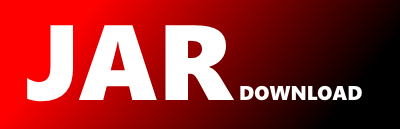
WMS_1_1_0.BoundingBox Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.10.31 at 02:43:57 PM CET
//
package WMS_1_1_0;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.CopyStrategy;
import org.jvnet.jaxb2_commons.lang.CopyTo;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBMergeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.MergeFrom;
import org.jvnet.jaxb2_commons.lang.MergeStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
@XmlRootElement(name = "BoundingBox")
public class BoundingBox
implements Cloneable, CopyTo, Equals, HashCode, MergeFrom, ToString
{
@XmlAttribute(name = "SRS", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String srs;
@XmlAttribute(name = "minx", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String minx;
@XmlAttribute(name = "miny", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String miny;
@XmlAttribute(name = "maxx", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String maxx;
@XmlAttribute(name = "maxy", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String maxy;
@XmlAttribute(name = "resx")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String resx;
@XmlAttribute(name = "resy")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
protected String resy;
/**
* Gets the value of the srs property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSRS() {
return srs;
}
/**
* Sets the value of the srs property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSRS(String value) {
this.srs = value;
}
/**
* Gets the value of the minx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMinx() {
return minx;
}
/**
* Sets the value of the minx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMinx(String value) {
this.minx = value;
}
/**
* Gets the value of the miny property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMiny() {
return miny;
}
/**
* Sets the value of the miny property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMiny(String value) {
this.miny = value;
}
/**
* Gets the value of the maxx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaxx() {
return maxx;
}
/**
* Sets the value of the maxx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaxx(String value) {
this.maxx = value;
}
/**
* Gets the value of the maxy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaxy() {
return maxy;
}
/**
* Sets the value of the maxy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaxy(String value) {
this.maxy = value;
}
/**
* Gets the value of the resx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResx() {
return resx;
}
/**
* Sets the value of the resx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResx(String value) {
this.resx = value;
}
/**
* Gets the value of the resy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResy() {
return resy;
}
/**
* Sets the value of the resy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResy(String value) {
this.resy = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theSRS;
theSRS = this.getSRS();
strategy.appendField(locator, this, "srs", buffer, theSRS);
}
{
String theMinx;
theMinx = this.getMinx();
strategy.appendField(locator, this, "minx", buffer, theMinx);
}
{
String theMiny;
theMiny = this.getMiny();
strategy.appendField(locator, this, "miny", buffer, theMiny);
}
{
String theMaxx;
theMaxx = this.getMaxx();
strategy.appendField(locator, this, "maxx", buffer, theMaxx);
}
{
String theMaxy;
theMaxy = this.getMaxy();
strategy.appendField(locator, this, "maxy", buffer, theMaxy);
}
{
String theResx;
theResx = this.getResx();
strategy.appendField(locator, this, "resx", buffer, theResx);
}
{
String theResy;
theResy = this.getResy();
strategy.appendField(locator, this, "resy", buffer, theResy);
}
return buffer;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof BoundingBox)) {
return false;
}
if (this == object) {
return true;
}
final BoundingBox that = ((BoundingBox) object);
{
String lhsSRS;
lhsSRS = this.getSRS();
String rhsSRS;
rhsSRS = that.getSRS();
if (!strategy.equals(LocatorUtils.property(thisLocator, "srs", lhsSRS), LocatorUtils.property(thatLocator, "srs", rhsSRS), lhsSRS, rhsSRS)) {
return false;
}
}
{
String lhsMinx;
lhsMinx = this.getMinx();
String rhsMinx;
rhsMinx = that.getMinx();
if (!strategy.equals(LocatorUtils.property(thisLocator, "minx", lhsMinx), LocatorUtils.property(thatLocator, "minx", rhsMinx), lhsMinx, rhsMinx)) {
return false;
}
}
{
String lhsMiny;
lhsMiny = this.getMiny();
String rhsMiny;
rhsMiny = that.getMiny();
if (!strategy.equals(LocatorUtils.property(thisLocator, "miny", lhsMiny), LocatorUtils.property(thatLocator, "miny", rhsMiny), lhsMiny, rhsMiny)) {
return false;
}
}
{
String lhsMaxx;
lhsMaxx = this.getMaxx();
String rhsMaxx;
rhsMaxx = that.getMaxx();
if (!strategy.equals(LocatorUtils.property(thisLocator, "maxx", lhsMaxx), LocatorUtils.property(thatLocator, "maxx", rhsMaxx), lhsMaxx, rhsMaxx)) {
return false;
}
}
{
String lhsMaxy;
lhsMaxy = this.getMaxy();
String rhsMaxy;
rhsMaxy = that.getMaxy();
if (!strategy.equals(LocatorUtils.property(thisLocator, "maxy", lhsMaxy), LocatorUtils.property(thatLocator, "maxy", rhsMaxy), lhsMaxy, rhsMaxy)) {
return false;
}
}
{
String lhsResx;
lhsResx = this.getResx();
String rhsResx;
rhsResx = that.getResx();
if (!strategy.equals(LocatorUtils.property(thisLocator, "resx", lhsResx), LocatorUtils.property(thatLocator, "resx", rhsResx), lhsResx, rhsResx)) {
return false;
}
}
{
String lhsResy;
lhsResy = this.getResy();
String rhsResy;
rhsResy = that.getResy();
if (!strategy.equals(LocatorUtils.property(thisLocator, "resy", lhsResy), LocatorUtils.property(thatLocator, "resy", rhsResy), lhsResy, rhsResy)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theSRS;
theSRS = this.getSRS();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "srs", theSRS), currentHashCode, theSRS);
}
{
String theMinx;
theMinx = this.getMinx();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "minx", theMinx), currentHashCode, theMinx);
}
{
String theMiny;
theMiny = this.getMiny();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "miny", theMiny), currentHashCode, theMiny);
}
{
String theMaxx;
theMaxx = this.getMaxx();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "maxx", theMaxx), currentHashCode, theMaxx);
}
{
String theMaxy;
theMaxy = this.getMaxy();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "maxy", theMaxy), currentHashCode, theMaxy);
}
{
String theResx;
theResx = this.getResx();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "resx", theResx), currentHashCode, theResx);
}
{
String theResy;
theResy = this.getResy();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "resy", theResy), currentHashCode, theResy);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof BoundingBox) {
final BoundingBox copy = ((BoundingBox) draftCopy);
if (this.srs!= null) {
String sourceSRS;
sourceSRS = this.getSRS();
String copySRS = ((String) strategy.copy(LocatorUtils.property(locator, "srs", sourceSRS), sourceSRS));
copy.setSRS(copySRS);
} else {
copy.srs = null;
}
if (this.minx!= null) {
String sourceMinx;
sourceMinx = this.getMinx();
String copyMinx = ((String) strategy.copy(LocatorUtils.property(locator, "minx", sourceMinx), sourceMinx));
copy.setMinx(copyMinx);
} else {
copy.minx = null;
}
if (this.miny!= null) {
String sourceMiny;
sourceMiny = this.getMiny();
String copyMiny = ((String) strategy.copy(LocatorUtils.property(locator, "miny", sourceMiny), sourceMiny));
copy.setMiny(copyMiny);
} else {
copy.miny = null;
}
if (this.maxx!= null) {
String sourceMaxx;
sourceMaxx = this.getMaxx();
String copyMaxx = ((String) strategy.copy(LocatorUtils.property(locator, "maxx", sourceMaxx), sourceMaxx));
copy.setMaxx(copyMaxx);
} else {
copy.maxx = null;
}
if (this.maxy!= null) {
String sourceMaxy;
sourceMaxy = this.getMaxy();
String copyMaxy = ((String) strategy.copy(LocatorUtils.property(locator, "maxy", sourceMaxy), sourceMaxy));
copy.setMaxy(copyMaxy);
} else {
copy.maxy = null;
}
if (this.resx!= null) {
String sourceResx;
sourceResx = this.getResx();
String copyResx = ((String) strategy.copy(LocatorUtils.property(locator, "resx", sourceResx), sourceResx));
copy.setResx(copyResx);
} else {
copy.resx = null;
}
if (this.resy!= null) {
String sourceResy;
sourceResy = this.getResy();
String copyResy = ((String) strategy.copy(LocatorUtils.property(locator, "resy", sourceResy), sourceResy));
copy.setResy(copyResy);
} else {
copy.resy = null;
}
}
return draftCopy;
}
public Object createNewInstance() {
return new BoundingBox();
}
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.INSTANCE;
mergeFrom(null, null, left, right, strategy);
}
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof BoundingBox) {
final BoundingBox target = this;
final BoundingBox leftObject = ((BoundingBox) left);
final BoundingBox rightObject = ((BoundingBox) right);
{
String lhsSRS;
lhsSRS = leftObject.getSRS();
String rhsSRS;
rhsSRS = rightObject.getSRS();
String mergedSRS = ((String) strategy.merge(LocatorUtils.property(leftLocator, "srs", lhsSRS), LocatorUtils.property(rightLocator, "srs", rhsSRS), lhsSRS, rhsSRS));
target.setSRS(mergedSRS);
}
{
String lhsMinx;
lhsMinx = leftObject.getMinx();
String rhsMinx;
rhsMinx = rightObject.getMinx();
String mergedMinx = ((String) strategy.merge(LocatorUtils.property(leftLocator, "minx", lhsMinx), LocatorUtils.property(rightLocator, "minx", rhsMinx), lhsMinx, rhsMinx));
target.setMinx(mergedMinx);
}
{
String lhsMiny;
lhsMiny = leftObject.getMiny();
String rhsMiny;
rhsMiny = rightObject.getMiny();
String mergedMiny = ((String) strategy.merge(LocatorUtils.property(leftLocator, "miny", lhsMiny), LocatorUtils.property(rightLocator, "miny", rhsMiny), lhsMiny, rhsMiny));
target.setMiny(mergedMiny);
}
{
String lhsMaxx;
lhsMaxx = leftObject.getMaxx();
String rhsMaxx;
rhsMaxx = rightObject.getMaxx();
String mergedMaxx = ((String) strategy.merge(LocatorUtils.property(leftLocator, "maxx", lhsMaxx), LocatorUtils.property(rightLocator, "maxx", rhsMaxx), lhsMaxx, rhsMaxx));
target.setMaxx(mergedMaxx);
}
{
String lhsMaxy;
lhsMaxy = leftObject.getMaxy();
String rhsMaxy;
rhsMaxy = rightObject.getMaxy();
String mergedMaxy = ((String) strategy.merge(LocatorUtils.property(leftLocator, "maxy", lhsMaxy), LocatorUtils.property(rightLocator, "maxy", rhsMaxy), lhsMaxy, rhsMaxy));
target.setMaxy(mergedMaxy);
}
{
String lhsResx;
lhsResx = leftObject.getResx();
String rhsResx;
rhsResx = rightObject.getResx();
String mergedResx = ((String) strategy.merge(LocatorUtils.property(leftLocator, "resx", lhsResx), LocatorUtils.property(rightLocator, "resx", rhsResx), lhsResx, rhsResx));
target.setResx(mergedResx);
}
{
String lhsResy;
lhsResy = leftObject.getResy();
String rhsResy;
rhsResy = rightObject.getResy();
String mergedResy = ((String) strategy.merge(LocatorUtils.property(leftLocator, "resy", lhsResy), LocatorUtils.property(rightLocator, "resy", rhsResy), lhsResy, rhsResy));
target.setResy(mergedResy);
}
}
}
public BoundingBox withSRS(String value) {
setSRS(value);
return this;
}
public BoundingBox withMinx(String value) {
setMinx(value);
return this;
}
public BoundingBox withMiny(String value) {
setMiny(value);
return this;
}
public BoundingBox withMaxx(String value) {
setMaxx(value);
return this;
}
public BoundingBox withMaxy(String value) {
setMaxy(value);
return this;
}
public BoundingBox withResx(String value) {
setResx(value);
return this;
}
public BoundingBox withResy(String value) {
setResy(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy