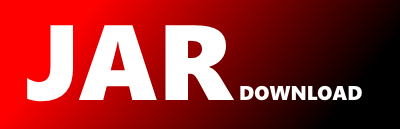
com.sun.xml.ws.commons.virtualbox.IVirtualSystemDescription Maven / Gradle / Ivy
The newest version!
package com.sun.xml.ws.commons.virtualbox;
import java.util.List;
import javax.xml.ws.Holder;
import javax.xml.ws.WebServiceException;
/**
* This interface is used in the {@link IAppliance#virtualSystemDescriptions} array.
* After {@link IAppliance#interpret} has been called, that array contains
* information about how the virtual systems described in the OVF should best be imported into VirtualBox
* virtual machines. See {@link IAppliance} for the steps required to import an OVF
* into VirtualBox.
*
*/
public class IVirtualSystemDescription
extends VBoxObject
{
public IVirtualSystemDescription(String _this, VboxPortType port) {
super(_this,port);
}
/**
* Return the number of virtual system description entries.
*
*/
public long getCount() {
try {
long retVal = port.iVirtualSystemDescriptionGetCount(_this);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* Returns information about the virtual system as arrays of instruction items. In each array, the
* items with the same indices correspond and jointly represent an import instruction for VirtualBox.
*
* The list below identifies the value sets that are possible depending on the {@link VirtualSystemDescriptionType} enum value in the array item in aTypes[]. In each case,
* the array item with the same index in aOvfValues[] will contain the original value as contained
* in the OVF file (just for informational purposes), and the corresponding item in aVboxValues[]
* will contain a suggested value to be used for VirtualBox. Depending on the description type,
* the aExtraConfigValues[] array item may also be used.- "OS": the guest operating system type. There must be exactly one such array item on import. The
* corresponding item in aVboxValues[] contains the suggested guest operating system for VirtualBox.
* This will be one of the values listed in {@link IVirtualBox#guestOSTypes}. The corresponding
* item in aOvfValues[] will contain a numerical value that described the operating system in the OVF
* (see {@link CIMOSType}).
- "Name": the name to give to the new virtual machine. There can be at most one such array item;
* if none is present on import, then an automatic name will be created from the operating system
* type. The correponding item im aOvfValues[] will contain the suggested virtual machine name
* from the OVF file, and aVboxValues[] will contain a suggestion for a unique VirtualBox {@link IMachine} name that does not exist yet.
- "Description": an arbitrary description.
- "License": the EULA section from the OVF, if present. It is the responsibility of the calling
* code to display such a license for agreement; the Main API does not enforce any such policy.
- Miscellaneous: reserved for future use.
- "CPU": the number of CPUs. There can be at most one such item, which will presently be ignored.
- "Memory": the amount of guest RAM, in bytes. There can be at most one such array item; if none
* is present on import, then VirtualBox will set a meaningful default based on the operating system
* type.
- "HarddiskControllerIDE": an IDE hard disk controller. There can be at most one such item. This
* has no value in aOvfValues[] or aVboxValues[].
* The matching item in the aRefs[] array will contain an integer that items of the "Harddisk"
* type can use to specify which hard disk controller a virtual disk should be connected to.
- "HarddiskControllerSATA": an SATA hard disk controller. There can be at most one such item. This
* has no value in aOvfValues[] or aVboxValues[].
* The matching item in the aRefs[] array will be used as with IDE controllers (see above).
- "HarddiskControllerSCSI": a SCSI hard disk controller. There can be at most one such item.
* The items in aOvfValues[] and aVboxValues[] will either be "LsiLogic" or "BusLogic".
* The matching item in the aRefs[] array will be used as with IDE controllers (see above).
- "HardDiskImage": a virtual hard disk, most probably as a reference to an image file. There can be an
* arbitrary number of these items, one for each virtual disk image that accompanies the OVF.
*
* The array item in aOvfValues[] will contain the file specification from the OVF file (without
* a path since the image file should be in the same location as the OVF file itself), whereas the
* item in aVboxValues[] will contain a qualified path specification to where VirtualBox uses the
* hard disk image. This means that on import the image will be copied and converted from the
* "ovf" location to the "vbox" location; on export, this will be handled the other way round.
* On import, the target image will also be registered with VirtualBox.
*
* The matching item in the aExtraConfigValues[] array must contain a string of the following
* format: "controller=<index>;channel=<c>"
* In this string, <index> must be an integer specifying the hard disk controller to connect
* the image to. That number must be the index of an array item with one of the hard disk controller
* types (HarddiskControllerSCSI, HarddiskControllerSATA, HarddiskControllerIDE).
* In addition, <c> must specify the channel to use on that controller. For IDE controllers,
* this can range from 0-2 (which VirtualBox will interpret as primary master, primary slave,
* secondary slave; VirtualBox reserves the secondary master for the CD-ROM drive). For SATA and
* SCSI conrollers, the channel can range from 0-29.
- "NetworkAdapter": a network adapter. The array item in aVboxValues[] will specify the hardware
* for the network adapter, whereas the array item in aExtraConfigValues[] will have a string
* of the "type=<X>" format, where <X> must be either "NAT" or "Bridged".
- "USBController": a USB controller. There can be at most one such item. If and only if such an
* item ispresent, USB support will be enabled for the new virtual machine.
- "SoundCard": a sound card. There can be at most one such item. If and only if such an item is
* present, sound support will be enabled for the new virtual machine. Note that the virtual
* machine in VirtualBox will always be presented with the standard VirtualBox soundcard, which
* may be different from the virtual soundcard expected by the appliance.
*
* @param aOvfValues
*
* @param aExtraConfigValues
*
* @param aVboxValues
*
* @param aRefs
*
* @param aTypes
*
*/
public void getDescription(Holder> aTypes, Holder> aRefs, Holder> aOvfValues, Holder> aVboxValues, Holder> aExtraConfigValues) {
try {
port.iVirtualSystemDescriptionGetDescription(_this, aTypes, aRefs, aOvfValues, aVboxValues, aExtraConfigValues);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* This is the same as {@link #getDescription} except that you can specify which types
* should be returned.
*
* @param aOvfValues
*
* @param aExtraConfigValues
*
* @param aVboxValues
*
* @param aRefs
*
* @param aType
*
* @param aTypes
*
*/
public void getDescriptionByType(VirtualSystemDescriptionType aType, Holder> aTypes, Holder> aRefs, Holder> aOvfValues, Holder> aVboxValues, Holder> aExtraConfigValues) {
try {
port.iVirtualSystemDescriptionGetDescriptionByType(_this, aType, aTypes, aRefs, aOvfValues, aVboxValues, aExtraConfigValues);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* This is the same as {@link #getDescriptionByType} except that you can specify which
* value types should be returned. See {@link VirtualSystemDescriptionValueType} for possible
* values.
*
* @param aWhich
*
* @param aType
*
* @return
*
*/
public List getValuesByType(VirtualSystemDescriptionType aType, VirtualSystemDescriptionValueType aWhich) {
try {
List retVal = port.iVirtualSystemDescriptionGetValuesByType(_this, aType, aWhich);
return retVal;
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* This method allows the appliance's user to change the configuration for the virtual
* system descriptions. For each array item returned from {@link #getDescription},
* you must pass in one boolean value and one configuration value.
*
* Each item in the boolean array determines whether the particular configuration item
* should be enabled.
* You can only disable items of the types HardDiskControllerIDE, HardDiskControllerSATA,
* HardDiskControllerSCSI, HardDiskImage, CDROM, Floppy, NetworkAdapter, USBController
* and SoundCard.
*
* For the "vbox" and "extra configuration" values, if you pass in the same arrays
* as returned in the aVboxValues and aExtraConfigValues arrays from getDescription(),
* the configuration remains unchanged. Please see the documentation for getDescription()
* for valid configuration values for the individual array item types. If the
* corresponding item in the aEnabled array is false, the configuration value is ignored.
*
* @param aEnabled
*
* @param aExtraConfigValues
*
* @param aVboxValues
*
*/
public void setFinalValues(List aEnabled, List aVboxValues, List aExtraConfigValues) {
try {
port.iVirtualSystemDescriptionSetFinalValues(_this, aEnabled, aVboxValues, aExtraConfigValues);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
/**
* This method adds an additional description entry to the stack of already
* available descriptions for this virtual system. This is handy for writing
* values which aren't directly supported by VirtualBox. One example would
* be the License type of {@link VirtualSystemDescriptionType}.
*
* @param aExtraConfigValue
*
* @param aVboxValue
*
* @param aType
*
*/
public void addDescription(VirtualSystemDescriptionType aType, String aVboxValue, String aExtraConfigValue) {
try {
port.iVirtualSystemDescriptionAddDescription(_this, aType, aVboxValue, aExtraConfigValue);
} catch (com.sun.xml.ws.commons.virtualbox.InvalidObjectFaultMsg e) {
throw new WebServiceException(e);
} catch (com.sun.xml.ws.commons.virtualbox.RuntimeFaultMsg e) {
throw new WebServiceException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy