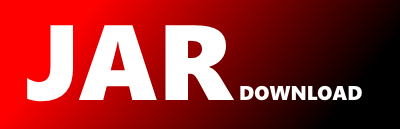
org.jvnet.jaxbcommons.lang.impl.DefaultClonedObjects Maven / Gradle / Ivy
package org.jvnet.jaxbcommons.lang.impl;
import org.jvnet.jaxbcommons.lang.ClonedObjects;
public class DefaultClonedObjects implements ClonedObjects {
private int size;
private transient Entry[] elementData;
private int cursor;
public DefaultClonedObjects(int initialCapacity) {
if (initialCapacity < 0)
throw new IllegalArgumentException("Illegal Capacity: " + initialCapacity);
this.elementData = new Entry[initialCapacity];
}
public DefaultClonedObjects() {
this(10);
}
public boolean isCloned(Object object) {
for (int i = 0; i < size; i++) {
if (object == elementData[i].getKey()) {
cursor = i;
return true;
}
}
return false;
}
public void addCloned(Object object, Object clone) {
ensureCapacity(size + 1);
elementData[size++] = new Entry(object, clone);
}
public Object getClone(Object object) {
if (size == 0) {
return null;
}
else {
int cursor = this.cursor;
if (cursor < 0) {
cursor = 0;
}
if (cursor > size - 1) {
cursor = size - 1;
}
for (int index = cursor; index < size; index++) {
final Entry entry = elementData[index];
if (object == entry.getKey()) {
this.cursor = cursor;
return entry.getValue();
}
}
for (int index = 0; index < cursor - 1; index++) {
final Entry entry = elementData[index];
if (object == entry.getKey()) {
this.cursor = cursor;
return entry.getValue();
}
}
return null;
}
}
private void ensureCapacity(int minCapacity) {
int oldCapacity = elementData.length;
if (minCapacity > oldCapacity) {
Object oldData[] = elementData;
int newCapacity = (oldCapacity * 3) / 2 + 1;
if (newCapacity < minCapacity)
newCapacity = minCapacity;
elementData = new Entry[newCapacity];
System.arraycopy(oldData, 0, elementData, 0, size);
}
}
private static class Entry {
private final Object key;
private final Object value;
public Entry(final Object key, final Object value) {
this.key = key;
this.value = value;
}
public Object getKey() {
return key;
}
public Object getValue() {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy