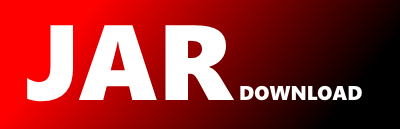
org.jwall.apache.httpd.service.DefaultFileSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apache-config Show documentation
Show all versions of apache-config Show documentation
A Java library for reading Apache httpd configuration files
The newest version!
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
*
* Copyright (C) 2010-2014 Christian Bockermann
*
* This file is part of the jwall.org apache-config library. The apache-config library is
* a parsing library to handle Apache HTTPD configuration files.
*
* More information and documentation for the jwall-tools can be found at
*
* http://www.jwall.org/apache-config
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program; if not, see .
*
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
package org.jwall.apache.httpd.service;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.Reader;
import java.net.URL;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DefaultFileSystem extends AbstractFileSystem {
static Logger log = LoggerFactory.getLogger( DefaultFileSystem.class );
File baseDir;
public DefaultFileSystem(){
this.baseDir = null;
}
public DefaultFileSystem( String base ) throws IOException {
this( new File( base ) );
}
public DefaultFileSystem( File baseDir ) throws IOException {
this.baseDir = baseDir;
if( this.baseDir.isFile() )
throw new IOException( "Cannot create file-system within a file!" );
if( ! baseDir.isDirectory() )
baseDir.mkdirs();
}
protected File map( File file ){
if( baseDir == null )
return file;
return new File( baseDir.getAbsolutePath() + File.separator + file.getPath() );
}
protected File unmap( File file ){
if( baseDir == null )
return file;
String prefix = baseDir.getAbsolutePath();
if( file.getAbsolutePath().startsWith( prefix ) ){
File f = new File( file.getAbsolutePath().replaceFirst( prefix, "" ) );
log.debug( "Un-mapped file: {}", f.getAbsolutePath() );
return f;
}
return file;
}
public Reader openFile(File file) throws IOException {
return new FileReader( map(file) );
}
public boolean isDirectory(File file) {
return map(file).isDirectory();
}
public File[] listFiles(File directory) {
File[] list = map(directory).listFiles();
for( int i = 0; i < list.length; i++ )
list[i] = unmap( list[i] );
return list;
}
public boolean canRead(File file) {
return map(file).canRead();
}
public boolean exists(File file) {
return map(file).exists();
}
public String getAbsolutePath(File file) {
return map(file).getAbsolutePath();
}
/**
* @see org.jwall.apache.httpd.service.FileSystem#getURL()
*/
public URL getURL( File file ) {
try {
return map(file).toURI().toURL();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public InputStream openInputStream(File file) throws IOException {
log.debug( "Opening FileInputStream on {}", map(file) );
return new FileInputStream( map(file) );
}
public OutputStream openOutputStream(File file) throws IOException {
File out = map( file );
log.debug( "Opening output-stream to {}", out );
if( out.getParentFile() != null && ! out.getParentFile().isDirectory() ){
out.getParentFile().mkdirs();
log.debug( "Created directory {}", out.getParent() );
}
return new FileOutputStream( out );
}
public byte[] get(File f) throws IOException {
File file = map( f );
Long size = file.length();
byte[] buf = new byte[ size.intValue() ];
FileInputStream fis = new FileInputStream( file );
fis.read( buf );
fis.close();
return buf;
}
public void put(File file, byte[] content) throws IOException {
OutputStream out = this.openOutputStream( map(file) );
out.write( content );
out.close();
}
public void createSymlink( String destination, File name) throws IOException {
log.debug( "Create symlink: {} -> {}", destination, name );
log.debug( " relative from {}", name.getParent() );
if( name.getParentFile() != null )
createDirectory( name.getParentFile() );
String cmd = "ln -s " + destination + " " + map(name); //.getAbsolutePath();
log.debug( "Creating symlink: {}", cmd );
Runtime.getRuntime().exec( cmd );
}
public boolean isSymlink(File file) {
try {
String cmd = "readlink " + map(file).getAbsolutePath();
Process p = Runtime.getRuntime().exec( cmd );
BufferedReader r = new BufferedReader( new InputStreamReader( p.getInputStream() ) );
String result = r.readLine();
r.close();
return result != null && !result.equals( file.getAbsolutePath() );
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
public String readSymlink( File file ) throws IOException {
try {
String cmd = "readlink " + map(file).getAbsolutePath();
Process p = Runtime.getRuntime().exec( cmd );
BufferedReader r = new BufferedReader( new InputStreamReader( p.getInputStream() ) );
String result = r.readLine();
r.close();
if( result != null )
return result;
return result;
} catch (Exception e) {
e.printStackTrace();
}
return "";
}
public boolean createDirectory(File file) throws IOException {
return map(file).mkdirs();
}
public boolean delete(File file) throws IOException {
return map(file).delete();
}
public File resolveSymlink(File symlink) throws IOException {
String lnk = readSymlink( symlink );
File dst = new File( lnk );
if( dst.isAbsolute() )
return dst;
else
return new File( symlink.getParent() + File.separator + lnk );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy