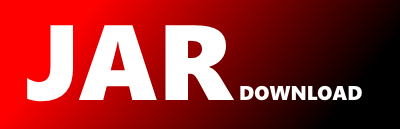
WMS_1_1_0.Style Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.10.31 at 02:43:57 PM CET
//
package WMS_1_1_0;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.CopyStrategy;
import org.jvnet.jaxb2_commons.lang.CopyTo;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBMergeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.MergeFrom;
import org.jvnet.jaxb2_commons.lang.MergeStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"name",
"title",
"_abstract",
"legendURL",
"styleSheetURL",
"styleURL"
})
@XmlRootElement(name = "Style")
public class Style
implements Cloneable, CopyTo, Equals, HashCode, MergeFrom, ToString
{
@XmlElement(name = "Name", required = true)
protected String name;
@XmlElement(name = "Title", required = true)
protected String title;
@XmlElement(name = "Abstract")
protected String _abstract;
@XmlElement(name = "LegendURL")
protected List legendURL;
@XmlElement(name = "StyleSheetURL")
protected StyleSheetURL styleSheetURL;
@XmlElement(name = "StyleURL")
protected StyleURL styleURL;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the abstract property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAbstract() {
return _abstract;
}
/**
* Sets the value of the abstract property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAbstract(String value) {
this._abstract = value;
}
/**
* Gets the value of the legendURL property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the legendURL property.
*
*
* For example, to add a new item, do as follows:
*
* getLegendURL().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LegendURL }
*
*
*/
public List getLegendURL() {
if (legendURL == null) {
legendURL = new ArrayList();
}
return this.legendURL;
}
/**
* Gets the value of the styleSheetURL property.
*
* @return
* possible object is
* {@link StyleSheetURL }
*
*/
public StyleSheetURL getStyleSheetURL() {
return styleSheetURL;
}
/**
* Sets the value of the styleSheetURL property.
*
* @param value
* allowed object is
* {@link StyleSheetURL }
*
*/
public void setStyleSheetURL(StyleSheetURL value) {
this.styleSheetURL = value;
}
/**
* Gets the value of the styleURL property.
*
* @return
* possible object is
* {@link StyleURL }
*
*/
public StyleURL getStyleURL() {
return styleURL;
}
/**
* Sets the value of the styleURL property.
*
* @param value
* allowed object is
* {@link StyleURL }
*
*/
public void setStyleURL(StyleURL value) {
this.styleURL = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName);
}
{
String theTitle;
theTitle = this.getTitle();
strategy.appendField(locator, this, "title", buffer, theTitle);
}
{
String theAbstract;
theAbstract = this.getAbstract();
strategy.appendField(locator, this, "_abstract", buffer, theAbstract);
}
{
List theLegendURL;
theLegendURL = (((this.legendURL!= null)&&(!this.legendURL.isEmpty()))?this.getLegendURL():null);
strategy.appendField(locator, this, "legendURL", buffer, theLegendURL);
}
{
StyleSheetURL theStyleSheetURL;
theStyleSheetURL = this.getStyleSheetURL();
strategy.appendField(locator, this, "styleSheetURL", buffer, theStyleSheetURL);
}
{
StyleURL theStyleURL;
theStyleURL = this.getStyleURL();
strategy.appendField(locator, this, "styleURL", buffer, theStyleURL);
}
return buffer;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Style)) {
return false;
}
if (this == object) {
return true;
}
final Style that = ((Style) object);
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = that.getName();
if (!strategy.equals(LocatorUtils.property(thisLocator, "name", lhsName), LocatorUtils.property(thatLocator, "name", rhsName), lhsName, rhsName)) {
return false;
}
}
{
String lhsTitle;
lhsTitle = this.getTitle();
String rhsTitle;
rhsTitle = that.getTitle();
if (!strategy.equals(LocatorUtils.property(thisLocator, "title", lhsTitle), LocatorUtils.property(thatLocator, "title", rhsTitle), lhsTitle, rhsTitle)) {
return false;
}
}
{
String lhsAbstract;
lhsAbstract = this.getAbstract();
String rhsAbstract;
rhsAbstract = that.getAbstract();
if (!strategy.equals(LocatorUtils.property(thisLocator, "_abstract", lhsAbstract), LocatorUtils.property(thatLocator, "_abstract", rhsAbstract), lhsAbstract, rhsAbstract)) {
return false;
}
}
{
List lhsLegendURL;
lhsLegendURL = (((this.legendURL!= null)&&(!this.legendURL.isEmpty()))?this.getLegendURL():null);
List rhsLegendURL;
rhsLegendURL = (((that.legendURL!= null)&&(!that.legendURL.isEmpty()))?that.getLegendURL():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "legendURL", lhsLegendURL), LocatorUtils.property(thatLocator, "legendURL", rhsLegendURL), lhsLegendURL, rhsLegendURL)) {
return false;
}
}
{
StyleSheetURL lhsStyleSheetURL;
lhsStyleSheetURL = this.getStyleSheetURL();
StyleSheetURL rhsStyleSheetURL;
rhsStyleSheetURL = that.getStyleSheetURL();
if (!strategy.equals(LocatorUtils.property(thisLocator, "styleSheetURL", lhsStyleSheetURL), LocatorUtils.property(thatLocator, "styleSheetURL", rhsStyleSheetURL), lhsStyleSheetURL, rhsStyleSheetURL)) {
return false;
}
}
{
StyleURL lhsStyleURL;
lhsStyleURL = this.getStyleURL();
StyleURL rhsStyleURL;
rhsStyleURL = that.getStyleURL();
if (!strategy.equals(LocatorUtils.property(thisLocator, "styleURL", lhsStyleURL), LocatorUtils.property(thatLocator, "styleURL", rhsStyleURL), lhsStyleURL, rhsStyleURL)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theName;
theName = this.getName();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "name", theName), currentHashCode, theName);
}
{
String theTitle;
theTitle = this.getTitle();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "title", theTitle), currentHashCode, theTitle);
}
{
String theAbstract;
theAbstract = this.getAbstract();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "_abstract", theAbstract), currentHashCode, theAbstract);
}
{
List theLegendURL;
theLegendURL = (((this.legendURL!= null)&&(!this.legendURL.isEmpty()))?this.getLegendURL():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "legendURL", theLegendURL), currentHashCode, theLegendURL);
}
{
StyleSheetURL theStyleSheetURL;
theStyleSheetURL = this.getStyleSheetURL();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "styleSheetURL", theStyleSheetURL), currentHashCode, theStyleSheetURL);
}
{
StyleURL theStyleURL;
theStyleURL = this.getStyleURL();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "styleURL", theStyleURL), currentHashCode, theStyleURL);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof Style) {
final Style copy = ((Style) draftCopy);
if (this.name!= null) {
String sourceName;
sourceName = this.getName();
String copyName = ((String) strategy.copy(LocatorUtils.property(locator, "name", sourceName), sourceName));
copy.setName(copyName);
} else {
copy.name = null;
}
if (this.title!= null) {
String sourceTitle;
sourceTitle = this.getTitle();
String copyTitle = ((String) strategy.copy(LocatorUtils.property(locator, "title", sourceTitle), sourceTitle));
copy.setTitle(copyTitle);
} else {
copy.title = null;
}
if (this._abstract!= null) {
String sourceAbstract;
sourceAbstract = this.getAbstract();
String copyAbstract = ((String) strategy.copy(LocatorUtils.property(locator, "_abstract", sourceAbstract), sourceAbstract));
copy.setAbstract(copyAbstract);
} else {
copy._abstract = null;
}
if ((this.legendURL!= null)&&(!this.legendURL.isEmpty())) {
List sourceLegendURL;
sourceLegendURL = (((this.legendURL!= null)&&(!this.legendURL.isEmpty()))?this.getLegendURL():null);
@SuppressWarnings("unchecked")
List copyLegendURL = ((List ) strategy.copy(LocatorUtils.property(locator, "legendURL", sourceLegendURL), sourceLegendURL));
copy.legendURL = null;
if (copyLegendURL!= null) {
List uniqueLegendURLl = copy.getLegendURL();
uniqueLegendURLl.addAll(copyLegendURL);
}
} else {
copy.legendURL = null;
}
if (this.styleSheetURL!= null) {
StyleSheetURL sourceStyleSheetURL;
sourceStyleSheetURL = this.getStyleSheetURL();
StyleSheetURL copyStyleSheetURL = ((StyleSheetURL) strategy.copy(LocatorUtils.property(locator, "styleSheetURL", sourceStyleSheetURL), sourceStyleSheetURL));
copy.setStyleSheetURL(copyStyleSheetURL);
} else {
copy.styleSheetURL = null;
}
if (this.styleURL!= null) {
StyleURL sourceStyleURL;
sourceStyleURL = this.getStyleURL();
StyleURL copyStyleURL = ((StyleURL) strategy.copy(LocatorUtils.property(locator, "styleURL", sourceStyleURL), sourceStyleURL));
copy.setStyleURL(copyStyleURL);
} else {
copy.styleURL = null;
}
}
return draftCopy;
}
public Object createNewInstance() {
return new Style();
}
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.INSTANCE;
mergeFrom(null, null, left, right, strategy);
}
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Style) {
final Style target = this;
final Style leftObject = ((Style) left);
final Style rightObject = ((Style) right);
{
String lhsName;
lhsName = leftObject.getName();
String rhsName;
rhsName = rightObject.getName();
String mergedName = ((String) strategy.merge(LocatorUtils.property(leftLocator, "name", lhsName), LocatorUtils.property(rightLocator, "name", rhsName), lhsName, rhsName));
target.setName(mergedName);
}
{
String lhsTitle;
lhsTitle = leftObject.getTitle();
String rhsTitle;
rhsTitle = rightObject.getTitle();
String mergedTitle = ((String) strategy.merge(LocatorUtils.property(leftLocator, "title", lhsTitle), LocatorUtils.property(rightLocator, "title", rhsTitle), lhsTitle, rhsTitle));
target.setTitle(mergedTitle);
}
{
String lhsAbstract;
lhsAbstract = leftObject.getAbstract();
String rhsAbstract;
rhsAbstract = rightObject.getAbstract();
String mergedAbstract = ((String) strategy.merge(LocatorUtils.property(leftLocator, "_abstract", lhsAbstract), LocatorUtils.property(rightLocator, "_abstract", rhsAbstract), lhsAbstract, rhsAbstract));
target.setAbstract(mergedAbstract);
}
{
List lhsLegendURL;
lhsLegendURL = (((leftObject.legendURL!= null)&&(!leftObject.legendURL.isEmpty()))?leftObject.getLegendURL():null);
List rhsLegendURL;
rhsLegendURL = (((rightObject.legendURL!= null)&&(!rightObject.legendURL.isEmpty()))?rightObject.getLegendURL():null);
List mergedLegendURL = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "legendURL", lhsLegendURL), LocatorUtils.property(rightLocator, "legendURL", rhsLegendURL), lhsLegendURL, rhsLegendURL));
target.legendURL = null;
if (mergedLegendURL!= null) {
List uniqueLegendURLl = target.getLegendURL();
uniqueLegendURLl.addAll(mergedLegendURL);
}
}
{
StyleSheetURL lhsStyleSheetURL;
lhsStyleSheetURL = leftObject.getStyleSheetURL();
StyleSheetURL rhsStyleSheetURL;
rhsStyleSheetURL = rightObject.getStyleSheetURL();
StyleSheetURL mergedStyleSheetURL = ((StyleSheetURL) strategy.merge(LocatorUtils.property(leftLocator, "styleSheetURL", lhsStyleSheetURL), LocatorUtils.property(rightLocator, "styleSheetURL", rhsStyleSheetURL), lhsStyleSheetURL, rhsStyleSheetURL));
target.setStyleSheetURL(mergedStyleSheetURL);
}
{
StyleURL lhsStyleURL;
lhsStyleURL = leftObject.getStyleURL();
StyleURL rhsStyleURL;
rhsStyleURL = rightObject.getStyleURL();
StyleURL mergedStyleURL = ((StyleURL) strategy.merge(LocatorUtils.property(leftLocator, "styleURL", lhsStyleURL), LocatorUtils.property(rightLocator, "styleURL", rhsStyleURL), lhsStyleURL, rhsStyleURL));
target.setStyleURL(mergedStyleURL);
}
}
}
public void setLegendURL(List value) {
this.legendURL = null;
if (value!= null) {
List draftl = this.getLegendURL();
draftl.addAll(value);
}
}
public Style withName(String value) {
setName(value);
return this;
}
public Style withTitle(String value) {
setTitle(value);
return this;
}
public Style withAbstract(String value) {
setAbstract(value);
return this;
}
public Style withLegendURL(LegendURL... values) {
if (values!= null) {
for (LegendURL value: values) {
getLegendURL().add(value);
}
}
return this;
}
public Style withLegendURL(Collection values) {
if (values!= null) {
getLegendURL().addAll(values);
}
return this;
}
public Style withStyleSheetURL(StyleSheetURL value) {
setStyleSheetURL(value);
return this;
}
public Style withStyleURL(StyleURL value) {
setStyleURL(value);
return this;
}
public Style withLegendURL(List value) {
setLegendURL(value);
return this;
}
}