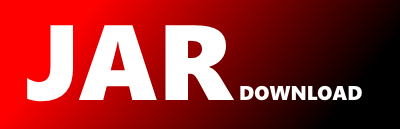
org.jwall.web.audit.AuditEventQueue Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit;
import java.io.IOException;
import java.util.Collection;
import java.util.Iterator;
import java.util.Queue;
import java.util.concurrent.LinkedBlockingQueue;
import org.jwall.web.audit.filter.AuditEventFilter;
import org.jwall.web.audit.io.AuditEventIterator;
import org.jwall.web.audit.io.AuditEventReader;
import org.jwall.web.audit.io.AuditEventSource;
import org.jwall.web.audit.io.ParseException;
/**
*
* A simple queue which holds some audit-events. The events are lined up
* in first-come-first-serve order. Accessing events may result in blocking
* if the queue is empty. See the methods comment for details.
*
* @author Christian Bockermann <[email protected]>
*
*/
public class AuditEventQueue
implements AuditEventSource, AuditEventReader, AuditEventListener
{
/** */
AuditEventFilter filter = null;
/** The internal structure which holds the events */
Queue events = new LinkedBlockingQueue();
/** A counter of all event that have been read from the queue */
protected long polled = 0;
/**
*
* This creates new empty queue for holding audit events.
*
*/
public AuditEventQueue(){
events = new LinkedBlockingQueue();
}
/**
* This creates a new queue which is initially filled with the
* given set of events.
*
* @param evts The initial set of events.
*/
public AuditEventQueue( Collection evts ){
events = new LinkedBlockingQueue();
events.addAll( evts );
}
/**
*
* This method returns true
, iff the queue is
* non-empty. A return-value of true
does not
* guarantee that the next call to nextEvent
* will not block, as a different thread might have emptied
* the list in the meantime.
*
* @return true
, iff queue is not empty.
*
*/
public boolean hasNext() {
return !events.isEmpty();
}
/**
*
* This method will return the head of the queue, i.e. the
* events that has been in the queue for the longest term.
* Calling this method will block in case of an empty queue.
*
* @return The first (head) audit-event of the queue.
*
*/
public AuditEvent nextEvent() {
AuditEvent e = events.poll();
if( e != null )
polled++;
return e;
}
/**
*
* This method sets a filter for the queue instance. The filter
* will be matched against every event arriving at the queue,
* thus a filtered queue will only contain events that match the
* filter.
*
* @param filter The filter used to match arriving events.
*/
public void setFilter(AuditEventFilter filter) {
this.filter = filter;
}
/**
* This method will add the event evt
to the queue. If
* a filter has been set beforehand, the event will only be added
* if it matches the filter.
*
* @see org.jwall.web.audit.AuditEventListener#eventArrived(org.jwall.web.audit.AuditEvent)
*/
public void eventArrived(AuditEvent evt) {
if( evt != null && (filter == null || filter.matches( evt ) ) )
events.add( evt );
}
/**
* This returns the number of events currently in the queue. Please
* not that the real number might differ if other threads concurrently
* added or removed events from the queue.
*
* @return The number of events in the queue.
*/
public int size(){
return events.size();
}
/**
* @see org.jwall.web.audit.io.AuditEventReader#bytesAvailable()
*/
public long bytesAvailable()
{
return 0;
}
/**
* @see org.jwall.web.audit.io.AuditEventReader#bytesRead()
*/
public long bytesRead()
{
return 0;
}
/**
* @see org.jwall.web.audit.io.AuditEventReader#close()
*/
public void close() throws IOException
{
}
public void eventsArrived( Collection events ){
for( AuditEvent evt : events )
eventArrived( evt );
}
/**
* @see org.jwall.web.audit.io.AuditEventReader#readNext()
*/
public AuditEvent readNext() throws IOException, ParseException
{
return this.nextEvent();
}
public boolean atEOF(){
return false;
}
@Override
public Iterator iterator()
{
try {
return new AuditEventIterator( this );
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy