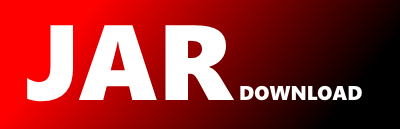
org.jvnet.jaxbcommons.addon.tests.AbstractAddOnTest Maven / Gradle / Ivy
package org.jvnet.jaxbcommons.addon.tests;
import java.io.File;
import java.io.FileFilter;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import junit.framework.TestCase;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.filefilter.SuffixFileFilter;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import com.sun.tools.xjc.Driver;
public abstract class AbstractAddOnTest extends TestCase {
public List getAddonOptions() {
final List addonOptions = new ArrayList();
return addonOptions;
}
protected Log log = LogFactory.getLog(getClass());
/**
* Returns command-line arguments for XJC invocation. This array will be
* constructed of what {@link #getOptions()},{@link #getBindingOptions()},
* {@link #getAddonOptions()}and {@link #getSchemaOptions()}return.
*
* @return Command-line arguments for XJC invocation.
*/
public String[] getArguments() {
final List args = new ArrayList();
args.addAll(getOptions());
args.addAll(getBindingOptions());
if (isDebug())
args.add("-debug");
if (isNv())
args.add("-nv");
if (isExtension())
args.add("-extension");
args.addAll(getAddonOptions());
args.addAll(getSchemaOptions());
return (String[]) args.toArray(new String[] {});
}
/**
* Returns command-line options for the add-on.
*
* @return Command line options for the add-on.
*/
public List getOptions() {
return Arrays.asList(new String[] { "-d",
getGeneratedSourcesDir().getAbsolutePath() });
}
protected boolean isDebug() {
return true;
}
protected boolean isNv() {
return true;
}
protected boolean isExtension() {
return true;
}
/**
* Returns schema options.
*
* @return Schema options.
*/
public List getSchemaOptions() {
final File[] schemaFiles = getSchemaFiles();
final List schemaOptions = new ArrayList(schemaFiles.length);
for (int index = 0; index < schemaFiles.length; index++) {
final File schemaFile = schemaFiles[index];
schemaOptions.add(schemaFile.getAbsolutePath());
}
return schemaOptions;
}
/**
* Returns schema directory.
*
* @return Schema directory.
*/
public File getSchemaDirectory() {
return new File(getBaseDir(), "src/main/resources");
}
/**
* Returns an array of schema files.
*
* @return Array of schema files.
*/
public File[] getSchemaFiles() {
final Collection schemaFiles = FileUtils.listFiles(
getSchemaDirectory(), new String[] { "xsd" }, true);
return (File[]) schemaFiles.toArray(new File[schemaFiles.size()]);
}
/**
* Returns binding directory.
*
* @return Binding directory.
*/
public File getBindingDirectory() {
return new File(getBaseDir(), "src/main/resources");
}
/**
* Returns an array of binding files.
*
* @return An array of binding files.
*/
public File[] getBindingFiles() {
final FileFilter fileFilter = new SuffixFileFilter(".xjb");
return getBindingDirectory().listFiles(fileFilter);
}
/**
* Binding options.
*
* @return Binding options.
*/
public List getBindingOptions() {
final File[] bindingFiles = getBindingFiles();
final List bindingOptions = new ArrayList();
if (null != bindingFiles && bindingFiles.length > 0) {
bindingOptions.add("-b");
for (int index = 0; index < bindingFiles.length; index++) {
final File bindingFile = bindingFiles[index];
bindingOptions.add(bindingFile.getAbsolutePath());
}
}
return bindingOptions;
}
/**
* Directory where the sources will be generated.
*
* @return "Generated sources" directory.
*/
public File getGeneratedSourcesDir() {
final File generatedSourcesDir = new File(getBaseDir(),
"target/generated-sources/xjc");
if (!generatedSourcesDir.exists()) {
generatedSourcesDir.mkdirs();
}
return generatedSourcesDir;
}
/**
* Runs the test.
*
* @throws Exception
* In case a problem occurs during running the test.
*/
public void testRun() throws Exception {
try {
Driver.run(getArguments(), System.out, System.err);
} catch (Exception ex) {
ex.printStackTrace();
throw ex;
}
}
public File getBaseDir() {
try {
final URI base = new URI(getClass().getProtectionDomain()
.getCodeSource().getLocation().toString());
return (new File(base)).getParentFile().getParentFile()
.getAbsoluteFile();
} catch (Exception ex) {
throw new AssertionError(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy