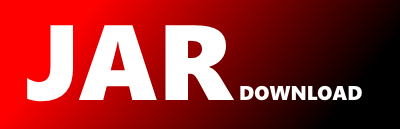
org.jwall.log.LogMessageProcessorPipeline Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.log;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Map;
import org.jwall.audit.EventProcessor;
import org.jwall.audit.EventProcessorException;
import org.jwall.audit.EventProcessorPipeline;
public class LogMessageProcessorPipeline
implements EventProcessorPipeline
{
final Order> order = new Order>();
ArrayList> eventProcessors = new ArrayList>();
/**
* @see org.jwall.audit.EventProcessorPipeline#process(org.jwall.audit.Event)
*/
@Override
public void process(LogMessage event) throws EventProcessorException {
Map context = new HashMap();
for( EventProcessor proc : eventProcessors ){
try {
proc.processEvent( event, context );
} catch (Exception e) {
e.printStackTrace();
}
}
}
/**
* @see org.jwall.audit.EventProcessorPipeline#register(java.lang.Double, org.jwall.audit.EventProcessor)
*/
@Override
public void register(Double priority, EventProcessor proc) {
synchronized( eventProcessors ){
order.set( proc, priority );
eventProcessors.add( proc );
Collections.sort( eventProcessors, order );
}
}
/**
* @see org.jwall.audit.EventProcessorPipeline#unregister(org.jwall.audit.EventProcessor)
*/
@Override
public void unregister(EventProcessor proc) {
synchronized( eventProcessors ){
order.unset( proc );
eventProcessors.remove( proc );
Collections.sort( eventProcessors, order );
}
}
protected final class Order implements Comparator {
Map prios;
public Order(){
prios = new HashMap();
}
public Order( Map prios ){
this.prios = prios;
}
public void set( T key, Double prio ){
prios.put( key, prio );
}
public void unset( T key ){
prios.remove( key );
}
@Override
public int compare(T arg0, T arg1) {
if(arg0 == arg1 || arg0.equals( arg1 ) )
return 0;
Double p1 = prios.get( arg0 );
Double p2 = prios.get( arg1 );
if( p1 == null && p2 == null )
return -1;
if( p1 == null )
return -1;
if( p2 == null )
return 1;
int rc = p1.compareTo( p2 );
if( rc == 0 )
return arg0.toString().compareTo( arg1.toString() );
return rc;
}
}
@Override
public Double getPriority(EventProcessor proc) {
return Double.NaN;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy