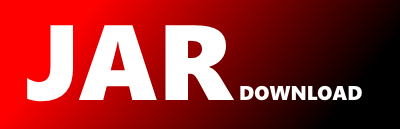
org.jvnet.jaxb.annox.parser.XAnnotationArrayAnnotationFieldParser Maven / Gradle / Ivy
The newest version!
package org.jvnet.jaxb.annox.parser;
import com.github.javaparser.ast.Node;
import com.github.javaparser.ast.expr.AnnotationExpr;
import com.github.javaparser.ast.expr.ArrayInitializerExpr;
import com.github.javaparser.ast.expr.Expression;
import java.lang.annotation.Annotation;
import java.text.MessageFormat;
import java.util.List;
import org.jvnet.jaxb.annox.annotation.NoSuchAnnotationFieldException;
import org.jvnet.jaxb.annox.javaparser.ast.visitor.AbstractGenericExpressionVisitor;
import org.jvnet.jaxb.annox.model.XAnnotation;
import org.jvnet.jaxb.annox.model.annotation.field.XAnnotationField;
import org.jvnet.jaxb.annox.model.annotation.field.XArrayAnnotationField;
import org.jvnet.jaxb.annox.model.annotation.value.XAnnotationValue;
import org.jvnet.jaxb.annox.model.annotation.value.XXAnnotationAnnotationValue;
import org.jvnet.jaxb.annox.parser.exception.AnnotationElementParseException;
import org.jvnet.jaxb.annox.parser.exception.AnnotationExpressionParseException;
import org.jvnet.jaxb.annox.util.AnnotationElementUtils;
import org.w3c.dom.Element;
public class XAnnotationArrayAnnotationFieldParser
extends XAnnotationFieldParser {
@Override
public XAnnotationField parse(Element annotationElement, String name,
Class> type) throws AnnotationElementParseException {
final Element[] elements = AnnotationElementUtils.getFieldElements(
annotationElement, name);
@SuppressWarnings("unchecked")
final XAnnotation[] xannotations = new XAnnotation[elements.length];
for (int index = 0; index < elements.length; index++) {
try {
@SuppressWarnings("unchecked")
final XAnnotation annotation = (XAnnotation) XAnnotationParser.INSTANCE
.parse(elements[index]);
xannotations[index] = annotation;
} catch (AnnotationElementParseException aepex) {
throw new AnnotationElementParseException(annotationElement,
aepex);
}
}
return construct(name, xannotations, type);
}
public XAnnotationField parse(Annotation annotation, String name,
Class> type) throws NoSuchAnnotationFieldException {
final A[] value = getAnnotationFieldValue(annotation, name);
@SuppressWarnings("unchecked")
final XAnnotation[] xannotations = new XAnnotation[value.length];
for (int index = 0; index < value.length; index++) {
@SuppressWarnings("unchecked")
final XAnnotation xannotation = (XAnnotation) XAnnotationParser.INSTANCE
.parse(value[index]);
xannotations[index] = xannotation;
}
return construct(name, xannotations, type);
}
@Override
public XAnnotationField parse(Expression expression, String name,
Class> type) throws AnnotationExpressionParseException {
final Expression[] items = expression.accept(
new AbstractGenericExpressionVisitor() {
@Override
public Expression[] visit(ArrayInitializerExpr n, Void arg) {
final List values = n.getValues();
return values.toArray(new Expression[values.size()]);
}
@Override
public Expression[] visitDefault(Expression n, Void arg) {
return new Expression[]{n};
}
@Override
public Expression[] visitDefault(Node n, Void arg) {
throw new IllegalArgumentException(
MessageFormat
.format("Unexpected expression [{0}], only array initializer expressions are expected.",
n));
}
}, null);
@SuppressWarnings("unchecked")
final XAnnotation[] xannotations = new XAnnotation[items.length];
for (int index = 0; index < items.length; index++) {
final Expression item = items[index];
final AnnotationExpr annotationExpression = item
.accept(new AbstractGenericExpressionVisitor() {
@Override
public AnnotationExpr visitDefault(Node n, Void arg) {
throw new IllegalArgumentException(
MessageFormat
.format("Unexpected expression [{0}], only annotation expressions are expected.",
n));
}
@Override
public AnnotationExpr visitDefault(AnnotationExpr n,
Void arg) {
return n;
}
}, null);
@SuppressWarnings("unchecked")
final XAnnotation annotation = (XAnnotation) XAnnotationParser.INSTANCE
.parse(annotationExpression);
xannotations[index] = annotation;
}
return construct(name, xannotations, type);
}
@SuppressWarnings("unchecked")
public XAnnotationField construct(String name, final A[] annotations,
Class> type) {
final XAnnotation[] xannotations = new XAnnotation[annotations.length];
final XAnnotationValue[] xannotationValues = new XAnnotationValue[annotations.length];
for (int index = 0; index < annotations.length; index++) {
final XAnnotation xannotation = (XAnnotation) XAnnotationParser.INSTANCE
.parse(annotations[index]);
xannotations[index] = xannotation;
xannotationValues[index] = new XXAnnotationAnnotationValue(
annotations[index], xannotations[index]);
}
return new XArrayAnnotationField(name, type, xannotationValues);
}
private XAnnotationField construct(String name,
final XAnnotation[] xannotations, Class> type) {
@SuppressWarnings("unchecked")
final XAnnotationValue[] xannotationValues = new XAnnotationValue[xannotations.length];
for (int index = 0; index < xannotations.length; index++) {
xannotationValues[index] = new XXAnnotationAnnotationValue(
xannotations[index]);
}
return new XArrayAnnotationField(name, type, xannotationValues);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy