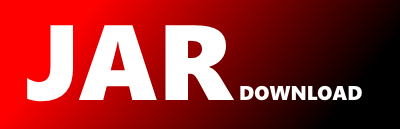
org.jwall.apache.httpd.config.Macro Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.config;
import java.io.File;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Macro extends ContainerDirective {
static Logger log = LoggerFactory.getLogger(Macro.class);
/** The unique class id */
private static final long serialVersionUID = -6440453746268549039L;
final String name;
String body = "";
public Macro(String line, File file, int lineNum) {
super(line, file, lineNum);
log.info("Creating macro object from line {}", line);
log.debug(" Macro args are: {}", getArgs());
this.name = getArgs().remove(0).toLowerCase();
}
public String getName() {
return name;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public String expand(List args) throws ParseException {
Map subs = new HashMap();
if (args.size() != getArgs().size()) {
throw new ParseException("Number of arguments for macro '" + name
+ "' does not match!");
}
for (int i = 0; i < args.size(); i++) {
subs.put(getArgs().get(i), args.get(i));
}
String exp = body;
for (String key : subs.keySet()) {
String repl = subs.get(key);
int repls = 0;
while (exp.indexOf(key) > 0) {
exp = exp.replace(key, repl);
repls++;
}
log.debug("replaced {} occurences of key '{}'", repls, key);
}
return exp;
}
public String toString() {
return toXML();
}
public String toXML() {
StringBuffer s = new StringBuffer(prefix());
String loc = "";
if (SHOW_LOCATION && location != null)
loc = " location=\"" + location.toString() + "\"";
StringBuffer arguments = new StringBuffer();
Iterator it = args.iterator();
while (it.hasNext()) {
String arg = it.next();
arguments.append(arg);
if (it.hasNext()) {
arguments.append(" ");
}
}
s.append(prefix() + "");
s.append("");
s.append(" \n");
return s.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy