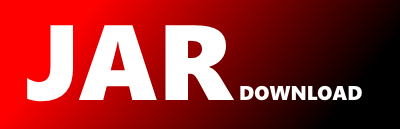
com.sun.xml.ws.commons.virtualbox.IParallelPort Maven / Gradle / Ivy
The newest version!
package com.sun.xml.ws.commons.virtualbox;
import javax.xml.ws.WebServiceException;
/**
* The IParallelPort interface represents the virtual parallel port device.
*
* The virtual parallel port device acts like an ordinary parallel port
* inside the virtual machine. This device communicates to the real
* parallel port hardware using the name of the parallel device on the host
* computer specified in the #path attribute.
*
* Each virtual parallel port device is assigned a base I/O address and an
* IRQ number that will be reported to the guest operating system and used
* to operate the given parallel port from within the virtual machine.
* @see IMachine::getParallelPort
*
*/
public class IParallelPort
extends VBoxObject
{
public IParallelPort(String _this, VboxPortType port) {
super(_this,port);
}
/**
* Slot number this parallel port is plugged into. Corresponds to
* the value you pass to {@link IMachine#getParallelPort} to obtain this instance.
*
*/
public long getSlot() {
try {
long retVal = port.iParallelPortGetSlot(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Flag whether the parallel port is enabled. If disabled,
* the parallel port will not be reported to the guest OS.
*
*/
public boolean getEnabled() {
try {
boolean retVal = port.iParallelPortGetEnabled(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Flag whether the parallel port is enabled. If disabled,
* the parallel port will not be reported to the guest OS.
*
*/
public void setEnabled(boolean value) {
try {
port.iParallelPortSetEnabled(_this, value);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Base I/O address of the parallel port.
*
*/
public long getIOBase() {
try {
long retVal = port.iParallelPortGetIOBase(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Base I/O address of the parallel port.
*
*/
public void setIOBase(long value) {
try {
port.iParallelPortSetIOBase(_this, value);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* IRQ number of the parallel port.
*
*/
public long getIRQ() {
try {
long retVal = port.iParallelPortGetIRQ(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* IRQ number of the parallel port.
*
*/
public void setIRQ(long value) {
try {
port.iParallelPortSetIRQ(_this, value);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Host parallel device name. If this parallel port is enabled, setting a
* @c null or an empty string as this attribute's value will result into
* an error.
*
*/
public String getPath() {
try {
String retVal = port.iParallelPortGetPath(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Host parallel device name. If this parallel port is enabled, setting a
* @c null or an empty string as this attribute's value will result into
* an error.
*
*/
public void setPath(String value) {
try {
port.iParallelPortSetPath(_this, value);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy