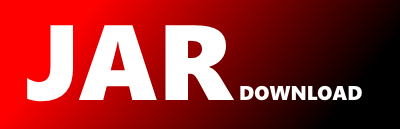
org.jvnet.hyperjaxb3.transform.TransformUtils Maven / Gradle / Ivy
package org.jvnet.hyperjaxb3.transform;
import java.util.List;
import jakarta.xml.bind.annotation.adapters.XmlAdapter;
import org.jvnet.hyperjaxb3.item.ConvertedList;
import org.jvnet.hyperjaxb3.item.Converter;
import org.jvnet.hyperjaxb3.xml.bind.annotation.adapters.XmlAdapterUtils;
public class TransformUtils {
public static boolean shouldBeWrapped(List inner) {
if (inner == null || !(inner instanceof ConvertedList)) {
return true;
} else {
return false;
}
}
public static List wrap(List inner, List outer,
Class extends XmlAdapter> xmlAdapterClass) {
if (inner == null || !(inner instanceof ConvertedList)) {
Converter converter = XmlAdapterUtils
.getConverter(xmlAdapterClass);
final List newInner = new ConvertedList(outer, converter);
if (inner != null) {
newInner.addAll(inner);
}
return newInner;
} else {
return inner;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy