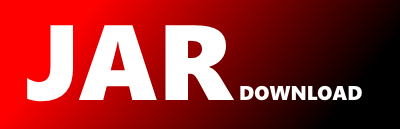
org.jvnet.jaxbcommons.tests.AbstractSamplesTest Maven / Gradle / Ivy
package org.jvnet.jaxbcommons.tests;
import java.io.File;
import java.util.Collection;
import javax.xml.bind.JAXBContext;
import junit.framework.TestCase;
import org.apache.commons.io.FileUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
public abstract class AbstractSamplesTest extends TestCase {
protected Log logger = LogFactory.getLog(getClass());
protected abstract String getContextPath();
protected abstract void checkSample(JAXBContext context, File sample)
throws Exception;
protected void checkSample(File sample) throws Exception {
logger.debug("Checking sample [" + sample.getName() + "].");
final JAXBContext context = createContext();
checkSample(context, sample);
}
public void testSamples() throws Exception {
logger.debug("Testing samples.");
final File[] sampleFiles = getSampleFiles();
for (int index = 0; index < sampleFiles.length; index++) {
final File sampleFile = sampleFiles[index];
checkSample(sampleFile);
}
logger.debug("Finished testing samples.");
}
protected File getBaseDir() {
try {
return (new File(getClass().getProtectionDomain().getCodeSource()
.getLocation().getFile())).getParentFile().getParentFile()
.getAbsoluteFile();
} catch (Exception ex) {
throw new AssertionError(ex);
}
}
protected File getSamplesDirectory() {
return new File(getBaseDir(), getSamplesDirectoryName());
}
public static final String DEFAULT_SAMPLES_DIRECTORY_NAME = "src/test/samples";
protected String getSamplesDirectoryName() {
return DEFAULT_SAMPLES_DIRECTORY_NAME;
}
protected File[] getSampleFiles() {
final Collection files = FileUtils.listFiles(getSamplesDirectory(),
new String[] { "xml" }, true);
return (File[]) files.toArray(new File[files.size()]);
}
protected JAXBContext createContext() throws Exception {
return JAXBContext.newInstance(getContextPath());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy