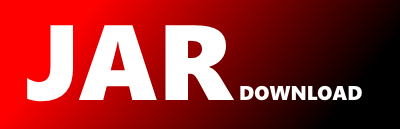
org.jvnet.jaxb.annox.parser.XGenericFieldParser Maven / Gradle / Ivy
The newest version!
package org.jvnet.jaxb.annox.parser;
import com.github.javaparser.ast.expr.Expression;
import java.lang.annotation.Annotation;
import org.jvnet.jaxb.annox.annotation.NoSuchAnnotationFieldException;
import org.jvnet.jaxb.annox.model.annotation.field.XAnnotationField;
import org.jvnet.jaxb.annox.parser.exception.AnnotationElementParseException;
import org.jvnet.jaxb.annox.parser.exception.AnnotationExpressionParseException;
import org.jvnet.jaxb.annox.parser.value.XBooleanAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XByteAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XCharAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XClassAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XDoubleAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XEnumAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XFloatAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XIntAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XLongAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XShortAnnotationValueParser;
import org.jvnet.jaxb.annox.parser.value.XStringAnnotationValueParser;
import org.w3c.dom.Element;
public class XGenericFieldParser extends XAnnotationFieldParser {
public static final XAnnotationFieldParser BOOLEAN = new XSingleAnnotationFieldParser(
new XBooleanAnnotationValueParser());
public static final XAnnotationFieldParser BYTE = new XSingleAnnotationFieldParser(
new XByteAnnotationValueParser());
public static final XAnnotationFieldParser INT = new XSingleAnnotationFieldParser(
new XIntAnnotationValueParser());
public static final XAnnotationFieldParser LONG = new XSingleAnnotationFieldParser(
new XLongAnnotationValueParser());
public static final XAnnotationFieldParser SHORT = new XSingleAnnotationFieldParser(
new XShortAnnotationValueParser());
public static final XAnnotationFieldParser CHAR = new XSingleAnnotationFieldParser(
new XCharAnnotationValueParser());
public static final XAnnotationFieldParser, Class>> CLASS = new XSingleAnnotationFieldParser, Class>>(
new XClassAnnotationValueParser());
public static final XAnnotationFieldParser DOUBLE = new XSingleAnnotationFieldParser(
new XDoubleAnnotationValueParser());
public static final XAnnotationFieldParser FLOAT = new XSingleAnnotationFieldParser(
new XFloatAnnotationValueParser());
public static final XAnnotationFieldParser, Enum>> ENUM = new XSingleAnnotationFieldParser, Enum>>(
new XEnumAnnotationValueParser());
public static final XAnnotationFieldParser STRING = new XSingleAnnotationFieldParser(
new XStringAnnotationValueParser());
@SuppressWarnings("rawtypes")
public static final XAnnotationFieldParser, ?> ANNOTATION = new XAnnotationSingleAnnotationFieldParser();
// @SuppressWarnings("rawtypes")
// public static final XAnnotationFieldParser, ?> XANNOTATION = new XXAnnotationSingleAnnotationFieldParser();
public static final XAnnotationFieldParser BOOLEAN_ARRAY = new XArrayAnnotationFieldParser(
new XBooleanAnnotationValueParser());
public static final XAnnotationFieldParser BYTE_ARRAY = new XArrayAnnotationFieldParser(
new XByteAnnotationValueParser());
public static final XAnnotationFieldParser INT_ARRAY = new XArrayAnnotationFieldParser(
new XIntAnnotationValueParser());
public static final XAnnotationFieldParser LONG_ARRAY = new XArrayAnnotationFieldParser(
new XLongAnnotationValueParser());
public static final XAnnotationFieldParser SHORT_ARRAY = new XArrayAnnotationFieldParser(
new XShortAnnotationValueParser());
public static final XAnnotationFieldParser CHAR_ARRAY = new XArrayAnnotationFieldParser(
new XCharAnnotationValueParser());
public static final XAnnotationFieldParser[], Class>[]> CLASS_ARRAY = new XArrayAnnotationFieldParser, Class>>(
new XClassAnnotationValueParser());
public static final XAnnotationFieldParser DOUBLE_ARRAY = new XArrayAnnotationFieldParser(
new XDoubleAnnotationValueParser());
public static final XAnnotationFieldParser FLOAT_ARRAY = new XArrayAnnotationFieldParser(
new XFloatAnnotationValueParser());
public static final XAnnotationFieldParser[], Enum>[]> ENUM_ARRAY = new XArrayAnnotationFieldParser, Enum>>(
new XEnumAnnotationValueParser());
public static final XAnnotationFieldParser STRING_ARRAY = new XArrayAnnotationFieldParser(
new XStringAnnotationValueParser());
// public static final XBooleanArrayAnnotationFieldParser BOOLEAN_ARRAY =
// new XBooleanArrayAnnotationFieldParser();
// public static final XByteArrayAnnotationFieldParser BYTE_ARRAY = new
// XByteArrayAnnotationFieldParser();
// public static final XIntArrayAnnotationFieldParser INT_ARRAY = new
// XIntArrayAnnotationFieldParser();
// public static final XLongArrayAnnotationFieldParser LONG_ARRAY = new
// XLongArrayAnnotationFieldParser();
// public static final XShortArrayAnnotationFieldParser SHORT_ARRAY = new
// XShortArrayAnnotationFieldParser();
// public static final XCharArrayAnnotationFieldParser CHAR_ARRAY = new
// XCharArrayAnnotationFieldParser();
// public static final XClassArrayAnnotationFieldParser CLASS_ARRAY = new
// XClassArrayAnnotationFieldParser();
// public static final XDoubleArrayAnnotationFieldParser DOUBLE_ARRAY = new
// XDoubleArrayAnnotationFieldParser();
// public static final XFloatArrayAnnotationFieldParser FLOAT_ARRAY = new
// XFloatArrayAnnotationFieldParser();
// public static final XEnumArrayAnnotationFieldParser ENUM_ARRAY = new
// XEnumArrayAnnotationFieldParser();
// public static final XStringArrayAnnotationFieldParser STRING_ARRAY = new
// XStringArrayAnnotationFieldParser();
@SuppressWarnings("rawtypes")
public static final XAnnotationArrayAnnotationFieldParser ANNOTATION_ARRAY = new XAnnotationArrayAnnotationFieldParser();
// @SuppressWarnings("rawtypes")
// public static final XXAnnotationArrayAnnotationFieldParser XANNOTATION_ARRAY = new XXAnnotationArrayAnnotationFieldParser();
@SuppressWarnings("rawtypes")
public static final XGenericFieldParser GENERIC = new XGenericFieldParser();
@Override
public XAnnotationField parse(Element element, String name, Class> type)
throws AnnotationElementParseException {
@SuppressWarnings("unchecked")
final XAnnotationFieldParser parser = (XAnnotationFieldParser) XGenericFieldParser
.detectType(type);
return parser.parse(element, name, type);
}
@Override
public XAnnotationField parse(Annotation annotation, String name,
Class> type) throws NoSuchAnnotationFieldException {
@SuppressWarnings("unchecked")
final XAnnotationFieldParser parser = (XAnnotationFieldParser) XGenericFieldParser
.detectType(type);
return parser.parse(annotation, name, type);
}
@Override
public XAnnotationField construct(String name, V value, Class> type) {
@SuppressWarnings("unchecked")
final XAnnotationFieldParser parser = (XAnnotationFieldParser) XGenericFieldParser
.detectType(type);
return parser.construct(name, value, type);
}
@Override
public XAnnotationField parse(Expression expression, String name,
Class> type) throws AnnotationExpressionParseException {
@SuppressWarnings("unchecked")
final XAnnotationFieldParser parser = (XAnnotationFieldParser) XGenericFieldParser
.detectType(type);
return parser.parse(expression, name, type);
}
public static XAnnotationFieldParser generic() {
@SuppressWarnings("unchecked")
final XAnnotationFieldParser parser = XGenericFieldParser.GENERIC;
return parser;
}
public static XAnnotationFieldParser, ?> detectType(Class> theClass) {
if (theClass == null)
throw new IllegalArgumentException("Class must not be null.");
if (theClass.isArray()) {
final XAnnotationFieldParser, ?> componentType = detectType(theClass
.getComponentType());
if (componentType == XGenericFieldParser.BOOLEAN)
return XGenericFieldParser.BOOLEAN_ARRAY;
else if (componentType == XGenericFieldParser.BYTE)
return XGenericFieldParser.BYTE_ARRAY;
else if (componentType == XGenericFieldParser.INT)
return XGenericFieldParser.INT_ARRAY;
else if (componentType == XGenericFieldParser.LONG)
return XGenericFieldParser.LONG_ARRAY;
else if (componentType == XGenericFieldParser.SHORT)
return XGenericFieldParser.SHORT_ARRAY;
else if (componentType == XGenericFieldParser.CHAR)
return XGenericFieldParser.CHAR_ARRAY;
else if (componentType == XGenericFieldParser.CLASS)
return XGenericFieldParser.CLASS_ARRAY;
else if (componentType == XGenericFieldParser.DOUBLE)
return XGenericFieldParser.DOUBLE_ARRAY;
else if (componentType == XGenericFieldParser.FLOAT)
return XGenericFieldParser.FLOAT_ARRAY;
else if (componentType == XGenericFieldParser.ENUM)
return XGenericFieldParser.ENUM_ARRAY;
else if (componentType == XGenericFieldParser.STRING)
return XGenericFieldParser.STRING_ARRAY;
else if (componentType == XGenericFieldParser.ANNOTATION)
return XGenericFieldParser.ANNOTATION_ARRAY;
// else if (componentType == XGenericFieldParser.XANNOTATION)
// return XGenericFieldParser.XANNOTATION_ARRAY;
else
throw new IllegalArgumentException(
"Unknown annotation field type.");
} else {
if (Boolean.class.equals(theClass) || Boolean.TYPE.equals(theClass)) {
return XGenericFieldParser.BOOLEAN;
} else if (Byte.class.equals(theClass)
|| Byte.TYPE.equals(theClass)) {
return XGenericFieldParser.BYTE;
} else if (Integer.class.equals(theClass)
|| Integer.TYPE.equals(theClass)) {
return XGenericFieldParser.INT;
} else if (Long.class.equals(theClass)
|| Long.TYPE.equals(theClass)) {
return XGenericFieldParser.LONG;
} else if (Short.class.equals(theClass)
|| Short.TYPE.equals(theClass)) {
return XGenericFieldParser.SHORT;
} else if (Character.class.equals(theClass)
|| Character.TYPE.equals(theClass)) {
return XGenericFieldParser.CHAR;
} else if (Double.class.equals(theClass)
|| Double.TYPE.equals(theClass)) {
return XGenericFieldParser.DOUBLE;
} else if (Float.class.equals(theClass)
|| Float.TYPE.equals(theClass)) {
return XGenericFieldParser.FLOAT;
} else if (Class.class.equals(theClass)) {
return XGenericFieldParser.CLASS;
} else if (String.class.equals(theClass)) {
return XGenericFieldParser.STRING;
} else if (Enum.class.isAssignableFrom(theClass)) {
return XGenericFieldParser.ENUM;
} else if (Annotation.class.isAssignableFrom(theClass)) {
return XGenericFieldParser.ANNOTATION;
// } else if (XAnnotation.class.isAssignableFrom(theClass)) {
// return XGenericFieldParser.XANNOTATION;
} else {
throw new IllegalArgumentException(
"Unknown annotation field type [" + theClass.getName()
+ "].");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy