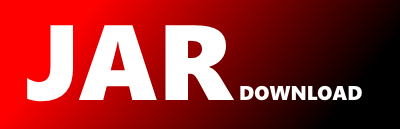
org.jvnet.jaxbcommons.tree.Node Maven / Gradle / Ivy
package org.jvnet.jaxbcommons.tree;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
public class Node {
private String name;
private Class type;
private Object value;
private Node[] children;
public Node(String name, Class type, Object value, Node[] children) {
this.name = name;
this.type = type;
this.value = value;
this.children = children;
}
public Node(String name, Class type, Object value, Node[][] children) {
this(name, type, value, concat(children));
}
public Node[] getChildren() {
return children;
}
public String getName() {
return name;
}
public Class getType() {
return type;
}
public Object getValue() {
return value;
}
public static Node[] concat(Node[][] nodeArrays) {
final List list = new ArrayList();
for (int index = 0; index < nodeArrays.length; index++) {
final Node[] nodes = nodeArrays[index];
for (int jndex = 0; jndex < nodes.length; jndex++) {
final Node node = nodes[jndex];
list.add(node);
}
}
return toArray(list);
}
public static Node[] toArray(Collection collection) {
if (collection == null) {
return null;
}
else {
return (Node[]) collection.toArray(new Node[collection.size()]);
}
}
public Object visit(NodeVisitor nodeVisitor){
return nodeVisitor.visitNode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy