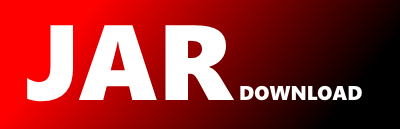
org.jvnet.hyperjaxb3.tests.po.USAddress Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0.2-b01-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2007.01.30 at 01:24:58 PM CET
//
package org.jvnet.hyperjaxb3.tests.po;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.builder.JAXBEqualsBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBHashCodeBuilder;
/**
* Java class for USAddress complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="USAddress">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="street" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="state" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="zip" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* </sequence>
* <attribute name="country" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" fixed="US" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "USAddress", propOrder = {
"name",
"street",
"city",
"state",
"zip"
})
public class USAddress
implements Equals, HashCode
{
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String street;
@XmlElement(required = true)
protected String city;
@XmlElement(required = true)
protected String state;
@XmlElement(required = true)
protected BigDecimal zip;
@XmlAttribute
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String country;
@XmlAttribute
protected Long hjid;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
public boolean isSetName() {
return (this.name!= null);
}
/**
* Gets the value of the street property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet() {
return street;
}
/**
* Sets the value of the street property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet(String value) {
this.street = value;
}
public boolean isSetStreet() {
return (this.street!= null);
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCity() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCity(String value) {
this.city = value;
}
public boolean isSetCity() {
return (this.city!= null);
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setState(String value) {
this.state = value;
}
public boolean isSetState() {
return (this.state!= null);
}
/**
* Gets the value of the zip property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getZip() {
return zip;
}
/**
* Sets the value of the zip property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setZip(BigDecimal value) {
this.zip = value;
}
public boolean isSetZip() {
return (this.zip!= null);
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
if (country == null) {
return "US";
} else {
return country;
}
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
public boolean isSetCountry() {
return (this.country!= null);
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof USAddress)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final USAddress rhs = ((USAddress) object);
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = rhs.getName();
equalsBuilder.append(lhsName, rhsName);
}
{
String lhsStreet;
lhsStreet = this.getStreet();
String rhsStreet;
rhsStreet = rhs.getStreet();
equalsBuilder.append(lhsStreet, rhsStreet);
}
{
String lhsCity;
lhsCity = this.getCity();
String rhsCity;
rhsCity = rhs.getCity();
equalsBuilder.append(lhsCity, rhsCity);
}
{
String lhsState;
lhsState = this.getState();
String rhsState;
rhsState = rhs.getState();
equalsBuilder.append(lhsState, rhsState);
}
{
BigDecimal lhsZip;
lhsZip = this.getZip();
BigDecimal rhsZip;
rhsZip = rhs.getZip();
equalsBuilder.append(lhsZip, rhsZip);
}
{
String lhsCountry;
lhsCountry = this.getCountry();
String rhsCountry;
rhsCountry = rhs.getCountry();
equalsBuilder.append(lhsCountry, rhsCountry);
}
}
public boolean equals(Object object) {
if (!(object instanceof USAddress)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
{
String theName;
theName = this.getName();
hashCodeBuilder.append(theName);
}
{
String theStreet;
theStreet = this.getStreet();
hashCodeBuilder.append(theStreet);
}
{
String theCity;
theCity = this.getCity();
hashCodeBuilder.append(theCity);
}
{
String theState;
theState = this.getState();
hashCodeBuilder.append(theState);
}
{
BigDecimal theZip;
theZip = this.getZip();
hashCodeBuilder.append(theZip);
}
{
String theCountry;
theCountry = this.getCountry();
hashCodeBuilder.append(theCountry);
}
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
/**
* Gets the value of the hjid property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getHjid() {
return hjid;
}
/**
* Sets the value of the hjid property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setHjid(Long value) {
this.hjid = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy