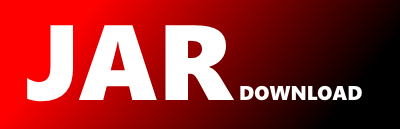
org.jwall.audit.EventView Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.audit;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.jwall.web.audit.filter.FilterExpression;
import org.jwall.web.audit.filter.Operator;
/**
* This interface defines a view for a set of log events. It allows for
* filtering and counting log events based on filter expressions.
*
* @author Christian Bockermann <[email protected]>
*
*/
public interface EventView {
/**
* This method returns the variable names which are supported for filtering
* by this view.
*
* @return
*/
public Set getVariables();
/**
* This method returns the set of variables which are indexed by a view.
* Indexed variables require no additional parsing of events and are thus
* much more efficiently to be accessed.
*
* @return
*/
public Set getIndexedVariables();
/**
* This method returns the list of values observed for the specified
* variable. Implementation of this method is optional, this method my
* simply return an empty list.
*
* @param variable
* @return
*/
public List getCompletions(String variable);
/**
* Returns the number of log-messages within this view which match the given
* filter expression.
*
* @param filter
* @return
*/
public Long count(FilterExpression filter) throws Exception;
/**
* Returns the counts of events grouped by the specified variable.
*
* @param variable
* @param filter
* @return
* @throws Exception
*/
public Map count(String variable, FilterExpression filter)
throws Exception;
/**
* Returns the list of log-messages which match the given filter expression.
* The offset and limit can be used to allow for a paging view.
*
* @param filter
* @param offset
* @param limit
* @return
*/
public List list(FilterExpression filter, int offset, int limit)
throws Exception;
/**
* Retrieve the event with the given ID from the view.
*
* @param id
* @return
* @throws Exception
*/
public E get(String id) throws Exception;
/**
* Delete the event with the given ID from the view (and the underlying
* storage).
*
* @param id
* @return
* @throws Exception
* @deprecated Use delete(FilterExpression) with a filter of TX_ID=id
*/
public Long delete(String id) throws Exception;
public Long delete(FilterExpression filter) throws Exception;
public void tag(FilterExpression filter, String tag) throws Exception;
public void untag(FilterExpression filter, String tag) throws Exception;
public Set getSupportedOperators();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy