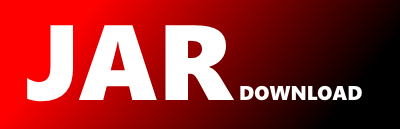
org.jwall.apache.httpd.ApacheLayout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd;
import java.io.File;
import java.io.InputStream;
import java.util.LinkedList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.annotations.XStreamAlias;
/**
*
* This class provides a simple container for information that is needed to properly
* set up all paths to the Apache web server files, what shall be used to create an
* Apache controller instance.
*
*
* This class does also incorporate the automatic layout guessing by probing different
* properties and files on the local system.
*
*
* @author Christian Bockermann <[email protected]>
*
*/
@XStreamAlias("ApacheLayout")
public class ApacheLayout
{
private static Logger log = Logger.getLogger( "org.apache.httpd.ApacheLayout.class" );
/** This attribute holds the apache home directory. */
public transient String APACHE_HOME = "/usr/sbin";
/** This file points to the apachectl script */
@XStreamAlias("control-skript")
public File apachectl = new File(APACHE_HOME+"/apachectl");
/** This shall at some later time point to the main apache configuration file. */
@XStreamAlias("httpd-config")
private File config = null;
/** The apache binary */
@XStreamAlias("httpd-binary")
public File httpd = null;
/** The apache home directory */
@XStreamAlias("home-directory")
private File apacheHome = null;
/** This is the name of the property which is used to locate the httpd.conf file */
public final static String PROPERTY_APACHE_CONFIG_LOCATION = "org.apache.conf";
/** The may be several names for the configuration file which are all probed for */
private static String[] HTTPD_CONFIG_NAMES = new String[]{
"httpd.conf",
"apache2.conf"
};
/** These are the name of possible candidates for the apache binary */
private static String[] HTTPD_NAMES = new String[]{
"httpd",
"httpd2",
"apache",
"apache2",
"httpd.exe"
};
@SuppressWarnings("unused")
private static String[] APACHE_HOMES = new String[]{
"/www/apache2",
"/usr/lib/apache2",
"/usr/local/apache",
"/usr/local/httpd"
};
/** When looking for the apachectl script, these locations get scanned: */
private static String[] APACHE_CTL_LOCATIONS = new String[]{
"/www/apache2/bin",
"/usr/sbin",
"/usr/local/sbin",
"/usr/local/httpd/bin",
"C:/Programme/Apache Software Foundation/Apache2.2/bin",
"C:/Programs/Apache Software Foundation/Apache2.2/bin"
};
private File locateApacheCtl(){
List files = new LinkedList();
files.add( apacheHome.getAbsolutePath() );
for( String s : APACHE_CTL_LOCATIONS )
files.add( s );
for( String loc : files ){
File f = new File( loc + "/apache2ctl" );
if( f.exists() )
return f;
f = new File( loc + "/apachectl" );
if( f.exists() )
return f;
}
// no apache2ctl found...
return null;
}
public File getHttpdConfig(){
return config;
}
private File locateHttpd(){
for( String loc : APACHE_CTL_LOCATIONS ){
for( String name : HTTPD_NAMES ){
File f = new File( loc + "/" + name );
if( f.exists() ){
apacheHome = f.getParentFile().getParentFile();
return f;
}
}
}
return null;
}
private File locateHttpdConf(){
List files = new LinkedList();
if( System.getProperty( "org.apache.conf" ) != null )
files.add( System.getProperty( "org.apache.conf") );
// If the httpd binary is already located, we might have a chance to
// find the associated config right beneath at ../conf
//
if( httpd != null && httpd.getParentFile() != null && httpd.getParentFile().getParentFile() != null ){
String relatedConfig = httpd.getParentFile().getParentFile().getAbsolutePath() + File.separator + "conf";
log.info( "Adding config-location related to " + httpd.getAbsolutePath() + ": " + relatedConfig );
files.add( relatedConfig );
}
files.add( "/etc/apache2" );
files.add( "/etc/httpd" );
files.add( apacheHome.getAbsolutePath() + File.separator + "conf" );
for( String s : APACHE_CTL_LOCATIONS )
files.add( s );
for( String loc : files ){
for( String name : HTTPD_CONFIG_NAMES ){
File f = new File( loc + "/" + name );
log.info( "Checking for config @ " + f.getAbsolutePath() );
if( f.exists() ){
return f;
}
}
}
return null;
}
/**
* This method parses the layout description from the given input stream. Parsing is
* done using the xstream library.
*
* @param in
* @return
* @throws Exception
*/
public static ApacheLayout read( InputStream in) throws Exception {
XStream xs = new XStream();
xs.processAnnotations( ApacheLayout.class );
ApacheLayout layout = (ApacheLayout) xs.fromXML( in );
return layout;
}
/**
* This method will start to probe several places to find apache configuration files.
* This can be a convenient way to set up an Apache controller instance by automatically
* probing for existence of the apachectl
-script and Apache's configuration
* files.
*
* @return An instance of this class which contains the best guess for a layout.
*/
public static ApacheLayout guess(){
ApacheLayout layout = new ApacheLayout();
log.info( "Guessing Apache locations, System is " + System.getProperty("os.name") );
layout.httpd = layout.locateHttpd();
if( layout.httpd != null )
log.warning( "Found Apache binary @ " + layout.httpd.getAbsolutePath() );
layout.config = layout.locateHttpdConf();
if( layout.config != null )
log.warning( "Found Apache configuration @ " + layout.config.getAbsolutePath() );
layout.apachectl = layout.locateApacheCtl();
if( layout.apachectl != null )
log.warning( "Found \"apachectl\" script @ " + layout.apachectl.getAbsolutePath() );
return layout;
}
public File getApacheCtl(){
return apachectl;
}
public static void main( String args[] ){
log.setLevel( Level.OFF );
log.info("Trying to guess the apache layout:");
ApacheLayout layout = ApacheLayout.guess();
XStream xs = new XStream();
xs.processAnnotations( ApacheLayout.class );
System.out.println( xs.toXML( layout ) );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy