object is
* {@link Persistence.PersistenceUnit.Properties }
*
*/
public void setProperties(Persistence.PersistenceUnit.Properties value) {
this.properties = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the transactionType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionType() {
return transactionType;
}
/**
* Sets the value of the transactionType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionType(String value) {
this.transactionType = value;
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Persistence.PersistenceUnit)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Persistence.PersistenceUnit that = ((Persistence.PersistenceUnit) object);
equalsBuilder.append(this.getDescription(), that.getDescription());
equalsBuilder.append(this.getProvider(), that.getProvider());
equalsBuilder.append(this.getJtaDataSource(), that.getJtaDataSource());
equalsBuilder.append(this.getNonJtaDataSource(), that.getNonJtaDataSource());
equalsBuilder.append(this.getMappingFile(), that.getMappingFile());
equalsBuilder.append(this.getJarFile(), that.getJarFile());
equalsBuilder.append(this.getClazz(), that.getClazz());
equalsBuilder.append(this.isExcludeUnlistedClasses(), that.isExcludeUnlistedClasses());
equalsBuilder.append(this.getProperties(), that.getProperties());
equalsBuilder.append(this.getName(), that.getName());
equalsBuilder.append(this.getTransactionType(), that.getTransactionType());
}
public boolean equals(Object object) {
if (!(object instanceof Persistence.PersistenceUnit)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getDescription());
hashCodeBuilder.append(this.getProvider());
hashCodeBuilder.append(this.getJtaDataSource());
hashCodeBuilder.append(this.getNonJtaDataSource());
hashCodeBuilder.append(this.getMappingFile());
hashCodeBuilder.append(this.getJarFile());
hashCodeBuilder.append(this.getClazz());
hashCodeBuilder.append(this.isExcludeUnlistedClasses());
hashCodeBuilder.append(this.getProperties());
hashCodeBuilder.append(this.getName());
hashCodeBuilder.append(this.getTransactionType());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
public Object copyTo(Object target, CopyBuilder copyBuilder) {
final Persistence.PersistenceUnit copy = ((target == null)?((Persistence.PersistenceUnit) createCopy()):((Persistence.PersistenceUnit) target));
if (this.description!= null) {
String sourceDescription;
sourceDescription = this.getDescription();
String copyDescription = ((String) copyBuilder.copy(sourceDescription));
copy.setDescription(copyDescription);
} else {
copy.description = null;
}
if (this.provider!= null) {
String sourceProvider;
sourceProvider = this.getProvider();
String copyProvider = ((String) copyBuilder.copy(sourceProvider));
copy.setProvider(copyProvider);
} else {
copy.provider = null;
}
if (this.jtaDataSource!= null) {
String sourceJtaDataSource;
sourceJtaDataSource = this.getJtaDataSource();
String copyJtaDataSource = ((String) copyBuilder.copy(sourceJtaDataSource));
copy.setJtaDataSource(copyJtaDataSource);
} else {
copy.jtaDataSource = null;
}
if (this.nonJtaDataSource!= null) {
String sourceNonJtaDataSource;
sourceNonJtaDataSource = this.getNonJtaDataSource();
String copyNonJtaDataSource = ((String) copyBuilder.copy(sourceNonJtaDataSource));
copy.setNonJtaDataSource(copyNonJtaDataSource);
} else {
copy.nonJtaDataSource = null;
}
if ((this.mappingFile!= null)&&(!this.mappingFile.isEmpty())) {
List sourceMappingFile;
sourceMappingFile = this.getMappingFile();
@SuppressWarnings("unchecked")
List copyMappingFile = ((List ) copyBuilder.copy(sourceMappingFile));
copy.mappingFile = null;
List uniqueMappingFilel = copy.getMappingFile();
uniqueMappingFilel.addAll(copyMappingFile);
} else {
copy.mappingFile = null;
}
if ((this.jarFile!= null)&&(!this.jarFile.isEmpty())) {
List sourceJarFile;
sourceJarFile = this.getJarFile();
@SuppressWarnings("unchecked")
List copyJarFile = ((List ) copyBuilder.copy(sourceJarFile));
copy.jarFile = null;
List uniqueJarFilel = copy.getJarFile();
uniqueJarFilel.addAll(copyJarFile);
} else {
copy.jarFile = null;
}
if ((this.clazz!= null)&&(!this.clazz.isEmpty())) {
List sourceClazz;
sourceClazz = this.getClazz();
@SuppressWarnings("unchecked")
List copyClazz = ((List ) copyBuilder.copy(sourceClazz));
copy.clazz = null;
List uniqueClazzl = copy.getClazz();
uniqueClazzl.addAll(copyClazz);
} else {
copy.clazz = null;
}
if (this.excludeUnlistedClasses!= null) {
Boolean sourceExcludeUnlistedClasses;
sourceExcludeUnlistedClasses = this.isExcludeUnlistedClasses();
Boolean copyExcludeUnlistedClasses = ((Boolean) copyBuilder.copy(sourceExcludeUnlistedClasses));
copy.setExcludeUnlistedClasses(copyExcludeUnlistedClasses);
} else {
copy.excludeUnlistedClasses = null;
}
if (this.properties!= null) {
Persistence.PersistenceUnit.Properties sourceProperties;
sourceProperties = this.getProperties();
Persistence.PersistenceUnit.Properties copyProperties = ((Persistence.PersistenceUnit.Properties) copyBuilder.copy(sourceProperties));
copy.setProperties(copyProperties);
} else {
copy.properties = null;
}
if (this.name!= null) {
String sourceName;
sourceName = this.getName();
String copyName = ((String) copyBuilder.copy(sourceName));
copy.setName(copyName);
} else {
copy.name = null;
}
if (this.transactionType!= null) {
String sourceTransactionType;
sourceTransactionType = this.getTransactionType();
String copyTransactionType = ((String) copyBuilder.copy(sourceTransactionType));
copy.setTransactionType(copyTransactionType);
} else {
copy.transactionType = null;
}
return copy;
}
public Object copyTo(Object target) {
final CopyBuilder copyBuilder = new JAXBCopyBuilder();
return copyTo(target, copyBuilder);
}
public Object createCopy() {
return new Persistence.PersistenceUnit();
}
public Object mergeFrom(Object leftObject, Object rightObject, MergeBuilder mergeBuilder) {
if ((leftObject instanceof Persistence.PersistenceUnit)&&(rightObject instanceof Persistence.PersistenceUnit)) {
final Persistence.PersistenceUnit target = this;
final Persistence.PersistenceUnit left = ((Persistence.PersistenceUnit) leftObject);
final Persistence.PersistenceUnit right = ((Persistence.PersistenceUnit) rightObject);
{
String leftDescription;
leftDescription = left.getDescription();
String rightDescription;
rightDescription = right.getDescription();
target.setDescription(mergeBuilder.merge(this, target, "Description", leftDescription, rightDescription));
}
{
String leftProvider;
leftProvider = left.getProvider();
String rightProvider;
rightProvider = right.getProvider();
target.setProvider(mergeBuilder.merge(this, target, "Provider", leftProvider, rightProvider));
}
{
String leftJtaDataSource;
leftJtaDataSource = left.getJtaDataSource();
String rightJtaDataSource;
rightJtaDataSource = right.getJtaDataSource();
target.setJtaDataSource(mergeBuilder.merge(this, target, "JtaDataSource", leftJtaDataSource, rightJtaDataSource));
}
{
String leftNonJtaDataSource;
leftNonJtaDataSource = left.getNonJtaDataSource();
String rightNonJtaDataSource;
rightNonJtaDataSource = right.getNonJtaDataSource();
target.setNonJtaDataSource(mergeBuilder.merge(this, target, "NonJtaDataSource", leftNonJtaDataSource, rightNonJtaDataSource));
}
{
List leftMappingFile;
leftMappingFile = left.getMappingFile();
List rightMappingFile;
rightMappingFile = right.getMappingFile();
target.mappingFile = null;
List uniqueMappingFilel = target.getMappingFile();
uniqueMappingFilel.addAll(mergeBuilder.merge(this, target, "MappingFile", leftMappingFile, rightMappingFile));
}
{
List leftJarFile;
leftJarFile = left.getJarFile();
List rightJarFile;
rightJarFile = right.getJarFile();
target.jarFile = null;
List uniqueJarFilel = target.getJarFile();
uniqueJarFilel.addAll(mergeBuilder.merge(this, target, "JarFile", leftJarFile, rightJarFile));
}
{
List leftClazz;
leftClazz = left.getClazz();
List rightClazz;
rightClazz = right.getClazz();
target.clazz = null;
List uniqueClazzl = target.getClazz();
uniqueClazzl.addAll(mergeBuilder.merge(this, target, "Clazz", leftClazz, rightClazz));
}
{
Boolean leftExcludeUnlistedClasses;
leftExcludeUnlistedClasses = left.isExcludeUnlistedClasses();
Boolean rightExcludeUnlistedClasses;
rightExcludeUnlistedClasses = right.isExcludeUnlistedClasses();
target.setExcludeUnlistedClasses(mergeBuilder.merge(this, target, "ExcludeUnlistedClasses", leftExcludeUnlistedClasses, rightExcludeUnlistedClasses));
}
{
Persistence.PersistenceUnit.Properties leftProperties;
leftProperties = left.getProperties();
Persistence.PersistenceUnit.Properties rightProperties;
rightProperties = right.getProperties();
target.setProperties(mergeBuilder.merge(this, target, "Properties", leftProperties, rightProperties));
}
{
String leftName;
leftName = left.getName();
String rightName;
rightName = right.getName();
target.setName(mergeBuilder.merge(this, target, "Name", leftName, rightName));
}
{
String leftTransactionType;
leftTransactionType = left.getTransactionType();
String rightTransactionType;
rightTransactionType = right.getTransactionType();
target.setTransactionType(mergeBuilder.merge(this, target, "TransactionType", leftTransactionType, rightTransactionType));
}
}
return this;
}
public Object mergeFrom(Object left, Object right) {
final MergeBuilder mergeBuilder = new MergeBuilder();
return mergeFrom(left, right, mergeBuilder);
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="property" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="value" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"property"
})
public static class Properties
implements CopyTo, Copyable, Equals, HashCode, MergeFrom, Mergeable
{
protected List property;
/**
* Gets the value of the property property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the property property.
*
*
* For example, to add a new item, do as follows:
*
* getProperty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Persistence.PersistenceUnit.Properties.Property }
*
*
*/
public List getProperty() {
if (property == null) {
property = new ArrayList();
}
return this.property;
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Persistence.PersistenceUnit.Properties)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Persistence.PersistenceUnit.Properties that = ((Persistence.PersistenceUnit.Properties) object);
equalsBuilder.append(this.getProperty(), that.getProperty());
}
public boolean equals(Object object) {
if (!(object instanceof Persistence.PersistenceUnit.Properties)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getProperty());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
public Object copyTo(Object target, CopyBuilder copyBuilder) {
final Persistence.PersistenceUnit.Properties copy = ((target == null)?((Persistence.PersistenceUnit.Properties) createCopy()):((Persistence.PersistenceUnit.Properties) target));
if ((this.property!= null)&&(!this.property.isEmpty())) {
List sourceProperty;
sourceProperty = this.getProperty();
@SuppressWarnings("unchecked")
List copyProperty = ((List ) copyBuilder.copy(sourceProperty));
copy.property = null;
List uniquePropertyl = copy.getProperty();
uniquePropertyl.addAll(copyProperty);
} else {
copy.property = null;
}
return copy;
}
public Object copyTo(Object target) {
final CopyBuilder copyBuilder = new JAXBCopyBuilder();
return copyTo(target, copyBuilder);
}
public Object createCopy() {
return new Persistence.PersistenceUnit.Properties();
}
public Object mergeFrom(Object leftObject, Object rightObject, MergeBuilder mergeBuilder) {
if ((leftObject instanceof Persistence.PersistenceUnit.Properties)&&(rightObject instanceof Persistence.PersistenceUnit.Properties)) {
final Persistence.PersistenceUnit.Properties target = this;
final Persistence.PersistenceUnit.Properties left = ((Persistence.PersistenceUnit.Properties) leftObject);
final Persistence.PersistenceUnit.Properties right = ((Persistence.PersistenceUnit.Properties) rightObject);
{
List leftProperty;
leftProperty = left.getProperty();
List rightProperty;
rightProperty = right.getProperty();
target.property = null;
List uniquePropertyl = target.getProperty();
uniquePropertyl.addAll(mergeBuilder.merge(this, target, "Property", leftProperty, rightProperty));
}
}
return this;
}
public Object mergeFrom(Object left, Object right) {
final MergeBuilder mergeBuilder = new MergeBuilder();
return mergeFrom(left, right, mergeBuilder);
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="value" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Property
implements CopyTo, Copyable, Equals, HashCode, MergeFrom, Mergeable
{
@XmlAttribute(required = true)
protected String name;
@XmlAttribute(required = true)
protected String value;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Persistence.PersistenceUnit.Properties.Property)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Persistence.PersistenceUnit.Properties.Property that = ((Persistence.PersistenceUnit.Properties.Property) object);
equalsBuilder.append(this.getName(), that.getName());
equalsBuilder.append(this.getValue(), that.getValue());
}
public boolean equals(Object object) {
if (!(object instanceof Persistence.PersistenceUnit.Properties.Property)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getName());
hashCodeBuilder.append(this.getValue());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
public Object copyTo(Object target, CopyBuilder copyBuilder) {
final Persistence.PersistenceUnit.Properties.Property copy = ((target == null)?((Persistence.PersistenceUnit.Properties.Property) createCopy()):((Persistence.PersistenceUnit.Properties.Property) target));
if (this.name!= null) {
String sourceName;
sourceName = this.getName();
String copyName = ((String) copyBuilder.copy(sourceName));
copy.setName(copyName);
} else {
copy.name = null;
}
if (this.value!= null) {
String sourceValue;
sourceValue = this.getValue();
String copyValue = ((String) copyBuilder.copy(sourceValue));
copy.setValue(copyValue);
} else {
copy.value = null;
}
return copy;
}
public Object copyTo(Object target) {
final CopyBuilder copyBuilder = new JAXBCopyBuilder();
return copyTo(target, copyBuilder);
}
public Object createCopy() {
return new Persistence.PersistenceUnit.Properties.Property();
}
public Object mergeFrom(Object leftObject, Object rightObject, MergeBuilder mergeBuilder) {
if ((leftObject instanceof Persistence.PersistenceUnit.Properties.Property)&&(rightObject instanceof Persistence.PersistenceUnit.Properties.Property)) {
final Persistence.PersistenceUnit.Properties.Property target = this;
final Persistence.PersistenceUnit.Properties.Property left = ((Persistence.PersistenceUnit.Properties.Property) leftObject);
final Persistence.PersistenceUnit.Properties.Property right = ((Persistence.PersistenceUnit.Properties.Property) rightObject);
{
String leftName;
leftName = left.getName();
String rightName;
rightName = right.getName();
target.setName(mergeBuilder.merge(this, target, "Name", leftName, rightName));
}
{
String leftValue;
leftValue = left.getValue();
String rightValue;
rightValue = right.getValue();
target.setValue(mergeBuilder.merge(this, target, "Value", leftValue, rightValue));
}
}
return this;
}
public Object mergeFrom(Object left, Object right) {
final MergeBuilder mergeBuilder = new MergeBuilder();
return mergeFrom(left, right, mergeBuilder);
}
}
}
}
}