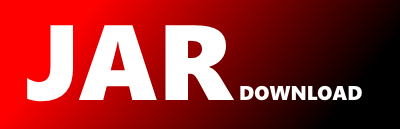
org.jwall.apache.httpd.config.SecRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.config;
import java.io.File;
import java.util.LinkedList;
import java.util.List;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamAsAttribute;
@XStreamAlias("SecRule")
public class SecRule
extends AbstractDirective
{
private static final long serialVersionUID = -6959753220166367641L;
static XStream xs = new XStream();
static {
xs.processAnnotations( SecRule.class );
xs.processAnnotations( SecAction.class );
}
@XStreamAsAttribute
protected String id = "";
@XStreamAlias("Selector")
protected String selector;
@XStreamAlias("Operator")
protected String operator;
@XStreamAlias("Actions")
LinkedList actions = null;
/**
* Create a new instance by parsing the given line and creating the appropriate attributes.
*
* @param line The raw configuration line as found in the config file.
* @param file The file from which this line has been read.
* @param lineNum The line number where this line can be found in the file.
* @throws ParseException
*/
public SecRule( String line, File file, int lineNum ) throws ParseException {
super( line, file, lineNum );
name = "SecRule";
actions = new LinkedList();
List args = QuotedStringTokenizer.splitRespectQuotes( line.trim() , ' ' ); //.split(" ");
if( args.size() < 1 || !args.get( 0 ).trim().equals("SecRule"))
throw new ParseException("Not a SecRule-directive: "+line);
if( args.size() > 1 )
selector = removeQuotes(args.get(1));
if( args.size() > 2 )
operator = removeQuotes(args.get(2));
if( args.size() > 3 ){
String unquoted = removeQuotes( args.get( 3 ) );
for( String action : QuotedStringTokenizer.splitRespectQuotes( unquoted , ',' ) ){
String act = removeQuotes( action.trim() );
actions.add( act );
if( action.startsWith("id") ){
id = action.replaceFirst("id:", "");
if( id.startsWith( "'" ) )
id = id.substring(1);
if( id.endsWith( "'" ) )
id = id.substring( 0, id.length() - 1 );
}
}
}
}
public String toXML(){
return xs.toXML( this );
/*
StringBuffer s = new StringBuffer(prefix()+"\n");
s.append( prefix() + " "+HTMLEntityEncode(selector)+" \n");
s.append( prefix() + " "+HTMLEntityEncode(operator)+" \n");
if( actions != null ){
//s.append( prefix() + " "+actions+" \n");
for( String action : actions ){
s.append( prefix() + " " );
s.append( action );
s.append( " \n" );
}
}
s.append(prefix() + " ");
return s.toString();
*/
}
public String getProviderName(){
return "mod_security.c";
}
/**
* @return the selector
*/
public String getSelector()
{
return selector;
}
/**
* @param selector the selector to set
*/
public void setSelector(String selector)
{
this.selector = selector;
}
/**
* @return the operator
*/
public String getOperator()
{
return operator;
}
/**
* @param operator the operator to set
*/
public void setOperator(String operator)
{
this.operator = operator;
}
/**
* @return the actions
*/
public LinkedList getActions()
{
return actions;
}
/**
* @param actions the actions to set
*/
public void setActions(LinkedList actions)
{
this.actions = actions;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy