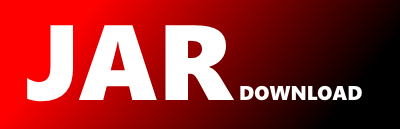
WMS_1_1_0.StyleSheetURL Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.10.31 at 02:43:57 PM CET
//
package WMS_1_1_0;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.CopyStrategy;
import org.jvnet.jaxb2_commons.lang.CopyTo;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBMergeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.MergeFrom;
import org.jvnet.jaxb2_commons.lang.MergeStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"format",
"onlineResource"
})
@XmlRootElement(name = "StyleSheetURL")
public class StyleSheetURL
implements Cloneable, CopyTo, Equals, HashCode, MergeFrom, ToString
{
@XmlElement(name = "Format", required = true)
protected Format format;
@XmlElement(name = "OnlineResource", required = true)
protected OnlineResource onlineResource;
/**
* Gets the value of the format property.
*
* @return
* possible object is
* {@link Format }
*
*/
public Format getFormat() {
return format;
}
/**
* Sets the value of the format property.
*
* @param value
* allowed object is
* {@link Format }
*
*/
public void setFormat(Format value) {
this.format = value;
}
/**
* Gets the value of the onlineResource property.
*
* @return
* possible object is
* {@link OnlineResource }
*
*/
public OnlineResource getOnlineResource() {
return onlineResource;
}
/**
* Sets the value of the onlineResource property.
*
* @param value
* allowed object is
* {@link OnlineResource }
*
*/
public void setOnlineResource(OnlineResource value) {
this.onlineResource = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
Format theFormat;
theFormat = this.getFormat();
strategy.appendField(locator, this, "format", buffer, theFormat);
}
{
OnlineResource theOnlineResource;
theOnlineResource = this.getOnlineResource();
strategy.appendField(locator, this, "onlineResource", buffer, theOnlineResource);
}
return buffer;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof StyleSheetURL)) {
return false;
}
if (this == object) {
return true;
}
final StyleSheetURL that = ((StyleSheetURL) object);
{
Format lhsFormat;
lhsFormat = this.getFormat();
Format rhsFormat;
rhsFormat = that.getFormat();
if (!strategy.equals(LocatorUtils.property(thisLocator, "format", lhsFormat), LocatorUtils.property(thatLocator, "format", rhsFormat), lhsFormat, rhsFormat)) {
return false;
}
}
{
OnlineResource lhsOnlineResource;
lhsOnlineResource = this.getOnlineResource();
OnlineResource rhsOnlineResource;
rhsOnlineResource = that.getOnlineResource();
if (!strategy.equals(LocatorUtils.property(thisLocator, "onlineResource", lhsOnlineResource), LocatorUtils.property(thatLocator, "onlineResource", rhsOnlineResource), lhsOnlineResource, rhsOnlineResource)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
Format theFormat;
theFormat = this.getFormat();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "format", theFormat), currentHashCode, theFormat);
}
{
OnlineResource theOnlineResource;
theOnlineResource = this.getOnlineResource();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "onlineResource", theOnlineResource), currentHashCode, theOnlineResource);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof StyleSheetURL) {
final StyleSheetURL copy = ((StyleSheetURL) draftCopy);
if (this.format!= null) {
Format sourceFormat;
sourceFormat = this.getFormat();
Format copyFormat = ((Format) strategy.copy(LocatorUtils.property(locator, "format", sourceFormat), sourceFormat));
copy.setFormat(copyFormat);
} else {
copy.format = null;
}
if (this.onlineResource!= null) {
OnlineResource sourceOnlineResource;
sourceOnlineResource = this.getOnlineResource();
OnlineResource copyOnlineResource = ((OnlineResource) strategy.copy(LocatorUtils.property(locator, "onlineResource", sourceOnlineResource), sourceOnlineResource));
copy.setOnlineResource(copyOnlineResource);
} else {
copy.onlineResource = null;
}
}
return draftCopy;
}
public Object createNewInstance() {
return new StyleSheetURL();
}
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.INSTANCE;
mergeFrom(null, null, left, right, strategy);
}
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof StyleSheetURL) {
final StyleSheetURL target = this;
final StyleSheetURL leftObject = ((StyleSheetURL) left);
final StyleSheetURL rightObject = ((StyleSheetURL) right);
{
Format lhsFormat;
lhsFormat = leftObject.getFormat();
Format rhsFormat;
rhsFormat = rightObject.getFormat();
Format mergedFormat = ((Format) strategy.merge(LocatorUtils.property(leftLocator, "format", lhsFormat), LocatorUtils.property(rightLocator, "format", rhsFormat), lhsFormat, rhsFormat));
target.setFormat(mergedFormat);
}
{
OnlineResource lhsOnlineResource;
lhsOnlineResource = leftObject.getOnlineResource();
OnlineResource rhsOnlineResource;
rhsOnlineResource = rightObject.getOnlineResource();
OnlineResource mergedOnlineResource = ((OnlineResource) strategy.merge(LocatorUtils.property(leftLocator, "onlineResource", lhsOnlineResource), LocatorUtils.property(rightLocator, "onlineResource", rhsOnlineResource), lhsOnlineResource, rhsOnlineResource));
target.setOnlineResource(mergedOnlineResource);
}
}
}
public StyleSheetURL withFormat(Format value) {
setFormat(value);
return this;
}
public StyleSheetURL withOnlineResource(OnlineResource value) {
setOnlineResource(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy