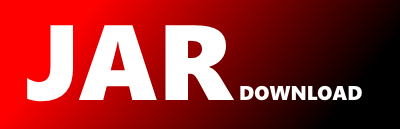
org.jwall.apache.httpd.config.AbstractDirective Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.config;
import java.io.File;
import java.io.Serializable;
import java.util.LinkedList;
import java.util.List;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamAsAttribute;
import com.thoughtworks.xstream.annotations.XStreamImplicit;
/**
*
* This class represents a simple Apache server directive.
*
* @author [email protected]
*
*/
@XStreamAlias("Directive")
public abstract class AbstractDirective
implements Comparable, Serializable, Directive
{
/** The unique class ID */
private static final long serialVersionUID = 3995333552634180617L;
public final static boolean SHOW_LOCATION = "true".equals( System.getProperty("org.apache.config.xml.show_location") );
@XStreamAsAttribute
protected String name = "";
@XStreamImplicit
protected LinkedList args;
@XStreamAlias("file")
@XStreamAsAttribute
protected String loc = "";
transient String rawLine = "";
protected transient Position location;
transient ContainerDirective parent;
transient boolean enabled = true;
/**
*
* @param name
* @param file
* @param line
*/
public AbstractDirective( String name, File file, int line ){
this.rawLine = name;
location = new Position( file, line );
loc = location.toString();
args = new LinkedList();
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#getName()
*/
public String getName(){
return name;
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#getArgs()
*/
public List getArgs(){
return args;
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#depth()
*/
public int depth(){
if( parent == null )
return 0;
return parent.depth() + 1;
}
/**
* @return the rawLine
*/
public String getRawLine()
{
return rawLine;
}
/**
* @param rawLine the rawLine to set
*/
public void setRawLine(String rawLine)
{
this.rawLine = rawLine;
}
/**
* @return the enabled
*/
public boolean isEnabled()
{
return enabled;
}
/**
* @param enabled the enabled to set
*/
public void setEnabled(boolean enabled)
{
this.enabled = enabled;
}
public String toString(){
StringBuffer s = new StringBuffer();
s.append( prefix() + name );
if( ! enabled )
s.append( " enabled=\"false\"");
if( args != null ){
for( String arg : args )
if( arg != null )
s.append( " " + arg );
}
//s.append("\n");
if( SHOW_LOCATION )
s.append( " # "+location.toString() );
return s.toString();
}
public String toXML(){
StringBuffer s = new StringBuffer( prefix() );
String loc = "";
if( SHOW_LOCATION )
loc = " location=\""+ location.toString() + "\"";
s.append("<"+name+loc+">");
if( args != null )
for( int i = 0; i < args.size(); i++ ){
if( i > 0 )
s.append(" ");
s.append( args.get(i) );
}
s.append( this.prefix() + ""+name+">");
return s.toString();
}
public String prefix(){
StringBuffer s = new StringBuffer();
for( int i = 0; i < depth(); i++ )
s.append(" ");
return s.toString();
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#compareTo(org.jwall.apache.config.Directive)
*/
public int compareTo( AbstractDirective other ){
if( this == other )
return 0;
if( location.sameFile( other.location ) ) {
return location.compareTo( other.location );
} else {
if( parent == null && other.parent != null )
return 1;
if( parent != null && other.parent == null )
return -1;
}
return -1;
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#isBelow(org.jwall.apache.config.DirectiveInterface)
*/
public boolean isBelow( Directive other ){
if( parent == null )
return false;
if( parent == other )
return true;
else
return parent.isBelow( other );
}
public String removeQuotes( String s ){
int start = 0;
if( s.startsWith("\"") )
s = s.substring( ++start );
if( s.endsWith("\"") )
s = s.substring(0, s.length() - 1 );
return s;
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#toPlainTxt()
*/
public String toPlainTxt(){
return rawLine;
/*
StringBuffer s = new StringBuffer();
for( int i = 0; i < this.depth(); i++ )
s.append(" ");
if( rawLine != null )
s.append( rawLine.trim() );
return s.toString(); //rawLine.trim();
*/
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#getLocation()
*/
public Position getLocation(){
return location;
}
/* (non-Javadoc)
* @see org.jwall.apache.config.DirectiveInterface#getProviderName()
*/
public String getProviderName(){
return "Apache";
}
public boolean matches( String s ){
return name != null && name.matches( s );
}
public int compareTo( Directive d ){
if( this == d )
return 0;
return this.getLocation().compareTo( d.getLocation() );
// return this.name.compareTo( d.getName() );
}
public static String HTMLEntityEncode( String s )
{
StringBuffer buf = new StringBuffer();
int len = (s == null ? -1 : s.length());
for ( int i = 0; i < len; i++ )
{
char c = s.charAt( i );
if ( c>='a' && c<='z' || c>='A' && c<='Z' || c>='0' && c<='9' )
{
buf.append( c );
}
else
{
buf.append( "" + (int)c + ";" );
}
}
return buf.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy