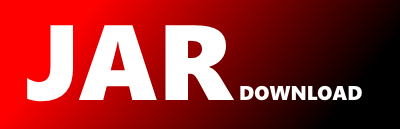
WMS_1_1_0.Request Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.10.31 at 02:43:57 PM CET
//
package WMS_1_1_0;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.CopyStrategy;
import org.jvnet.jaxb2_commons.lang.CopyTo;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBMergeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.MergeFrom;
import org.jvnet.jaxb2_commons.lang.MergeStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"getCapabilities",
"getMap",
"getFeatureInfo",
"describeLayer"
})
@XmlRootElement(name = "Request")
public class Request
implements Cloneable, CopyTo, Equals, HashCode, MergeFrom, ToString
{
@XmlElement(name = "GetCapabilities", required = true)
protected GetCapabilities getCapabilities;
@XmlElement(name = "GetMap", required = true)
protected GetMap getMap;
@XmlElement(name = "GetFeatureInfo")
protected GetFeatureInfo getFeatureInfo;
@XmlElement(name = "DescribeLayer")
protected DescribeLayer describeLayer;
/**
* Gets the value of the getCapabilities property.
*
* @return
* possible object is
* {@link GetCapabilities }
*
*/
public GetCapabilities getGetCapabilities() {
return getCapabilities;
}
/**
* Sets the value of the getCapabilities property.
*
* @param value
* allowed object is
* {@link GetCapabilities }
*
*/
public void setGetCapabilities(GetCapabilities value) {
this.getCapabilities = value;
}
/**
* Gets the value of the getMap property.
*
* @return
* possible object is
* {@link GetMap }
*
*/
public GetMap getGetMap() {
return getMap;
}
/**
* Sets the value of the getMap property.
*
* @param value
* allowed object is
* {@link GetMap }
*
*/
public void setGetMap(GetMap value) {
this.getMap = value;
}
/**
* Gets the value of the getFeatureInfo property.
*
* @return
* possible object is
* {@link GetFeatureInfo }
*
*/
public GetFeatureInfo getGetFeatureInfo() {
return getFeatureInfo;
}
/**
* Sets the value of the getFeatureInfo property.
*
* @param value
* allowed object is
* {@link GetFeatureInfo }
*
*/
public void setGetFeatureInfo(GetFeatureInfo value) {
this.getFeatureInfo = value;
}
/**
* Gets the value of the describeLayer property.
*
* @return
* possible object is
* {@link DescribeLayer }
*
*/
public DescribeLayer getDescribeLayer() {
return describeLayer;
}
/**
* Sets the value of the describeLayer property.
*
* @param value
* allowed object is
* {@link DescribeLayer }
*
*/
public void setDescribeLayer(DescribeLayer value) {
this.describeLayer = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
GetCapabilities theGetCapabilities;
theGetCapabilities = this.getGetCapabilities();
strategy.appendField(locator, this, "getCapabilities", buffer, theGetCapabilities);
}
{
GetMap theGetMap;
theGetMap = this.getGetMap();
strategy.appendField(locator, this, "getMap", buffer, theGetMap);
}
{
GetFeatureInfo theGetFeatureInfo;
theGetFeatureInfo = this.getGetFeatureInfo();
strategy.appendField(locator, this, "getFeatureInfo", buffer, theGetFeatureInfo);
}
{
DescribeLayer theDescribeLayer;
theDescribeLayer = this.getDescribeLayer();
strategy.appendField(locator, this, "describeLayer", buffer, theDescribeLayer);
}
return buffer;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Request)) {
return false;
}
if (this == object) {
return true;
}
final Request that = ((Request) object);
{
GetCapabilities lhsGetCapabilities;
lhsGetCapabilities = this.getGetCapabilities();
GetCapabilities rhsGetCapabilities;
rhsGetCapabilities = that.getGetCapabilities();
if (!strategy.equals(LocatorUtils.property(thisLocator, "getCapabilities", lhsGetCapabilities), LocatorUtils.property(thatLocator, "getCapabilities", rhsGetCapabilities), lhsGetCapabilities, rhsGetCapabilities)) {
return false;
}
}
{
GetMap lhsGetMap;
lhsGetMap = this.getGetMap();
GetMap rhsGetMap;
rhsGetMap = that.getGetMap();
if (!strategy.equals(LocatorUtils.property(thisLocator, "getMap", lhsGetMap), LocatorUtils.property(thatLocator, "getMap", rhsGetMap), lhsGetMap, rhsGetMap)) {
return false;
}
}
{
GetFeatureInfo lhsGetFeatureInfo;
lhsGetFeatureInfo = this.getGetFeatureInfo();
GetFeatureInfo rhsGetFeatureInfo;
rhsGetFeatureInfo = that.getGetFeatureInfo();
if (!strategy.equals(LocatorUtils.property(thisLocator, "getFeatureInfo", lhsGetFeatureInfo), LocatorUtils.property(thatLocator, "getFeatureInfo", rhsGetFeatureInfo), lhsGetFeatureInfo, rhsGetFeatureInfo)) {
return false;
}
}
{
DescribeLayer lhsDescribeLayer;
lhsDescribeLayer = this.getDescribeLayer();
DescribeLayer rhsDescribeLayer;
rhsDescribeLayer = that.getDescribeLayer();
if (!strategy.equals(LocatorUtils.property(thisLocator, "describeLayer", lhsDescribeLayer), LocatorUtils.property(thatLocator, "describeLayer", rhsDescribeLayer), lhsDescribeLayer, rhsDescribeLayer)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
GetCapabilities theGetCapabilities;
theGetCapabilities = this.getGetCapabilities();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "getCapabilities", theGetCapabilities), currentHashCode, theGetCapabilities);
}
{
GetMap theGetMap;
theGetMap = this.getGetMap();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "getMap", theGetMap), currentHashCode, theGetMap);
}
{
GetFeatureInfo theGetFeatureInfo;
theGetFeatureInfo = this.getGetFeatureInfo();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "getFeatureInfo", theGetFeatureInfo), currentHashCode, theGetFeatureInfo);
}
{
DescribeLayer theDescribeLayer;
theDescribeLayer = this.getDescribeLayer();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "describeLayer", theDescribeLayer), currentHashCode, theDescribeLayer);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof Request) {
final Request copy = ((Request) draftCopy);
if (this.getCapabilities!= null) {
GetCapabilities sourceGetCapabilities;
sourceGetCapabilities = this.getGetCapabilities();
GetCapabilities copyGetCapabilities = ((GetCapabilities) strategy.copy(LocatorUtils.property(locator, "getCapabilities", sourceGetCapabilities), sourceGetCapabilities));
copy.setGetCapabilities(copyGetCapabilities);
} else {
copy.getCapabilities = null;
}
if (this.getMap!= null) {
GetMap sourceGetMap;
sourceGetMap = this.getGetMap();
GetMap copyGetMap = ((GetMap) strategy.copy(LocatorUtils.property(locator, "getMap", sourceGetMap), sourceGetMap));
copy.setGetMap(copyGetMap);
} else {
copy.getMap = null;
}
if (this.getFeatureInfo!= null) {
GetFeatureInfo sourceGetFeatureInfo;
sourceGetFeatureInfo = this.getGetFeatureInfo();
GetFeatureInfo copyGetFeatureInfo = ((GetFeatureInfo) strategy.copy(LocatorUtils.property(locator, "getFeatureInfo", sourceGetFeatureInfo), sourceGetFeatureInfo));
copy.setGetFeatureInfo(copyGetFeatureInfo);
} else {
copy.getFeatureInfo = null;
}
if (this.describeLayer!= null) {
DescribeLayer sourceDescribeLayer;
sourceDescribeLayer = this.getDescribeLayer();
DescribeLayer copyDescribeLayer = ((DescribeLayer) strategy.copy(LocatorUtils.property(locator, "describeLayer", sourceDescribeLayer), sourceDescribeLayer));
copy.setDescribeLayer(copyDescribeLayer);
} else {
copy.describeLayer = null;
}
}
return draftCopy;
}
public Object createNewInstance() {
return new Request();
}
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.INSTANCE;
mergeFrom(null, null, left, right, strategy);
}
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Request) {
final Request target = this;
final Request leftObject = ((Request) left);
final Request rightObject = ((Request) right);
{
GetCapabilities lhsGetCapabilities;
lhsGetCapabilities = leftObject.getGetCapabilities();
GetCapabilities rhsGetCapabilities;
rhsGetCapabilities = rightObject.getGetCapabilities();
GetCapabilities mergedGetCapabilities = ((GetCapabilities) strategy.merge(LocatorUtils.property(leftLocator, "getCapabilities", lhsGetCapabilities), LocatorUtils.property(rightLocator, "getCapabilities", rhsGetCapabilities), lhsGetCapabilities, rhsGetCapabilities));
target.setGetCapabilities(mergedGetCapabilities);
}
{
GetMap lhsGetMap;
lhsGetMap = leftObject.getGetMap();
GetMap rhsGetMap;
rhsGetMap = rightObject.getGetMap();
GetMap mergedGetMap = ((GetMap) strategy.merge(LocatorUtils.property(leftLocator, "getMap", lhsGetMap), LocatorUtils.property(rightLocator, "getMap", rhsGetMap), lhsGetMap, rhsGetMap));
target.setGetMap(mergedGetMap);
}
{
GetFeatureInfo lhsGetFeatureInfo;
lhsGetFeatureInfo = leftObject.getGetFeatureInfo();
GetFeatureInfo rhsGetFeatureInfo;
rhsGetFeatureInfo = rightObject.getGetFeatureInfo();
GetFeatureInfo mergedGetFeatureInfo = ((GetFeatureInfo) strategy.merge(LocatorUtils.property(leftLocator, "getFeatureInfo", lhsGetFeatureInfo), LocatorUtils.property(rightLocator, "getFeatureInfo", rhsGetFeatureInfo), lhsGetFeatureInfo, rhsGetFeatureInfo));
target.setGetFeatureInfo(mergedGetFeatureInfo);
}
{
DescribeLayer lhsDescribeLayer;
lhsDescribeLayer = leftObject.getDescribeLayer();
DescribeLayer rhsDescribeLayer;
rhsDescribeLayer = rightObject.getDescribeLayer();
DescribeLayer mergedDescribeLayer = ((DescribeLayer) strategy.merge(LocatorUtils.property(leftLocator, "describeLayer", lhsDescribeLayer), LocatorUtils.property(rightLocator, "describeLayer", rhsDescribeLayer), lhsDescribeLayer, rhsDescribeLayer));
target.setDescribeLayer(mergedDescribeLayer);
}
}
}
public Request withGetCapabilities(GetCapabilities value) {
setGetCapabilities(value);
return this;
}
public Request withGetMap(GetMap value) {
setGetMap(value);
return this;
}
public Request withGetFeatureInfo(GetFeatureInfo value) {
setGetFeatureInfo(value);
return this;
}
public Request withDescribeLayer(DescribeLayer value) {
setDescribeLayer(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy