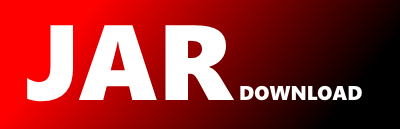
org.jwall.apache.httpd.config.ContainerDirective Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.config;
import java.io.File;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.TreeSet;
import java.util.Vector;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamImplicit;
@XStreamAlias("ContainerDirective")
public class ContainerDirective
extends AbstractDirective
{
private static final long serialVersionUID = 3957948047475584242L;
@XStreamImplicit
TreeSet nested = new TreeSet();
public ContainerDirective( String line, File file, int lineNum ){
super( line, file, lineNum );
if( line.trim().startsWith( "<" ) )
name = line.trim().substring(1); // chop off leading '<'
if( name.endsWith( ">" ) )
name = name.substring(0, name.length() - 1 ); // chop off trailing '>'
String[] token = name.split("\\s+", 2);
name = token[0];
if( token.length > 1 )
for( String param : token[1].split( "\\s+" ) )
args.add( param );
}
public void add( AbstractDirective d ){
d.parent = this;
nested.add( d );
}
/**
* @return
*/
public Collection getChildren(){
return nested;
}
public Vector getChildren( String name ){
Vector c = new Vector();
for( Directive d : getChildren() )
if( d.getName().toLowerCase().equals(name.toLowerCase()) )
c.add( d );
//
// if no virtual-host turned out, we probably need to descent one level further...
//
/*
if( c.size() == 0 && getChildren().size() > 0 ){
for( Directive d: getChildren("ApacheConfig") ){
ContainerDirective sub = (ContainerDirective) d;
c.addAll( sub.getChildren( name ) );
}
}
*/
return c;
}
public List findDirectiveByName( String name, boolean recursive ){
List found = new LinkedList();
for( Directive d : this.getChildren() ){
if( d.getName().matches( name ) || d.matches( name ) )
found.add(d);
}
if( recursive )
for( Directive subConfig : this.nested )
if( subConfig instanceof ContainerDirective ){
ContainerDirective container = (ContainerDirective) subConfig;
found.addAll( container.findDirectiveByName( name, recursive ) );
}
return found;
}
public List findDirectiveByProviderName( String provider, boolean recursive ){
List found = new LinkedList();
for( Directive d: getChildren() ){
if( d.getProviderName().equals( provider ) )
found.add( d );
}
if( recursive ){
for( Directive subConfig : nested )
if( subConfig instanceof ContainerDirective ){
ContainerDirective container = (ContainerDirective) subConfig;
found.addAll( container.findDirectiveByProviderName( provider, recursive) );
}
}
return found;
}
public String toString(){
StringBuffer s = new StringBuffer();
s.append( prefix() + "<"+name);
for( String arg : args )
s.append(" "+arg);
if( SHOW_LOCATION )
s.append("> # " + location + "\n");
else
s.append(">\n");
for( Directive d : nested ){
s.append( d.toString() + "\n" );
}
s.append( prefix() + ""+name+">\n");
return s.toString();
}
public String toPlainTxt(){
StringBuffer s = new StringBuffer();
s.append( prefix() + "<"+name);
if( args != null ){
for( String arg : args )
s.append(" "+arg);
}
s.append(">\n");
for( Directive d : nested ){
s.append( d.toPlainTxt() + "\n" );
}
s.append( prefix() + ""+name+">");
return s.toString();
}
public String toXML(){
StringBuffer s = new StringBuffer( prefix() );
String loc = "";
if( SHOW_LOCATION && location != null )
loc = " location=\""+ location.toString() + "\"";
s.append("<" + getName() + loc + ">");
if( args != null )
for( int i = 0; i < args.size(); i++ ){
if( i > 0 )
s.append(" ");
s.append( args.get(i) );
}
if( !this.nested.isEmpty() ){
s.append("\n");
for( Directive d : this.nested )
s.append( d.toXML() + "\n" );
}
s.append( prefix() + "" + getName() + ">\n");
return s.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy