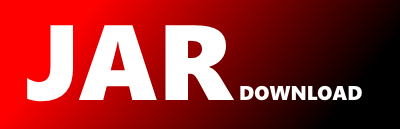
org.jvnet.hyperjaxb3.annotation.util.AnnotationUtils Maven / Gradle / Ivy
package org.jvnet.hyperjaxb3.annotation.util;
import java.lang.annotation.Annotation;
import java.lang.reflect.Array;
import org.jvnet.jaxb.annox.model.XAnnotation;
import org.jvnet.jaxb.annox.model.annotation.field.XAnnotationField;
import org.jvnet.jaxb.annox.model.annotation.field.XArrayAnnotationField;
import org.jvnet.jaxb.annox.model.annotation.field.XSingleAnnotationField;
import org.jvnet.jaxb.annox.model.annotation.value.XBooleanAnnotationValue;
import org.jvnet.jaxb.annox.model.annotation.value.XEnumAnnotationValue;
import org.jvnet.jaxb.annox.model.annotation.value.XIntAnnotationValue;
import org.jvnet.jaxb.annox.model.annotation.value.XStringAnnotationValue;
import org.jvnet.jaxb.annox.model.annotation.value.XXAnnotationAnnotationValue;
public class AnnotationUtils {
public static XAnnotationField create(
final String name, final XAnnotation value) {
if (value == null) {
return null;
} else {
return new XSingleAnnotationField(name,
value.getAnnotationClass(),
new XXAnnotationAnnotationValue(value));
}
}
public static XAnnotationField create(final String name,
final String value) {
if (value == null) {
return null;
} else {
return new XSingleAnnotationField(name, String.class,
new XStringAnnotationValue(value));
}
}
public static XAnnotationField create(final String name,
final Boolean value) {
if (value == null) {
return null;
} else {
return new XSingleAnnotationField(name, Boolean.class,
new XBooleanAnnotationValue(value));
}
}
public static XAnnotationField create(final String name,
final Integer value) {
if (value == null) {
return null;
} else {
return new XSingleAnnotationField(name, Integer.class,
new XIntAnnotationValue(value));
}
}
public static > XAnnotationField create(
final String name, final E value) {
if (value == null) {
return null;
} else {
return new XSingleAnnotationField(name, value.getClass(),
new XEnumAnnotationValue(value));
}
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static XAnnotationField create(
final String name, final XAnnotation>[] value,
Class annotationClass) {
if (value == null) {
return null;
} else {
final XXAnnotationAnnotationValue[] values = new XXAnnotationAnnotationValue[value.length];
for (int index = 0; index < value.length; index++) {
values[index] = new XXAnnotationAnnotationValue(value[index]);
}
return new XArrayAnnotationField(name, Array
.newInstance(annotationClass, 0).getClass(), values);
}
}
public static > XAnnotationField create(
final String name, final E[] value) {
if (value == null) {
return null;
} else {
@SuppressWarnings("unchecked")
final XEnumAnnotationValue[] values = new XEnumAnnotationValue[value.length];
for (int index = 0; index < value.length; index++) {
values[index] = new XEnumAnnotationValue(value[index]);
}
return new XArrayAnnotationField(name, value.getClass(), values);
}
}
public static XAnnotationField create(final String name,
final String[] value) {
if (value == null) {
return null;
} else {
final XStringAnnotationValue[] values = new XStringAnnotationValue[value.length];
for (int index = 0; index < value.length; index++) {
values[index] = new XStringAnnotationValue(value[index]);
}
return new XArrayAnnotationField(name, String[].class, values);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy