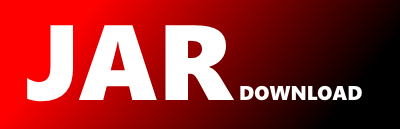
org.jvnet.hyperjaxb3.hibernate.customizations.MappingCreationStrategy Maven / Gradle / Ivy
The newest version!
package org.jvnet.hyperjaxb3.hibernate.customizations;
import java.util.Collection;
import java.util.List;
import org.jvnet.hyperjaxb3.hibernate.customizations.AbstractCollectionPropertyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.CacheType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ClassType;
import org.jvnet.hyperjaxb3.hibernate.customizations.CollectionIdType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ColumnType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ComponentType;
import org.jvnet.hyperjaxb3.hibernate.customizations.CompositeElementType;
import org.jvnet.hyperjaxb3.hibernate.customizations.DiscriminatorType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ElementType;
import org.jvnet.hyperjaxb3.hibernate.customizations.GeneratorType;
import org.jvnet.hyperjaxb3.hibernate.customizations.IdType;
import org.jvnet.hyperjaxb3.hibernate.customizations.KeyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ListIndexType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ManyToAnyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ManyToManyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.ManyToOneType;
import org.jvnet.hyperjaxb3.hibernate.customizations.OneToManyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.OneToOneType;
import org.jvnet.hyperjaxb3.hibernate.customizations.SimpleSinglePropertyType;
import org.jvnet.hyperjaxb3.hibernate.customizations.TypeType;
import org.jvnet.hyperjaxb3.hibernate.customizations.VersionType;
import org.jvnet.hyperjaxb3.hibernate.customizations.WildcardSinglePropertyType;
import com.sun.tools.xjc.outline.ClassOutline;
import com.sun.tools.xjc.outline.FieldOutline;
public interface MappingCreationStrategy {
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Column createColumn(
final ColumnType ccolumn, final String defaultColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Type createType(
final TypeType ctype, final TypeType defaultType);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.List createList(
final AbstractCollectionPropertyType cproperty, final String name,
final String defaultTableName, final String defaultKeyColumnName,
final String defaultListIndexColumnName,
final String defaultAccessorClassName, final Collection content);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Bag createBag(
final AbstractCollectionPropertyType cproperty, final String name,
final String defaultTableName, final String defaultKeyColumnName,
final String defaultAccessorClassName, final Collection content);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Idbag createIdbag(
final AbstractCollectionPropertyType cproperty, final String name,
final String defaultTableName, final String defaultKeyColumnName,
final String defaultCollectionIdType,
final String defaultCollectionIdColumnName,
final String defaultCollectionIdGeneratorClassName,
final String defaultAccessorClassName, final Collection content);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Cache createCache(
final CacheType ccache, String defaultUsage);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Key createKey(
final KeyType ckey, String defaultKeyColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.ListIndex createListIndex(
ListIndexType clistIndex, String defaultListIndexColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Element createElement(
ElementType celement, TypeType defaultType,
String defaultElementColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.OneToMany createOneToMany(
OneToManyType coneToMany, String clazz);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.ManyToMany createManyToMany(
ManyToManyType cmanyToMany, String clazz,
String defaultManyToManyColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Any createAny(
final WildcardSinglePropertyType cproperty, final String name,
final String idType, final String defaultMetaType,
final String defaultAnyClassColumnName,
final String defaultAnyIdColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.ManyToAny createManyToAny(
ManyToAnyType cproperty, String idType, String defaultMetaType,
String defaultManyToAnyClassColumnName,
String defaultManyToAnyIdColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Property createProperty(
final SimpleSinglePropertyType cproperty,
final String propertyName, final String defaultColumnName,
final TypeType defaultType, final String defaultAccessorClassName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.OneToOne createOneToOne(
final OneToOneType coneToOne, final String propertyName,
final String clazz);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.ManyToOne createManyToOne(
final ManyToOneType cmanyToOne, final String propertyName,
final String defaultColumnName, final String clazz);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Clazz createClass(
final ClassType cclass,
final String className,
final String defaultTableName,
final String defaultDiscriminatorValue,
final String defaultCacheUsage,
final org.jvnet.hyperjaxb3.hibernate.mapping.Id id,
final org.jvnet.hyperjaxb3.hibernate.mapping.Discriminator discriminator,
final org.jvnet.hyperjaxb3.hibernate.mapping.Version version,
final List fieldMappings);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Subclass createSubclass(
final ClassType cclass, String className, String superClassName,
String defaultDiscriminatorValue, List fieldMappings,
String defaultTableName, String defaultIdentifierColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Join createJoin(
final ClassType cclass, final List content,
final String defaultTableName, final String defaultKeyColumnName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Generator createGenerator(
final GeneratorType cgenerator, final String defaultGeneratorClass);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.CollectionId createCollectionId(
final CollectionIdType cid, final String defaultIdType,
final String defaultColumnName, final String defaultGeneratorClass);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Id createId(
final IdType cid, final String fieldPropertyName,
final String idType, final String defaultGeneratorClass,
final String defaultAccessorClassName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Version createVersion(
final VersionType cversion, final String fieldPropertyName,
final String fieldType, final String defaultAccessorClassName);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Discriminator createDiscriminator(
final DiscriminatorType cdiscriminator, final String defaultName,
final String defaultType);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.Component createComponent(
ComponentType ccomponent, String name, String clazz,
List fieldMappings);
public abstract org.jvnet.hyperjaxb3.hibernate.mapping.CompositeElement createCompositeElement(
CompositeElementType ccompositeElement, String clazz,
List fieldMappings);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy