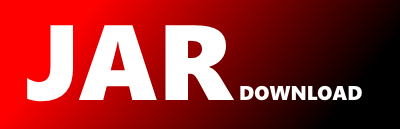
org.jwall.apache.httpd.service.AbstractFileSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.service;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import org.jwall.apache.httpd.MD5;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public abstract class AbstractFileSystem implements FileSystem {
static Logger log = LoggerFactory.getLogger( AbstractFileSystem.class );
List listener = new ArrayList();
public void addFileSystemListener( FileSystemListener l ){
if( ! listener.contains( l ) )
listener.add( l );
}
public void removeFileSystemListener( FileSystemListener l ){
if( listener.contains( l ) )
listener.remove( l );
}
protected File map( File file ){
return file;
}
protected File unmap( File file ){
return file;
}
/**
* @see org.jwall.apache.httpd.service.FileSystem#md5(java.io.File)
*/
public String md5(File file) throws IOException {
log.debug( "Computing md5 sum of {} (operating on {})", file, map(file) );
if( ! exists( file ) ){
log.debug( " file {} does not exist!", file );
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
InputStream fis = openInputStream( file );
byte[] buf = new byte[ 16 * 1024 ];
int read = fis.read( buf );
while( read > 0 ){
out.write( buf, 0, read );
read = fis.read( buf );
}
out.close();
return MD5.md5( out.toByteArray() );
}
public void copy(File file, FileSystem fs, File targetFile ) throws IOException {
log.debug( " copy( {}, fs, {} )", file, targetFile );
if( ! isSymlink( file ) ){
log.debug( "Not a symbolic link: {}", file );
copy( this.openInputStream( file ), fs.openOutputStream( targetFile ) );
} else {
String target = readSymlink( file ); // this.getAbsolutePath(targetFile).getRealPath( file );
File real = new File( resolveSymlink( file ).getPath() );
log.debug( "Need to copy 'real file' {} for symblic link {}", real, file );
copy( real, fs, real );
log.debug( " Creating symblink link {} -> {} ", file, target );
fs.createSymlink( target, file );
}
}
public void copy( File file, FileSystem targetFs ) throws IOException {
copy( file, targetFs, file );
}
public long copy( InputStream in, OutputStream out ) throws IOException {
byte[] buf = new byte[ 16 * 1024 ];
long total = 0L;
int read = in.read( buf );
while( read > 0 ){
out.write( buf, 0, read );
total += read;
read = in.read( buf );
}
log.debug( "copied {} bytes ", total );
out.flush();
out.close();
return total;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy