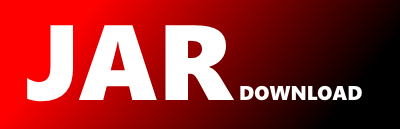
org.jlibrtp.protocols.rtp.CircularByteBuffer Maven / Gradle / Ivy
The newest version!
/**
* Java RTP Library (jlibrtp)
* Copyright (C) 2009 Arne Kepp
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
package org.jlibrtp.protocols.rtp;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class CircularByteBuffer {
private int readOfs = 0;
private int writeOfs = 0;
private int initialSize;
private CircularByteBufferInputStream is;
private CircularByteBufferOutputStream os;
private byte[] buf;
public CircularByteBuffer(int initialSize) {
buf = new byte[initialSize];
this.initialSize = initialSize;
is = new CircularByteBufferInputStream(this);
os = new CircularByteBufferOutputStream(this);
}
private synchronized int bytesLeft() {
return buf.length - bytesUsed();
}
private synchronized int bytesUsed() {
if(readOfs == writeOfs)
return 0;
if(readOfs < writeOfs)
return writeOfs - readOfs;
return buf.length - (writeOfs - readOfs);
}
private void doubleBuf() {
byte[] oldBuf = buf;
buf = new byte[oldBuf.length*2];
System.arraycopy(oldBuf, 0, buf, 0, oldBuf.length);
}
private synchronized void write(byte[] data, int offset, int length) {
while(length > bytesLeft()) {
doubleBuf();
}
if(writeOfs + length > buf.length) {
int endLength = buf.length - this.writeOfs;
System.arraycopy(data, 0, buf, writeOfs, endLength);
writeOfs = 0;
length = length - endLength;
offset = endLength + offset;
}
System.arraycopy(data, offset, buf, writeOfs, length);
writeOfs += length;
}
private synchronized int read(byte[] buffer, int offset, int length) {
int maxLeft= this.bytesUsed();
int bytesLeft = length;
if(maxLeft < bytesLeft) {
bytesLeft = maxLeft;
length = maxLeft;
}
if(readOfs + length > buf.length) {
int endLength = buf.length - readOfs;
System.arraycopy(buf, readOfs, buffer, offset, endLength);
readOfs = 0;
bytesLeft = bytesLeft - endLength;
offset = offset + endLength;
}
System.arraycopy(buf, readOfs, buffer, offset, bytesLeft);
readOfs += bytesLeft;
return length;
}
public synchronized void clear() {
readOfs = 0;
writeOfs = 0;
buf = new byte[this.initialSize];
}
public String debugPrintFunction() {
return this.buf.length + " " + readOfs + " " + writeOfs + " " + this.bytesLeft() + " " + this.bytesUsed();
}
public String debugPrintData() {
String str = "";
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy